Conditional Statements in JAVA
Submitted by moazkhan on Thursday, February 12, 2015 - 07:28.
Conditional Statements in JAVA
In this tutorial you will learn: 1. JAVA coding essentials 2. Taking input in java console 3. JAVA code for making a calculator 4. How the basic arithmetic operations are performed in JAVA Making Java Project Basic Java console program: • Open Eclipse > Workspace • File > new > java project • File > new > class Basic Coding Strategy for making a Calculator In this tutorial I will teach you guys how to make a calculator, the calculator here can perform the operations of addition, subtraction, multiplication and division. A good coder always makes a concrete plan and strategy before starting to write his code. The structure will be as, first we will take input of two numbers by the user, take in the operation as input and will perform the operation and display the result back to the user. Here for each of the operation we will use a separate portion of the program. Java programming is totally object oriented based, and everything here is done in classes, so here we have first declared the main(which is also inside the class) of the program here in which we are first taking the inputs by the user, as you can clearly see that for the first number ,by using the scanner class object we get the number and store it in the variable ‘num1’, then same procedure is followed for the second integer which is ‘num2’ and also for the symbol declared as ‘choice’. Here the data types must be carefully determined.- import java.util.Scanner;
- public class Calculator
- {
- int num1,num2;
- char choice;
- }
- num1 = inputScanner.nextInt();
- choice = inputScanner.next().charAt(0);//1 for addition
- num2 = inputScanner.nextInt();
Note: The name of your public class should be the same as your class name.
In this tutorial I have taught you guys to make a calculator by the simplest way possible which is using if/else statement, same result can be achieved by using switch statements. We can also use functions for the purpose , keep following they will be taught in upcoming tutorials.
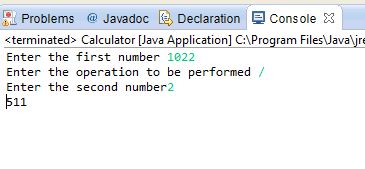