Drag/Drop and Copy Control in a TreeView Using Visual Basic 2008
Submitted by janobe on Sunday, April 27, 2014 - 09:47.
In this tutorial, I will show you how to drag/drop and copy in a Treeview by using Visual Basic 2008. I made this to show you not only a PictureBox or a TextBox can be drag and drop but also a treeview.
Let’s begin:
Open Visual Basic 2008, create a new Windows Application and drag the two Treeview in the Form. Name it “trview_left” and “trview_right”.
After that, click the two Treeviews and go to the properties. In the properties, click the events that look like a lightning symbol and double click the
Then, click the method name and choose the
Go back to the method name again and choose the
You can download the complete source code.
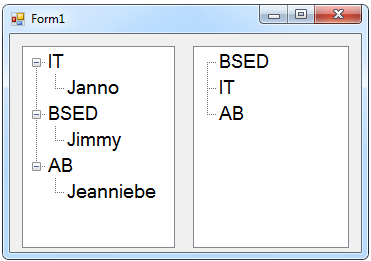
Drag/Drop
event handler. And do this following code.
Declare this constant variable above the sub procedure.
- 'DECLARE A CONSTANT VARIABLE
- 'IT IS USED FOR DETECTING THE CTRL KEY WHETHER THE CTRL KEY WAS PRESSED OR NOT DURING THE DRAG OPERATION
- Const mask_ctrl As Int32 = 8
- Private Sub TreeView_DragDrop(ByVal sender As System.Object, ByVal e As System.Windows.Forms.DragEventArgs) Handles trview_left.DragDrop, trview_right.DragDrop
- 'SET A VARIABLE TO HOLDS THE NODE TO BE DRAGGED
- Dim nodeorigination As TreeNode = CType(e.Data.GetData("System.Windows.Forms.TreeNode"), TreeNode)
- 'GETDATAPRESENT IS A BIT DIFFERENT FROM A TREEVIEW AND PICTUREBOX CONTROL.
- 'THE TREENODE IS NOT INCLUDED IN THE DATAFORMATS CLASS. THAT'S WHY IT'S NOT A PREDEFINED TYPE
- 'ONE OF THE GETDATAPRESENT CONTAINS A STRING THAT SPECIFY THE TYPE.
- If e.Data.GetDataPresent("System.Windows.Forms.TreeNode", False) Then
- Dim pnt As Point
- Dim distination_node As TreeNode
- 'POINTTOCLIENT IS USED TO COMPUTE THE LOCATION OF THE MOUSE OVER THE DESTINATION TREEVIEW
- pnt = CType(sender, TreeView).PointToClient(New Point(e.X, e.Y))
- 'POINT IS USED TO GET THE CLOSEST NODE IN THE DESTINATION TREEVIEW
- distination_node = CType(sender, TreeView).GetNodeAt(pnt)
- 'IF THE NEW NODE WAS NOT DROPPED DIRECTLY AT THE TOP OF THE NODE,
- 'THEN THE RESULT OF THE DESTINATIONNODE WILL BE NOTHING
- If distination_node IsNot Nothing Then
- 'IF THE ORIGINAL NODE AND DISTINATION NODE ARE THE SAME, THE THE NODE WOULD DISAPPEAR.
- 'THIS CODE INSURES THAT IT WILL NOT HAPPEN.
- If Not distination_node.TreeView Is nodeorigination.TreeView Then
- distination_node.Nodes.Add(CType(nodeorigination.Clone, TreeNode))
- 'EMPHASIZE PARENT NOT WHEN ADDING THE NEW NODE, WITHOUT THIS , ONLY THE a+ SYMBOL WILL APPEAR.
- distination_node.Expand()
- If (e.KeyState And maskctrl) <> maskctrl Then 'CHECKING THE CTRL KEY WAS NOT PRESSED
- nodeorigination.Remove() 'REMOVE THE NODE
- End If
- End If
- End If
- End If
- End Sub
Drag/Enter
events handler, and do this following code.
- Private Sub TreeView_DragEnter(ByVal sender As System.Object, ByVal e As System.Windows.Forms.DragEventArgs) Handles trview_left.DragEnter, trview_right.DragEnter
- If (e.Data.GetDataPresent("System.Windows.Forms.TreeNode")) Then 'CHECKING THE DRAG CONTENT TO BE SURE THAT IT IS THE CORRECT TYPE.
- If (e.KeyState And maskctrl) = maskctrl Then 'CHECKINKG IF THE CTRL KEY WAS PRESSED.
- 'IF IT WAS PRESSED
- e.Effect = DragDropEffects.Copy 'PERFORM COPY
- Else
- 'IF IT WAS NOT PRESSED
- e.Effect = DragDropEffects.Move 'PERFORM A MOVE
- End If
- Else
- 'REJECT THE DROP
- e.Effect = DragDropEffects.None
- End If
- End Sub
Item/Drag
event handler and do this following code.
- Private Sub TreeView_ItemDrag(ByVal sender As System.Object, ByVal e As System.Windows.Forms.ItemDragEventArgs) Handles trview_left.ItemDrag, trview_right.ItemDrag
- If e.Button = Windows.Forms.MouseButtons.Left Then
- 'PERFORM THE DRAG AND DROP OPERATION.
- DoDragDrop(e.Item, DragDropEffects.Move Or DragDropEffects.Copy)
- End If
- End Sub
Add new comment
- 270 views