In this article, we will create a program that can generate a random password when we pressed the button.
Now, let's start this tutorial!
1. Let's start with creating a Windows Form Application for this tutorial by following the following steps in Microsoft Visual Studio: Go to
File, click
New Project, and choose
Windows Application.
2. Next, add only one Button named
Button1 and labeled it as "
Generate Password". Add also TextBox named
TextBox1 for displaying the generated password. You must design your interface like this:
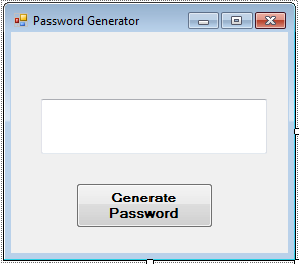
3. Declare these Global Variables:
Dim pNum As New Random(100)
Dim pLowerCase As New Random(500)
Dim pUpperCase As New Random(50)
Dim password As String
Dim RandomSelect As New Random(50)
Variable pNum is used for number/numeric content of the password.
Variable pLowerCase is used for LowerCase character content of the password.
Variable pUpperCaseis used for UpperCase character content of the password.
Variable passwordis used for resetting the new password.
Variable RandomSelecis used for random selection of the password.
4. Create a Function named
getPassword as String with parameters of
passLength as Integer and
Reset as Boolean.
Public Function getPassword(ByVal passLength As Integer, Optional ByVal Reset As Boolean = False) As String
Dim i As Integer
Dim ctr(2) As Integer
Dim charSelect(2) As String
Dim iSel As Integer
clear old passwords:
If Reset = True Then
password = ""
End If
create random numbers that will represent each upercase,lowercase,numbers:
For i = 1 To passLength
ctr(0) = pNum.Next(48, 57) 'Numbers 1 to 9
ctr(1) = pLowerCase.Next(65, 90) ' Lowercase Characters
ctr(2) = pUpperCase.Next(97, 122) ' Uppercase Characters
'put characters in strings
charSelect(0) = System.Convert.ToChar(ctr(0)).ToString
charSelect(1) = System.Convert.ToChar(ctr(1)).ToString
charSelect(2) = System.Convert.ToChar(ctr(2)).ToString
pick one of the three above for a character At Random: iSel = RandomSelect.Next(0, 3)
'colect all characters generated through the loop
password &= charSelect(iSel)
reset with new password:
If Reset = True Then
password
.Replace(password, charSelect
(iSel
)) End If
Next
Return password
End Function
5. Insert this code in your Button1_Click so that the function two generate password will be used in the textbox.
Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click
TextBox1.Text = getPassword(8, True)
End Sub
We used the getPassword function to display the 8 digit random characters in the textbox and when we press again the button it will reset the password and generate again as we declare that the Reset variable is set to True.
Output:
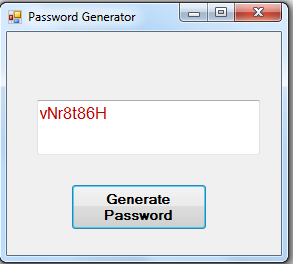
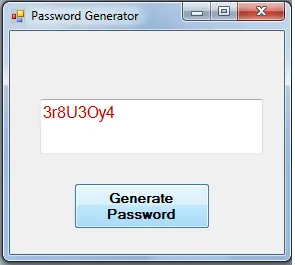
Download the source code below and try it! :)
For more inquiries just contact my number or e-mail below.
Best Regards,
Engr. Lyndon R. Bermoy
IT Instructor/System Developer/Android Developer
Mobile: 09079373999
Telephone: 826-9296
E-mail:[email protected]
Visit and like my page on Facebook at: Bermz ISware Solutions
Subscribe at my YouTube Channel at: SerBermz