Python - How To Apply Bootstrap On Django
Submitted by razormist on Monday, July 31, 2017 - 17:31.
In this tutorial we will try to Apply Bootstrap To Django Framework to make a stunning website. Django is a free and open source web application framework, written in Python. The official project site describes Django as "a high-level Python Web framework that encourages rapid development and clean, pragmatic design. And It’s free and open source to every developer in the world."
Getting started
First you will have to download & install the Python IDLE's, here's the link for the Integrated Development And Learning Environment for Python https://www.python.org/downloads/.
Then download the bootstrap front-end framework, here's the link for the Bootstrap http://getbootstrap.com/
After Python IDLE's is installed, open the command prompt then type "pip install Django", and hit enter.
Wait for the django to be downloaded and installed at the same time. Then After that type "python -m django version" to check if django is installed and what version of django is.
Creating the App
After setting django we will now create the web app for the web server. First create a new folder named "Python - How To Apply Bootstrap On Django", then cd to a newly created folder, then type "django-admin startproject webserver". A new folder will be created on the directory named 'webserver'.
Running The Server
After creating a project, cd to the newly created directory, then type "manage.py runserver" to start the server running. The "manage.py" is a command of django-admin that utilize the administrative tasks of python web framework.
Here is the image of python web server:
Note: Type '127.0.0.1:8000' in the url browser to view the server. When there is code changes in the server just (ctrl + C) to command prompt to stop the server from running, then start again to avoid errors.
Creating The Website
This time will now create the web app to display the web models. First locate the directory of the app via command prompt cd, then type "manage.py startapp bootstrap". A new directory will be create inside the app named "bootstrap".
Setting up The URL
This time will now create a url address to connect the app from the server. First Go to webserver directory, then open urls via Python IDLE's or any text editor. Then import "include" module beside the url module. After that copy/paste the code below inside the urlpatterns.
It will be look like this.
Creating The Path For The Pages
Now that we set the connect we will now create a path for the web pages. All you have to do first is to go to bootstrap directory, then copy/paste the code below and save it inside bootstrap directory namely 'urls.py' The file name must be urls.py or else there will be an error in the code.
Creating The Views
The views contains the interface of the website. This is where you assign the html code for rendering it to django framework. To do that first open the views.py, the copy/paste the code below.
Importing The Bootstrap
We will now import the bootstrap framework. All you have to do is to go to bootstrap directory, then create a directory called 'static' and after that create a sub directory of it namely 'bootstrap'. After the directory is created import the css folder of bootstrap inside bootstrap directory sub directory of static.
Creating The Mark up Language
Now we will create the html interface for the django framework. First go to bootstrap directory, then create a directory called "templates" and create a sub directory on it called bootstrap.
base.html
Save it as "base.html" inside the bootstrap directory sub directory of templates.
index.html
Save it as "index.html" inside the bootstrap directory "sub directory of templates".
mission.html
Save it as "mission.html" inside the bootstrap directory "sub directory of templates".
vision.html
Save it as "vision.html" inside the bootstrap directory "sub directory of templates".
aboutus.html
Save it as "aboutus.html" inside the bootstrap directory "sub directory of templates".
Registering The App To The Server
Now that we created the inteface we will now then register the app to the server. To do that go to the webserver directory, then open "settings.py" via Python IDLE's or any text editor. Then copy/paste this script inside the INSTALLED_APP variables 'bootstrap.apps.BootstrapConfig'.
It will be like this:
Migrating The App To The Server
Now that we done in setting up all the necessary needed, we will now then migrate the app to the server. To do that open the command prompt then go to the "webserver" directory, then type "manage.py migrate".
Now try to run the server again, and see if all things are done.
There you have it we successfully Apply Bootstrap For Django A Web Framework Of Python. I hope that this simple tutorial help you for what you are looking for. For more updates and tutorials just kindly visit this site. Enjoy Coding!!!
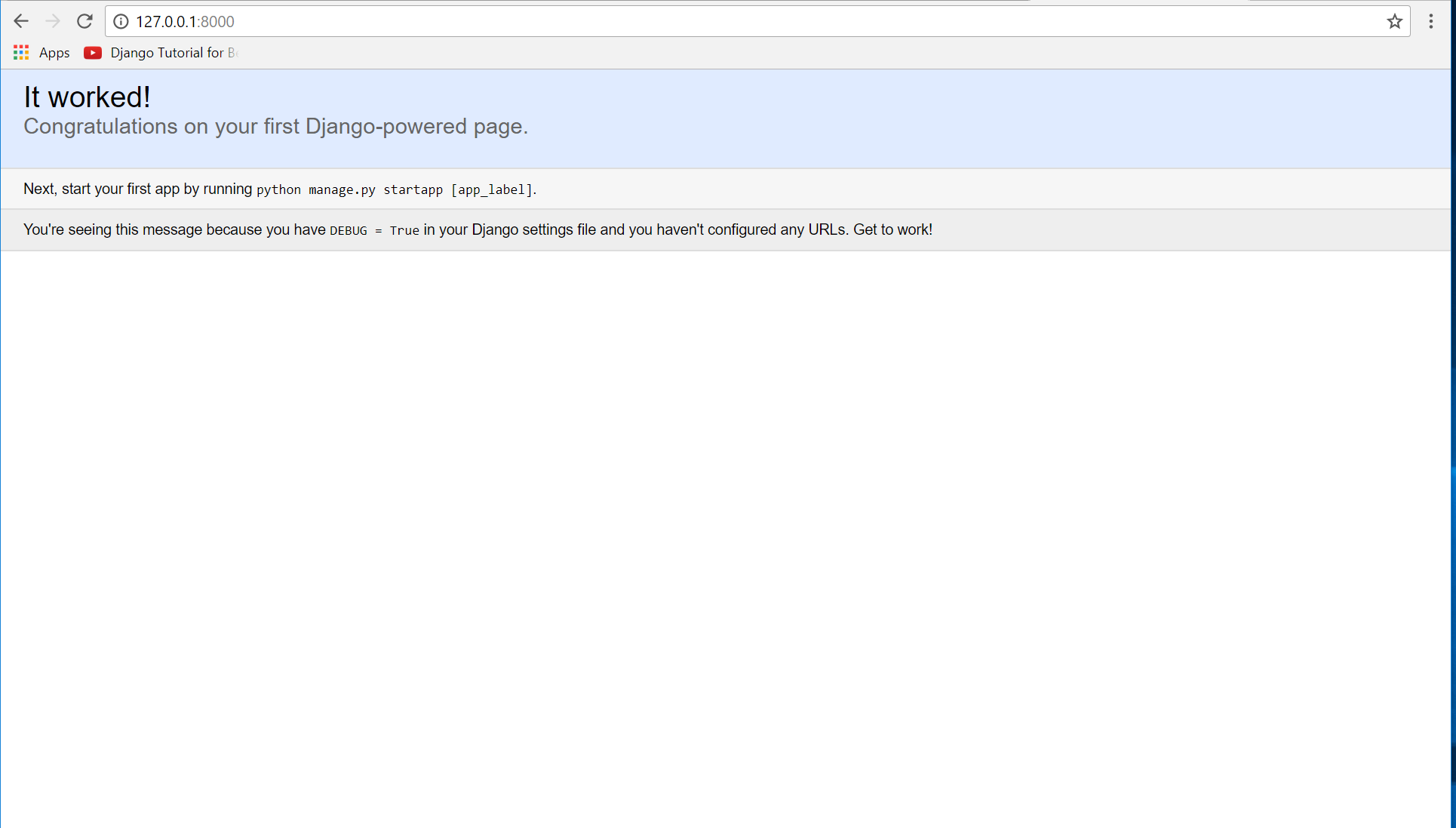
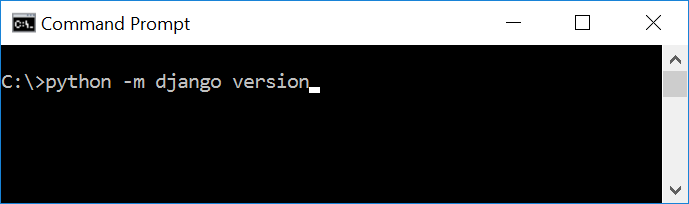

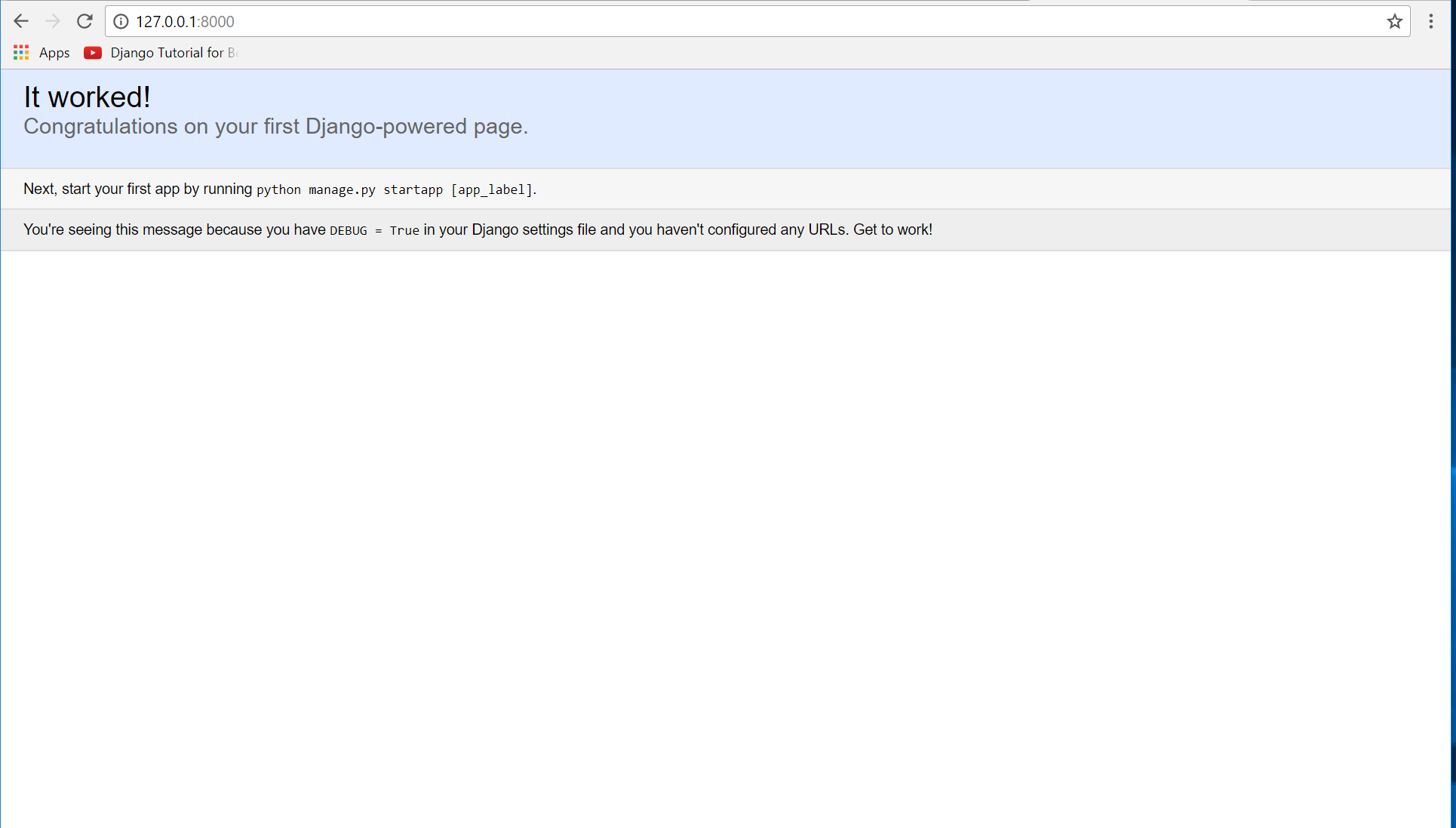

- url(r'^bootstrap/', include('bootstrap.urls')),
- from django.conf.urls import include, url
- from django.contrib import admin
- urlpatterns = [
- url(r'^admin/', admin.site.urls),
- url(r'^bootstrap/', include('bootstrap.urls')),
- ]
- from django.conf.urls import url
- from . import views
- app_name = "bootstrap"
- urlpatterns = [
- url(r'^$', views.index, name = "index"),
- url(r'^mission$', views.mission, name = "mission"),
- url(r'^vision$', views.vision, name = "vision"),
- url(r'^aboutus$', views.aboutus, name = "aboutus"),
- ]
- from django.shortcuts import render
- # Create your views here.
- def index(request):
- return render(request, 'bootstrap/index.html')
- def mission(request):
- return render(request, 'bootstrap/mission.html')
- def vision(request):
- return render(request, 'bootstrap/vision.html')
- def aboutus(request):
- return render(request, 'bootstrap/aboutus.html')
- <!DOCTYPE html>
- <html lang = "eng">
- <head>
- <meta charset = "UTF-8" name = "viewport" content = "device=width-device, initial-scale=1" />
- {% load staticfiles %}
- <link rel = "stylesheet" type = "text/css" href = "{% static 'bootstrap/css/bootstrap.css' %}"/>
- </head>
- <body>
- <nav class = "navbar navbar-inverse">
- <div class = "container-fluid">
- </div>
- </nav>
- {% block body %}
- {% endblock %}
- </body>
- </html>
- INSTALLED_APPS = [
- 'bootstrap.apps.BootstrapConfig',
- 'django.contrib.admin',
- 'django.contrib.auth',
- 'django.contrib.contenttypes',
- 'django.contrib.sessions',
- 'django.contrib.messages',
- 'django.contrib.staticfiles',
- ]
