PHP - Simple Categorizing Result Using MySQLi
Submitted by razormist on Thursday, June 6, 2019 - 11:11.
In this tutorial we will create a Simple Categorizing Result using MySQLi. This code will categorize the data that display in the table base on the user selected category. The code use MySQLi SELECT query to categorize the data in the table by providing a POST value in the WHERE clause. This a user-friendly program feel free to modify and use it to your system.
We will be using PHP as a scripting language that interepret in the webserver such as xamp, wamp, etc. It is widely use by modern website application to handle and protect user confidential information.
There you have it we successfully created Simple Categorizing Result using MySQLi. I hope that this simple tutorial help you to what you are looking for. For more updates and tutorials just kindly visit this site. Enjoy Coding!
Getting Started:
First you have to download & install XAMPP or any local server that run PHP scripts. Here's the link for XAMPP server https://www.apachefriends.org/index.html. And, this is the link for the bootstrap that i used for the layout design https://getbootstrap.com/. Lastly, this is the link for the jquery that i usedhttps://jquery.com/.Creating Database
Open your database web server then create a database name in it db_result, after that click Import then locate the database file inside the folder of the application then click ok.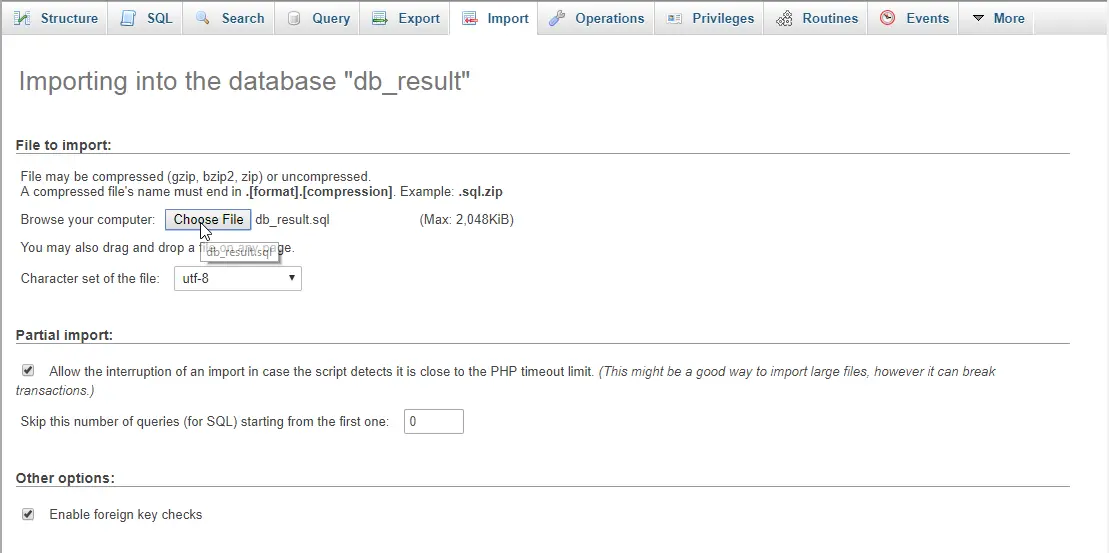
Creating the database connection
Open your any kind of text editor(notepad++, etc..). Then just copy/paste the code below then name it conn.php.- <?php
- if(!$conn){
- }
- ?>
Creating The Interface
This is where we will create a simple form for our application. To create the forms simply copy and write it into your text editor, then save it as index.php.- <!DOCTYPE html>
- <html lang="en">
- <head>
- <meta charse="UTF-8" name="viewport" content="width=device-width, initial-scale=1" />
- <link rel="stylesheet" type="text/css" href="css/bootstrap.css" />
- </head>
- <body>
- <nav class="navbar navbar-default">
- <div class="container-fluid">
- <a class="navbar-brand" href="https://sourcecodester.com">Sourcecodester</a>
- </div>
- </nav>
- <div class="col-md-3"></div>
- <div class="col-md-6 well">
- <h3 class="text-primary">PHP - Simple Categoring Result Using MySQLi</h3>
- <hr style="border-top:1px dotted #ccc;"/>
- <button class="btn btn-success" data-toggle="modal" data-target="#form_modal"><span class="glyphicon glyphicon-plus"></span> Add student</button>
- <br /><br />
- <form class="form-inline" method="POST" action="">
- <label>Select Category:</label>
- <select class="form-control" name="category">
- <option value="All">All</option>
- <option value="Math">Math</option>
- <option value="Science">Science</option>
- <option value="English">English</option>
- <option value="MAPEH">MAPEH</option>
- </select>
- <button class="btn btn-warning" name="change">Change</button>
- </form>
- <br />
- <h4>Display by: <span style="font-weight:bold;"><?php if(ISSET($_POST['change'])){echo $_POST['category'];}else{echo "All";}?></span></h4>
- <table class="table table-bordered">
- <thead class="alert-info">
- <tr>
- <th>Category</th>
- <th>Student Name</th>
- </tr>
- </thead>
- <tbody>
- <?php include'display.php'?>
- </tbody>
- </table>
- </div>
- <div class="modal fade" id="form_modal" aria-hidden="true">
- <div class="modal-dialog">
- <div class="modal-content">
- <form method="POST" action="save_student.php">
- <div class="modal-header">
- <h3 class="modal-title">Add Student</h3>
- </div>
- <div class="modal-body">
- <div class="col-md-2"></div>
- <div class="col-md-8">
- <div class="form-group">
- <label>Subject</label>
- <select name="subject" class="form-control" required="required">
- <option value="">Select an option</option>
- <option value="Math">Math</option>
- <option value="Science">Science</option>
- <option value="English">English</option>
- <option value="MAPEH">MAPEH</option>
- </select>
- </div>
- <div class="form-group">
- <label>Student Name</label>
- <input type="text" class="form-control" name="stud_name" required="required"/>
- </div>
- </div>
- </div>
- <br style="clear:both;"/>
- <div class="modal-footer">
- <button type="button" class="btn btn-danger" data-dismiss="modal"><span class="glyphicon glyphicon-remove"></span> Close</button>
- <button class="btn btn-primary" name="save"><span class="glyphicon glyphicon-save"></span> Save</button>
- </div>
- </form>
- </div>
- </div>
- </div>
- <script src="js/jquery-3.2.1.min.js"></script>
- <script src="js/bootstrap.js"></script>
- </body>
- </html>
Creating the PHP Query
This code contains the php query of the application. This code will store the user data inputs to the database server. To make this just copy and write these block of codes below inside the text editor, then save it as save_student.php.- <?php
- require_once'conn.php';
- $subject=$_POST['subject'];
- $stud_name=$_POST['stud_name'];
- }
- mysqli_query($conn, "INSERT INTO `student` VALUES('', '$subject', '$stud_name')") or die(mysqli_error());
- ?>
Creating the Main Function
This code contains the main function of the application. This code will categorize the data in the table when the button is clicked. To make this just copy and write these block of codes below inside the text editor, then save it as display.php- <?php
- require'conn.php';
- $category=$_POST['category'];
- switch($category){
- case"Math":
- $query=mysqli_query($conn, "SELECT * FROM `student` WHERE `subject`='$category'") or die(mysqli_error());
- echo"<tr><td>".$fetch['subject']."</td><td>".$fetch['stud_name']."</td></tr>";
- }
- break;
- case"Science":
- $query=mysqli_query($conn, "SELECT * FROM `student` WHERE `subject`='$category'") or die(mysqli_error());
- echo"<tr><td>".$fetch['subject']."</td><td>".$fetch['stud_name']."</td></tr>";
- }
- break;
- case"English":
- $query=mysqli_query($conn, "SELECT * FROM `student` WHERE `subject`='$category'") or die(mysqli_error());
- echo"<tr><td>".$fetch['subject']."</td><td>".$fetch['stud_name']."</td></tr>";
- }
- break;
- case"MAPEH":
- $query=mysqli_query($conn, "SELECT * FROM `student` WHERE `subject`='$category'") or die(mysqli_error());
- echo"<tr><td>".$fetch['subject']."</td><td>".$fetch['stud_name']."</td></tr>";
- }
- break;
- case"All":
- echo"<tr><td>".$fetch['subject']."</td><td>".$fetch['stud_name']."</td></tr>";
- }
- break;
- }
- }else{
- echo"<tr><td>".$fetch['subject']."</td><td>".$fetch['stud_name']."</td></tr>";
- }
- }
- ?>
Add new comment
- 296 views