PHP - Simple Restore Deleted Data
Submitted by razormist on Friday, May 31, 2019 - 10:44.
In this tutorial we will create a Simple Restore Deleted Data using PHP. This code can restore a deleted data when the user click the button. The code use mysqli_query() to fetch all data in the database, therefore it can retrieve back the delete primary key by alternating the INSERT and DELETE query. This a user-friendly program feel free to modify and use it to your system.
We will be using PHP as a scripting language that interpret in the webserver such as xamp, wamp, etc. It is widely use by modern website application to handle and protect user confidential information.
trash.php
restore.php
There you have it we successfully created Simple Restore Deleted Data using PHP. I hope that this simple tutorial help you to what you are looking for. For more updates and tutorials just kindly visit this site. Enjoy Coding!
Getting Started:
First you have to download & install XAMPP or any local server that run PHP scripts. Here's the link for XAMPP server https://www.apachefriends.org/index.html. And, this is the link for the bootstrap that i used for the layout design https://getbootstrap.com/.Creating Database
Open your database web server then create a database name in it db_restore, after that click Import then locate the database file inside the folder of the application then click ok.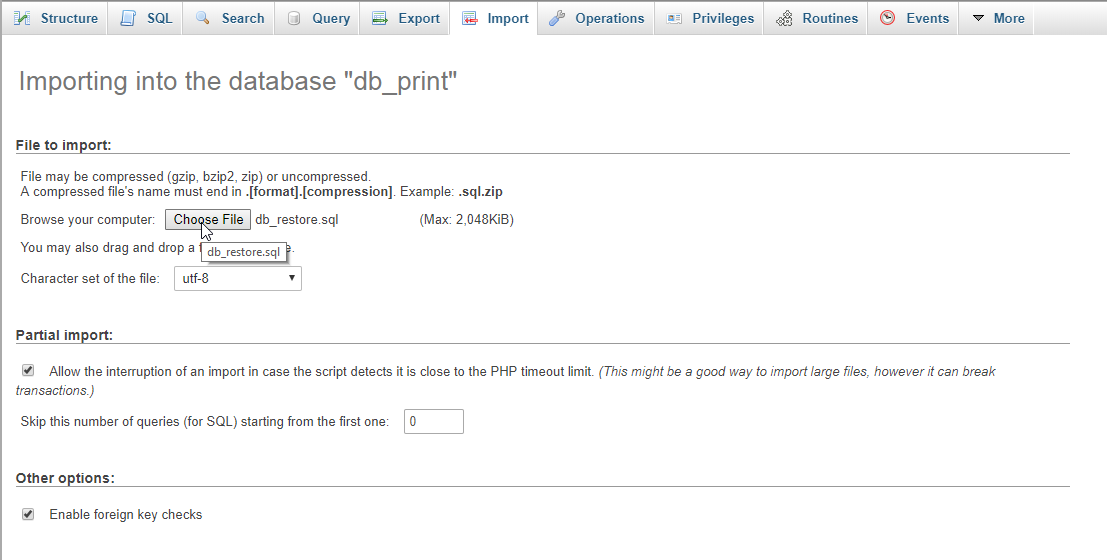
Creating the database connection
Open your any kind of text editor(notepad++, etc..). Then just copy/paste the code below then name it conn.php.- <?php
- if(!$conn){
- }
- ?>
Creating The Interface
This is where we will create a simple form for our application. To create the forms simply copy and write it into your text editor, then save it as shown below index.php- <!DOCTYPE html>
- <html lang="en">
- <head>
- <meta charset="UTF-8" name="viewport" content="width=device-width, initial-scale=1"/>
- <link rel="stylesheet" type="text/css" href="css/bootstrap.css"/>
- </head>
- <body>
- <nav class="navbar navbar-default">
- <div class="container-fluid">
- <a class="navbar-brand" href="https://sourcecodester.com">Sourcecodester</a>
- </div>
- </nav>
- <div class="col-md-3"></div>
- <div class="col-md-6 well">
- <h3 class="text-primary">PHP - Simple Restore Deleted Data</h3>
- <hr style="border-top:1px dotted #ccc;"/>
- <button type="button" class="btn btn-success" data-toggle="modal" data-target="#form_modal"><span class="glyphicon glyphicon-plus"></span> Add member</button>
- <a href="trash.php" class="pull-right"><span class="glyphicon glyphicon-trash"></span> Trash Bin</a>
- <br /><br />
- <table class="table table-bordered">
- <thead class="alert-info">
- <tr>
- <th>Firstname</th>
- <th>Lastname</th>
- <th>Age</th>
- <th>Address</th>
- <th>Action</th>
- </tr>
- </thead>
- <tbody style="background-color:#fff;">
- <?php
- require 'conn.php';
- ?>
- <tr>
- <td><?php echo $fetch['firstname']?></td>
- <td><?php echo $fetch['lastname']?></td>
- <td><?php echo $fetch['age']?></td>
- <td><?php echo $fetch['address']?></td>
- <td><a class="btn btn-danger" href="remove.php?mem_id=<?php echo $fetch['mem_id']?>"><span class="glyphicon glyphicon-remove"></span> Remove</a></td>
- </tr>
- <?php
- }
- ?>
- </tbody>
- </table>
- </div>
- <div class="modal fade" id="form_modal" aria-hidden="true">
- <div class="modal-dialog">
- <div class="modal-content">
- <form method="POST" action="save_member.php">
- <div class="modal-header">
- <h3 class="modal-title">Add Member</h3>
- </div>
- <div class="modal-body">
- <div class="col-md-2"></div>
- <div class="col-md-8">
- <div class="form-group">
- <label>Firstname</label>
- <input type="text" name="firstname" class="form-control" required="required"/>
- </div>
- <div class="form-group">
- <label>Lastname</label>
- <input type="text" name="lastname" class="form-control" required="required"/>
- </div>
- <div class="form-group">
- <label>Age</label>
- <input type="number" min="1" name="age" class="form-control" required="required" />
- </div>
- <div class="form-group">
- <label>Address</label>
- <input type="text" name="address" class="form-control" required="required" />
- </div>
- </div>
- </div>
- <div style="clear:both;"></div>
- <div class="modal-footer">
- <button name="save" class="btn btn-primary"><span class="glyphicon glyphicon-save"></span> Save</button>
- <button class="btn btn-danger" type="button" data-dismiss="modal"><span class="glyphicon glyphicon-remove"></span> Close</button>
- </div>
- </div>
- </form>
- </div>
- </div>
- </div>
- <script src="js/jquery-3.2.1.min.js"></script>
- <script src="js/bootstrap.js"></script>
- </body>
- </html>
- <!DOCTYPE html>
- <html lang="en">
- <head>
- <meta charset="UTF-8" name="viewport" content="width=device-width, initial-scale=1"/>
- <link rel="stylesheet" type="text/css" href="css/bootstrap.css"/>
- </head>
- <body>
- <nav class="navbar navbar-default">
- <div class="container-fluid">
- <a class="navbar-brand" href="https://sourcecodester.com">Sourcecodester</a>
- </div>
- </nav>
- <div class="col-md-3"></div>
- <div class="col-md-6 well">
- <h3 class="text-primary">PHP - Simple Restore Deleted Data</h3>
- <hr style="border-top:1px dotted #ccc;"/>
- <a href="index.php" class="pull-right">Go to main page</a>
- <br /><br />
- <div class="alert alert-danger">TRASH BIN</div>
- <table class="table table-bordered">
- <thead class="alert-info">
- <tr>
- <th>Firstname</th>
- <th>Lastname</th>
- <th>Age</th>
- <th>Address</th>
- <th>Action</th>
- </tr>
- </thead>
- <tbody style="background-color:#fff;">
- <?php
- require 'conn.php';
- ?>
- <tr>
- <td><?php echo $fetch['firstname']?></td>
- <td><?php echo $fetch['lastname']?></td>
- <td><?php echo $fetch['age']?></td>
- <td><?php echo $fetch['address']?></td>
- <td><a class="btn btn-success" href="restore.php?trash_id=<?php echo $fetch['mem_id']?>"><span class="glyphicon glyphicon-reset"></span> Restore</a></td>
- </tr>
- <?php
- }
- ?>
- </tbody>
- </table>
- </div>
- </body>
- </html>
Creating the PHP Query
This code contains the php query of the application. This code will store the data inputs to the database server. To do that just copy and write this block of codes inside the text editor, then save it as save_member.php.- <?php
- require_once 'conn.php';
- $firstname = $_POST['firstname'];
- $lastname = $_POST['lastname'];
- $age = $_POST['age'];
- $address = $_POST['address'];
- mysqli_query($conn, "INSERT INTO `member` VALUES('', '$firstname', '$lastname', '$age', '$address')") or die(mysqli_error());
- }
- ?>
Creating Main Function
This code contains the main function of the application. This code can remove and restore the data when the button is clicked. To do that just copy and write this block of codes inside the text editor, then save it as shown below. remove.php- <?php
- require_once 'conn.php';
- $mem_id = $_REQUEST['mem_id'];
- $query=mysqli_query($conn, "SELECT * FROM `member` WHERE `mem_id` = '$mem_id'") or die(mysqli_error());
- mysqli_query($conn, "INSERT INTO `trash` VALUES('', '$fetch[mem_id]', '$fetch[firstname]', '$fetch[lastname]', '$fetch[age]', '$fetch[address]')") or die(mysqli_error());
- }
- ?>
- <?php
- require_once 'conn.php';
- $trash_id = $_REQUEST['trash_id'];
- $query=mysqli_query($conn, "SELECT * FROM `trash` WHERE `trash_id` = '$trash_id'") or die(mysqli_error());
- mysqli_query($conn, "INSERT INTO `member` VALUES('', '$fetch[firstname]', '$fetch[lastname]', '$fetch[age]', '$fetch[address]')") or die(mysqli_error());
- }
- ?>