PHP - Simple Update Table Row Using PDO
Submitted by razormist on Wednesday, May 29, 2019 - 09:08.
In this tutorial we will create a Simple Update Table Row using PDO. This code can update a data in the database server by the use of advance PDO query. The code use a PDO to update a specific data in the table row with user precaution for saving changes in the data.
We will be using PDO as a query scripting it an acronym for PHP Data Objects. It provides a data-access abstraction layer, which means that, regardless of which database you're using, you use the same functions to issue queries and fetch data. This means developers can write portable code much easier.
edit.php
There you have it we successfully created a Simple Update Table Row using PDO. I hope that this simple tutorial help you to what you are looking for. For more updates and tutorials just kindly visit this site. Enjoy Coding!
Getting Started:
First you have to download & install XAMPP or any local server that run PHP scripts. Here's the link for XAMPP server https://www.apachefriends.org/index.html. And, this is the link for the bootstrap that i used for the layout design https://getbootstrap.com/.Creating Database
Open your database web server then create a database name in it db_update_pdo, after that click Import then locate the database file inside the folder of the application then click ok.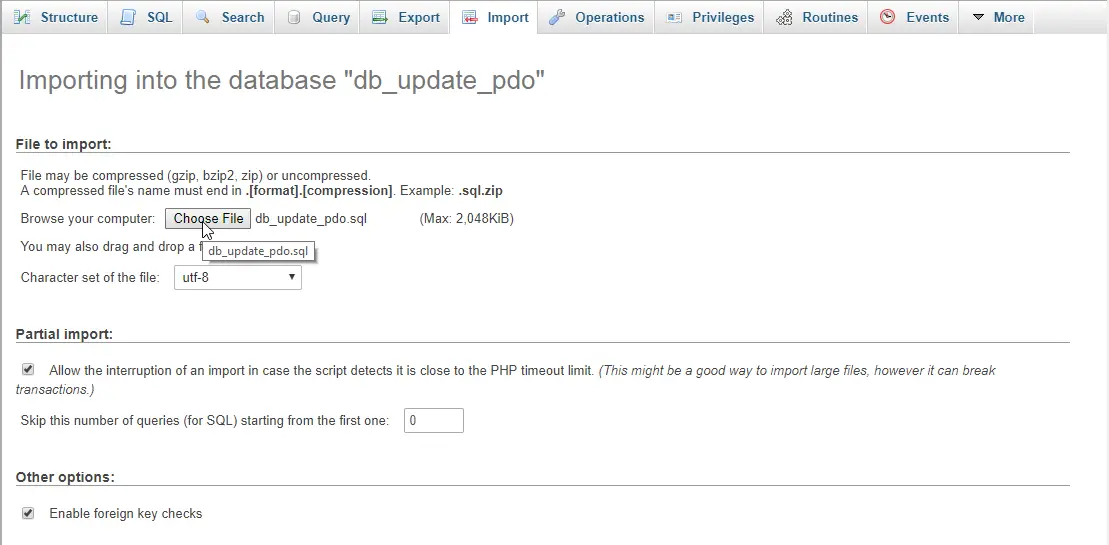
Creating the database connection
Open your any kind of text editor(notepad++, etc..). Then just copy/paste the code below then name it conn.php.- <?php
- $conn = new PDO( 'mysql:host=localhost;dbname=db_update_pdo', 'root', '');
- if(!$conn){
- }
- ?>
Creating The Interface
This is where we will create a simple form for our application. To create the forms simply copy and write it into your text editor, then save it as shown below. index.php- <!DOCTYPE html>
- <html lang="en">
- <head>
- <link rel="stylesheet" type="text/css" href="css/bootstrap.css">
- <meta charset="UTF-8" name="viewport" content="width=device-width, initial-scale=1"/>
- </head>
- <body>
- <nav class="navbar navbar-default">
- <div class="container-fluid">
- <a href="https://sourcecodester.com" class="navbar-brand">Sourcecodester</a>
- </div>
- </nav>
- <div class="col-md-3"></div>
- <div class="col-md-6 well">
- <h3 class="text-primary">PHP - Simple Update Table Row Using PDO</h3>
- <hr style="border-top:1px dotted #ccc;" />
- <table class="table table-bordered">
- <thead class="alert-info">
- <tr>
- <th>Firstname</th>
- <th>Lastname</th>
- <th>Username</th>
- <th>Password</th>
- <th>Action</th>
- </tr>
- </thead>
- <tbody>
- <?php
- require 'conn.php';
- $sql = $conn->prepare("SELECT * FROM `user` ORDER BY `lastname` ASC");
- $sql->execute();
- while($row = $sql->fetch()){
- ?>
- <tr>
- <td><?php echo $row['firstname']?></td>
- <td><?php echo $row['lastname']?></td>
- <td><?php echo $row['username']?></td>
- <td>********</td>
- <td><a class="btn btn-warning" href="edit.php?id=<?php echo $row['user_id']?>"><span class="glyphicon glyphicon-edit"></span> Edit</a></td>
- </tr>
- <?php
- }
- ?>
- </tbody>
- </table>
- </div>
- </body>
- </html>
- <!DOCTYPE html>
- <html lang="en">
- <head>
- <link rel="stylesheet" type="text/css" href="css/bootstrap.css">
- <meta charset="UTF-8" name="viewport" content="width=device-width, initial-scale=1"/>
- </head>
- <body>
- <nav class="navbar navbar-default">
- <div class="container-fluid">
- <a href="https://sourcecodester.com" class="navbar-brand">Sourcecodester</a>
- </div>
- </nav>
- <div class="col-md-3"></div>
- <div class="col-md-6 well">
- <h3 class="text-primary">PHP - Simple Update Table Row Using PDO</h3>
- <hr style="border-top:1px dotted #ccc;" />
- <div class="col-md-3"></div>
- <div class="col-md-6">
- <?php
- require_once 'conn.php';
- $id = $_GET['id'];
- $sql = $conn->prepare("SELECT * FROM `user` WHERE `user_id`='$id'");
- $sql->execute();
- $row = $sql->fetch();
- }
- ?>
- <form method="POST" action="update.php?id=<?php echo $id?>">
- <div class="form-group">
- <label>Firstname</label>
- <input class="form-control" type="text" value="<?php echo $row['firstname']?>" name="firstname"/>
- </div>
- <div class="form-group">
- <label>Lastname</label>
- <input class="form-control" type="text" value="<?php echo $row['lastname']?>" name="lastname"/>
- </div>
- <div class="form-group">
- <label>Username</label>
- <input class="form-control" type="text" value="<?php echo $row['username']?>" name="username"/>
- </div>
- <div class="form-group">
- <label>Password</label>
- <input class="form-control" type="password" value="<?php echo $row['password']?>" name="password"/>
- </div>
- <div class="form-group">
- <button class="btn btn-warning form-control" name="update">Save Changes</button>
- </div>
- </form>
- <?php
- $conn = null;
- ?>
- </div>
- </div>
- </body>
- </html>
Creating the Main Function
This code contains the main function of the application. This code will update a data when the button is clicked. To do this just kindly write these block of codes inside the text editor, then save it as update.php.- <?php
- require_once 'conn.php';
- try{
- $id = $_GET['id'];
- $firstname = $_POST['firstname'];
- $lastname = $_POST['lastname'];
- $username = $_POST['username'];
- $password = $_POST['password'];
- $conn->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);
- $sql = "UPDATE `user`SET `firstname` = '$firstname', `lastname` = '$lastname', `username` = '$username', `password` = '$password' WHERE `user_id` = '$id'";
- }catch(PDOException $e){
- echo $e->getMessage();
- }
- $conn = null;
- echo "<script>alert('Succesfully updated an account!')</script>";
- echo "<script>window.location='index.php'</script>";
- }
- ?>