Image Upload With Gallery in PHP Tutorial
In this tutorial we will create a Image Upload With Gallery using PHP. This code will automatically arrange the uploaded image into a gallery-style format. The code use MySQLi SELECT parameter to establish a gallery setup page by looping the images in the database server. This is a user-friendly program feel free to modify and use it in your system.
We will be using PHP as a scripting language that interprets in the web server such as XAMPP, WAMP, etc. It is widely used by modern website applications to handle and protect user confidential information.
Getting Started:
First you have to download & install XAMPP or any local server that run PHP scripts. Here's the link for XAMPP server https://www.apachefriends.org/index.html.
Lastly, this is the link for the bootstrap that i used for the layout design https://getbootstrap.com/.Creating Database
Open your database web server then create a database name in it db_gallery, after that click Import then locate the database file inside the folder of the application then click ok.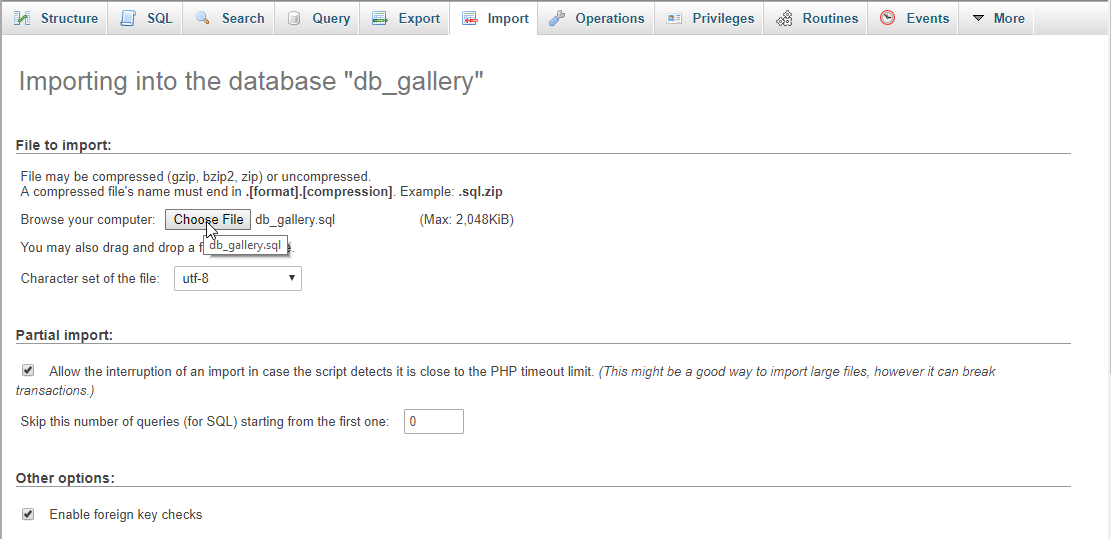
Or, you can simple copy/paste the SQL
script below to created the table and its column. To do this, navigate your Database to the SQL Tab on the PHPMyAdmin and paste the script in the provided text field and click the Go button.
Creating the database connection
Open your any kind of text editor(notepad++, etc..). Then just copy/paste the code below then name it conn.php.
- <?php
- if(!$conn){
- }
- ?>
Creating The Interface
This is where we will create a simple form for our application. To create the forms simply copy and write it into your text editor, then save it as index.php.
- <!DOCTYPE html>
- <html lang="en">
- <head>
- <meta charset="UTF-8" name="viewport" content="width=device-width, initial-scale=1"/>
- <link rel="stylesheet" type="text/css" href="css/bootstrap.css"/>
- </head>
- <body>
- <nav class="navbar navbar-default">
- <div class="container-fluid">
- <a class="navbar-brand" href="https://sourcecodester.com">Sourcecodester</a>
- </div>
- </nav>
- <div class="col-md-3"></div>
- <div class="col-md-6 well">
- <h3 class="text-primary">PHP - Image Upload With Gallery</h3>
- <hr style="border-top:1px dotted #ccc;"/>
- <form method="POST" action="upload.php" enctype="multipart/form-data">
- <div class="form-inline">
- <label>Upload here</label>
- <input type="file" name="image" class="form-control" required="required"/>
- <button class="btn btn-primary" name="upload"><span class="glyphicon glyphicon-upload"></span> Upload</button>
- </div>
- </form>
- <br />
- <div class="alert alert-info">My Gallery</div>
- <?php
- require 'conn.php';
- ?>
- <div style="border:1px solid #000; height:190px; width:190px; padding:4px; float:left; margin:10px;">
- <a href="<?php echo $fetch['location']?>"><img src="<?php echo $fetch['location']?>" width="180" height="180"/></a>
- </div>
- <?php
- }
- ?>
- </div>
- </body>
- </html>
Creating PHP Query
This code contains the PHP query of the application. This code will upload and display the images when the button is clicked. To do that just copy and write this block of codes inside the text editor, then save it as upload.php.
- <?php
- require_once 'conn.php';
- $image_name = $_FILES['image']['name'];
- $image_temp = $_FILES['image']['tmp_name'];
- $image_size = $_FILES['image']['size'];
- $path = "upload/".$name;
- if($image_size > 5242880){
- echo "<script>alert('File too large!')</script>";
- echo "<script>window.location = 'index.php'</script>";
- }else{
- echo "<script>alert('Image uploaded!')</script>";
- echo "<script>window.location = 'index.php'</script>";
- }
- }
- }else{
- echo "<script>alert('Invalid image format!')</script>";
- echo "<script>window.location = 'index.php'</script>";
- }
- }
- ?>
DEMO Video
There you have it we successfully created Image Upload With Gallery using PHP. I hope that this simple tutorial helps you to what you are looking for. For more updates and tutorials just kindly visit this site.
Enjoy Coding!