PHP - Retrieve MySQLi Data as JSON Format
Submitted by razormist on Wednesday, February 27, 2019 - 14:14.
In this tutorial we will create a Retrieve MySQLi Data as JSON Format. By using PHP, you can let your user directly interact with the script and easily to learned its syntax. It is mostly used by a newly coders for its user friendly environment. jQuery is a cross-platform JavaScript library designed to simplify the client-side scripting of HTML. So Let's do the coding...
There you have it we successfully created Retrieve MySQLi Data as JSON Format. I hope that this simple tutorial help you to what you are looking for. For more updates and tutorials just kindly visit this site. Enjoy Coding!!!
Getting started:
First you have to download & install XAMPP or any local server that run PHP scripts. Here's the link for XAMPP server https://www.apachefriends.org/index.html. And this is the link for the jquery that i used in this tutorial https://jquery.com/. Lastly, this is the link for the bootstrap that i used for the layout design https://getbootstrap.com/.Creating Database
Open your database web server then create a database name in it db_json, after that click Import then locate the database file inside the folder of the application then click ok.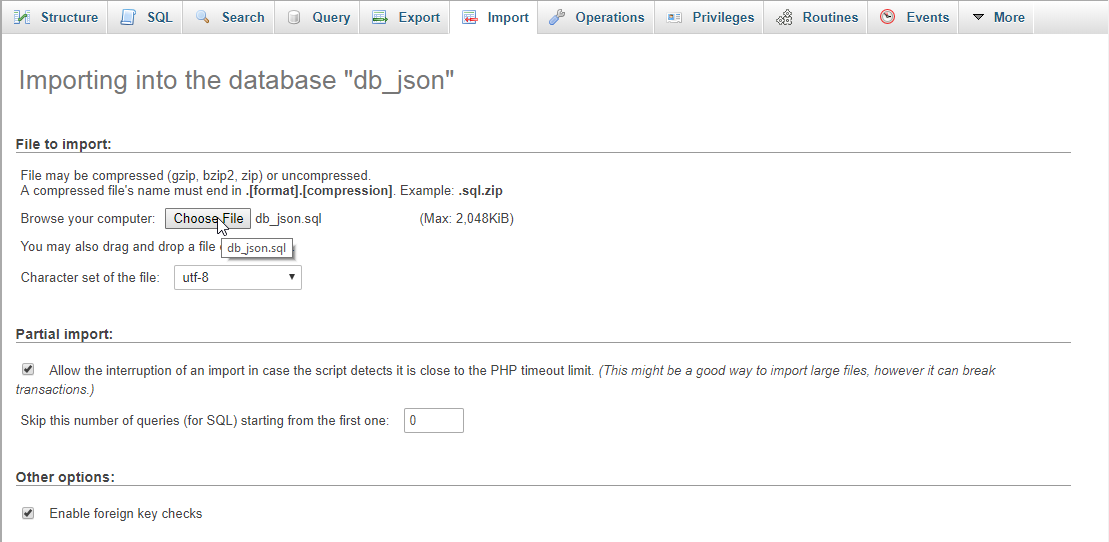
Creating the database connection
Open your any kind of text editor(notepad++, etc..). Then just copy/paste the code below then name it conn.php.- <?php
- if(!$conn){
- }
- ?>
Creating The Interface
This is where we will create a simple form for our application. To create the forms simply copy and write it into your text editor, then save it as index.php.- <!DOCTYPE html>
- <html lang="en">
- <head>
- <meta charset="UTF-8" name="viewport" content="width=device-width, initial-scale=1"/>
- <link rel="stylesheet" type="text/css" href="css/bootstrap.css" />
- </head>
- <body>
- <nav class="navbar navbar-default">
- <div class="container-fluid">
- <a class="navbar-brand" href="https://sourcecodester.com">Sourcecodester</a>
- </div>
- </nav>
- <div class="col-md-3"></div>
- <div class="col-md-6 well">
- <h3 class="text-primary">PHP - Retrieve MySQLi Data as JSON Format</h3>
- <hr style="border-top:1px dotted #ccc;"/>
- <button class="btn btn-success" data-toggle="modal" data-target="#form_modal"><span class="glyphicon glyphicon-plus"></span> Add member</button>
- <br /><br />
- <div class="col-md-6">
- <table class="table table-bordered">
- <thead class="alert-info">
- <tr>
- <th>Firstname</th>
- <th>Lastname</th>
- </tr>
- </thead>
- <tbody>
- <?php
- require 'conn.php';
- ?>
- <tr>
- <td><?php echo $fetch['firstname']?></td>
- <td><?php echo $fetch['lastname']?></td>
- </tr>
- <?php
- }
- ?>
- </tbody>
- <tfooter>
- <tr>
- <td colspan="2">
- <form method="POST">
- <button class="btn btn-primary" name="retrieve">Retrieve JSON</button>
- </form>
- </td>
- </tr>
- </tfooter>
- </table>
- </div>
- <div class="col-md-6" style="word-wrap:break-word; border:1px SOLID #ccc;">
- <?php include 'retrieve.php'?>
- </div>
- </div>
- <div id="form_modal" class="modal fade" aria-hidden="true">
- <div class="modal-dialog">
- <div class="modal-content">
- <form method="POST" action="save.php">
- <div class="modal-header">
- <h3 class="modal-title">Add member</h3>
- </div>
- <div class="modal-body">
- <div class="col-md-3"></div>
- <div class="col-md-6">
- <div class="form-group">
- <label>Firstname</label>
- <input type="text" name="firstname" class="form-control"/>
- </div>
- <div class="form-group">
- <label>Lastname</label>
- <input type="text" name="lastname" class="form-control"/>
- </div>
- </div>
- </div>
- <br style="clear:both;"/>
- <div class="modal-footer">
- <button type="button" data-dismiss="modal" class="btn btn-danger"><span class="glyphicon glyphicon-remove"></span> Close</button>
- <button class="btn btn-primary" name="save"><span class="glyphicon glyphicon-save"></span> Save</button>
- </div>
- </form>
- </div>
- </div>
- </div>
- <script src="js/jquery-3.2.1.min.js"></script>
- <script src="js/bootstrap.js"></script>
- </body>
- </html>
Creating PHP Query
This code contains the php query of the application. This code will save the data inputs to the MySQL database server. To do that just copy and write this block of codes inside the text editor, then save it as save.php.- <?php
- require_once 'conn.php';
- $firstname = $_POST['firstname'];
- $lastname = $_POST['lastname'];
- mysqli_query($conn, "INSERT INTO `member` VALUES('', '$firstname', '$lastname')") or die(mysqli_error());
- }
- ?>
Creating the Main Function
This code contains the main function of the application. This code will forcefully convert the MySQLi data into JSON array when the button is clicked. To do that just kindly copy and write these block of codes inside the text editor, then save it as retrieve.php- <?php
- require_once 'conn.php';
- $array[] = $fetch;
- }
- }
- ?>