How to Convert PHP Array into Javascript Array
Submitted by nurhodelta_17 on Thursday, April 19, 2018 - 21:24.
Creating our PHP Array
First we are going to create our PHP array.
The above code will create an array that looks like this:
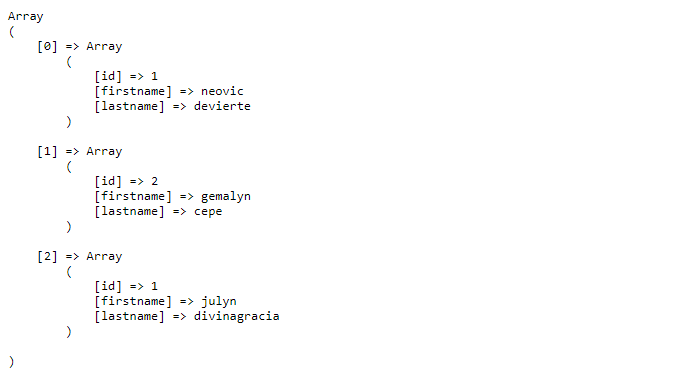
Converting into Javascript Array
Finally, we convert our created PHP array into Javascript array.- <script type="text/javascript">
- //converting php array to js array
- var array = <?php echo json_encode($array); ?>;
- console.log(array);
- </script>
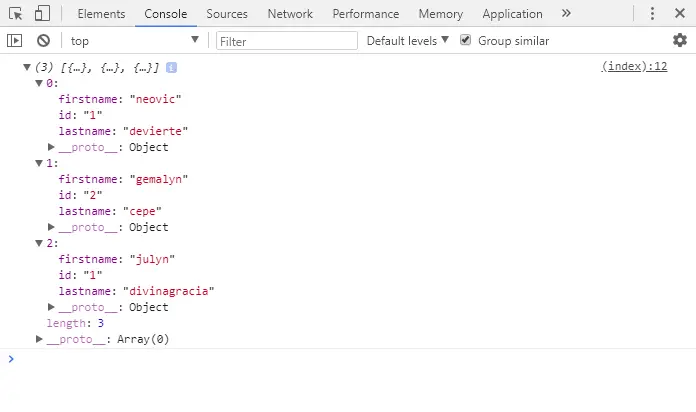
Full Source Code
Here's the Full Page Code.- <?php
- //create example array
- 'id' => '1',
- 'firstname' => 'neovic',
- 'lastname' => 'devierte'
- ),
- 'id' => '2',
- 'firstname' => 'gemalyn',
- 'lastname' => 'cepe'
- ),
- 'id' => '1',
- 'firstname' => 'julyn',
- 'lastname' => 'divinagracia'
- )
- );
- ?>
- <!DOCTYPE html>
- <html>
- <head>
- <meta charset="utf-8">
- <title></title>
- </head>
- <body>
- <script type="text/javascript">
- //converting php array to js array
- console.log(array);
- </script>
- </body>
- </html>