How to Add Google ReCaptcha
Submitted by nurhodelta_17 on Wednesday, April 11, 2018 - 21:27.
Creating our App
First step is to create our App or registering our App in the reCaptcha Admin Page of your Google Account. 1. Go to reCaptcha Admin Page and login with your Google Account. 2. On the Register a new site form, enter the ff values.Field | Value | Explaination |
---|---|---|
Label | localhost | We put localhost since we're using this server for development |
Choose the type of reCAPTCHA | reCAPTCHA v2 | This is what we are using |
Domains | localhost 127.0.0.1 | This is so we can use recaptcha in any localhost development |
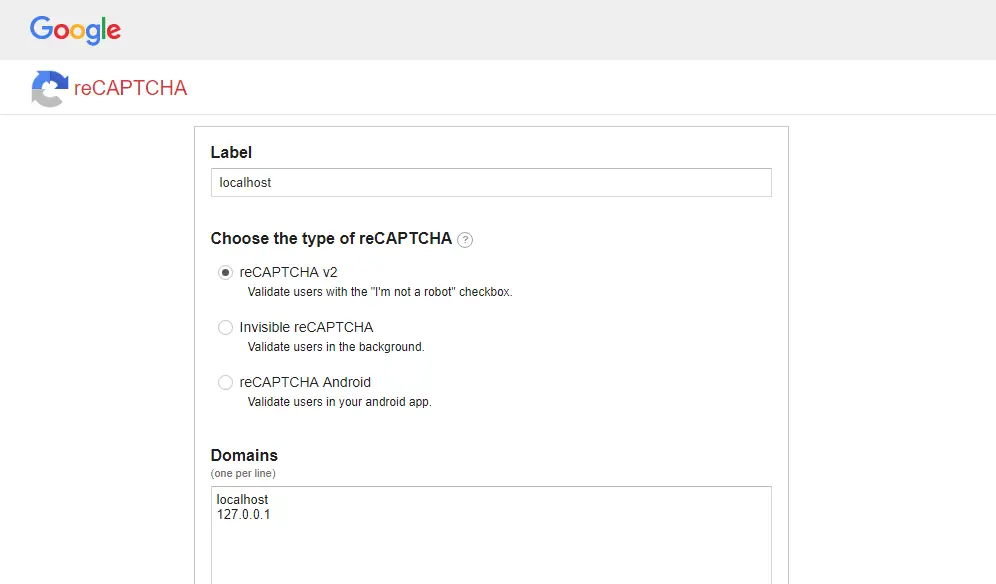
Integrating reCaptcha to our Application
First, we create the form where we want to put our recaptcha. In this tutorial, I wont be adding any more fields in the form except the recaptcha field. Create a new file, name it as index.php and paste the codes below.- <!DOCTYPE html>
- <html>
- <head>
- <meta charset="utf-8">
- <title>How to Add Google ReCaptcha</title>
- <link rel="stylesheet" type="text/css" href="bootstrap4/css/bootstrap.min.css">
- <script src='https://www.google.com/recaptcha/api.js'></script>
- <style type="text/css">
- .mt20{
- margin-top:20px;
- }
- .mt10{
- margin-top:10px;
- }
- </style>
- </head>
- <body>
- <div class="container">
- <h1 class="text-center mt20">How to Add Google reCaptcha</h1>
- <hr>
- <div class="row justify-content-md-center">
- <div class="col-sm-5">
- <?php
- echo "
- <div class='alert alert-danger text-center'>
- ".$_SESSION['error']."
- </div>
- ";
- }
- echo "
- <div class='alert alert-success text-center'>
- ".$_SESSION['success']."
- </div>
- ";
- }
- ?>
- <form method="POST" action="submit.php">
- <div class="g-recaptcha" data-sitekey="6LevO1IUAAAAAFX5PpmtEoCxwae-I8cCQrbhTfM6"></div>
- <button type="submit" class="btn btn-primary mt10" name="submit">Submit</button>
- </form>
- </div>
- </div>
- </div>
- </body>
- </html>
Submitting the reCaptcha Form
Lastly, we create the script if the visitor submitted the form and to check whether he correctly answers the recaptcha. Create a new file, name it as submit.php and paste the codes below.- <?php
- require('recaptcha/src/autoload.php');
- $recaptcha = new \ReCaptcha\ReCaptcha('6LevO1IUAAAAAFCCiOHERRXjh3VrHa5oywciMKcw', new \ReCaptcha\RequestMethod\SocketPost());
- $resp = $recaptcha->verify($_POST['g-recaptcha-response'], $_SERVER['REMOTE_ADDR']);
- if (!$resp->isSuccess()){
- $_SESSION['error'] = 'Please answer recaptcha correctly';
- }
- else{
- $_SESSION['success'] = 'Captcha Verified User';
- }
- }
- else{
- $_SESSION['error'] = 'Please verify that you are human';
- }
- }
- ?>