Creating a CodeIgniter Signup From with Email Verification
This tutorial tackles how to create a simple signup form with validation and email verification in CodeIgniter. CodeIgniter is a lightweight PHP framework that uses MVC(Model-View-Controller) architecture. Sending email verification is a way to check whether the users' inputted email is valid or not.
Installing CodeIgniter
If you don't have CodeIgniter installed yet, you can use this link to download the latest version of CodeIgniter which is 3.1.7 that I've used in this source code.
After downloading, extract the file in the folder of your server. Since I'm using XAMPP as my localhost server, I've put the folder in htdocs folder of my XAMPP.
Then, you can test whether you have successfully installed CodeIgniter by typing your app name in your browser. In my case, I named my app as codeigniter_register_email
so I'm using the below code.
- localhost/codeigniter_register_email
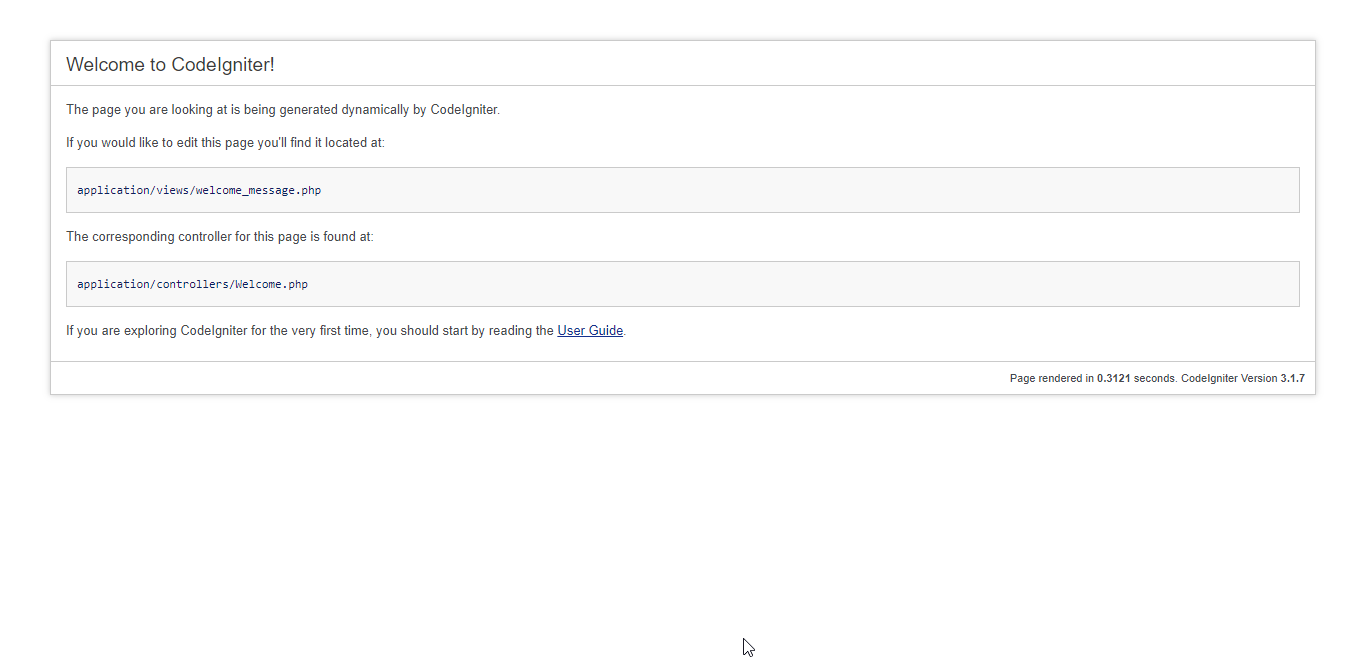
Removing index.php in our URLs
By default, index.php is included in every URL of our app. To remove this, please refer to my tutorial How to Remove index.php in the URL of Codeigniter Application.
Creating our Database
I've included a .sql file located in the DB folder in the downloadable of this tutorial. All you need to do is import the file into your phpMyAdmin. If you have no idea how to import, please refer to my tutorial How import .sql file to restore MySQL database.
You should be able to add a database named codeigniter.
Or you can create the database programmatically by creating a new database naming codeigniter
. After that, go to the SQL
tab in your PHPMyAdmin
and Copy/Paste
the code below. Then, click the Go
button.
Connecting our App into our Database
Next, we're going to connect our CodeIgniter application to the database that we created earlier.
- In your codeigniter app folder, open
database.php
located inapplication/config
folder. - Update
database.php
with your credential the same as what I did below.
- 'dsn' => '',
- 'hostname' => 'localhost',
- 'username' => 'root',
- 'password' => '',
- 'database' => 'codeigniter',
- 'dbdriver' => 'mysqli',
- 'dbprefix' => '',
- 'pconnect' => FALSE,
- 'db_debug' => (ENVIRONMENT !== 'production'),
- 'cache_on' => FALSE,
- 'cachedir' => '',
- 'char_set' => 'utf8',
- 'dbcollat' => 'utf8_general_ci',
- 'swap_pre' => '',
- 'encrypt' => FALSE,
- 'compress' => FALSE,
- 'stricton' => FALSE,
- 'save_queries' => TRUE
- );
Configuring our App
Next, we configure our base URL and remove index.php
on our index page.
- In your codeigniter app folder, open
config.php
located inapplication/config
folder. - Find and edit the ff lines:
- $config['index_page'] = '';
- $config['base_url'] = 'http://localhost/codeigniter_register_email/';
Defining our Routes
Next, we define our routes by naming our default controller and the route for our register.
Open routes.php
located in application/config
folder and update or add the ff lines.
- $route['default_controller'] = 'user';
- $route['register'] = 'user';
Creating our Model
Next, we create the model for our app. Take note that the first letter of your model name should be in a CAPITAL letter and the name of the model should be the same as the file name to avoid confusion.
Create a file named Users_model.php
in application/models folder of our app and put the ff codes:
- <?php
- class Users_model extends CI_Model {
- function __construct(){
- parent::__construct();
- $this->load->database();
- }
- public function getAllUsers(){
- $query = $this->db->get('users');
- return $query->result();
- }
- public function insert($user){
- $this->db->insert('users', $user);
- return $this->db->insert_id();
- }
- public function getUser($id){
- return $query->row_array();
- }
- public function activate($data, $id){
- $this->db->where('users.id', $id);
- return $this->db->update('users', $data);
- }
- }
Creating our Controller
The next step is to create our controller. Controllers follow the same naming convention as models.
Create a file named User.php
in application/controllers folder of our app and put the ff codes.
- <?php
- <?php
- class User extends CI_Controller {
- function __construct(){
- parent::__construct();
- $this->load->model('users_model');
- $this->load->library('form_validation');
- $this->load->library('session');
- //get all users
- $this->data['users'] = $this->users_model->getAllUsers();
- }
- public function index(){
- $this->load->view('register', $this->data);
- }
- public function register(){
- $this->form_validation->set_rules('email', 'Email', 'valid_email|required');
- $this->form_validation->set_rules('password', 'Password', 'required|min_length[7]|max_length[30]');
- $this->form_validation->set_rules('password_confirm', 'Confirm Password', 'required|matches[password]');
- if ($this->form_validation->run() == FALSE) {
- $this->load->view('register', $this->data);
- }
- else{
- //get user inputs
- $email = $this->input->post('email');
- $password = $this->input->post('password');
- //generate simple random code
- $set = '123456789abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ';
- //insert user to users table and get id
- $user['email'] = $email;
- $user['password'] = $password;
- $user['code'] = $code;
- $user['active'] = false;
- $id = $this->users_model->insert($user);
- //set up email
- 'protocol' => 'mail',
- 'smtp_host' => 'smtp.gmail.com',
- 'smtp_port' => 465,
- 'smtp_pass' => 'sourcecodester', // change it to yours
- 'mailtype' => 'html',
- 'wordwrap' => TRUE
- );
- $message = "
- <html>
- <head>
- <title>Verification Code</title>
- </head>
- <body>
- <h2>Thank you for Registering.</h2>
- <p>Your Account:</p>
- <p>Email: ".$email."</p>
- <p>Password: ".$password."</p>
- <p>Please click the link below to activate your account.</p>
- <h4><a href='".base_url()."user/activate/".$id."/".$code."'>Activate My Account</a></h4>
- </body>
- </html>
- ";
- $this->load->library('email', $config);
- $this->email->set_newline("\r\n");
- $this->email->from($config['smtp_user']);
- $this->email->to($email);
- $this->email->subject('Signup Verification Email');
- $this->email->message($message);
- //sending email
- if($this->email->send()){
- $this->session->set_flashdata('message','Activation code sent to email');
- }
- else{
- $this->session->set_flashdata('message', $this->email->print_debugger());
- }
- redirect('register');
- }
- }
- public function activate(){
- $id = $this->uri->segment(3);
- $code = $this->uri->segment(4);
- //fetch user details
- $user = $this->users_model->getUser($id);
- //if code matches
- if($user['code'] == $code){
- //update user active status
- $data['active'] = true;
- $query = $this->users_model->activate($data, $id);
- if($query){
- $this->session->set_flashdata('message', 'User activated successfully');
- }
- else{
- $this->session->set_flashdata('message', 'Something went wrong in activating account');
- }
- }
- else{
- $this->session->set_flashdata('message', 'Cannot activate account. Code didnt match');
- }
- redirect('register');
- }
- }
Creating our Register Form
Lastly, we create the register form of our app. Take note that I've used Bootstrap in the views. You may download bootstrap using this link.
Create the ff files inside the application/views
folder.
register.php
- <!DOCTYPE html>
- <html>
- <head>
- <meta charset="utf-8">
- <title>CodeIgniter Signup with Email Verification</title>
- <link rel="stylesheet" type="text/css" href="<?php echo base_url(); ?>bootstrap/css/bootstrap.min.css">
- </head>
- <body>
- <div class="container">
- <h1 class="page-header text-center">CodeIgniter Signup with Email Verification</h1>
- <div class="row">
- <div class="col-sm-4">
- <?php
- if(validation_errors()){
- ?>
- <div class="alert alert-info text-center">
- <?php echo validation_errors(); ?>
- </div>
- <?php
- }
- if($this->session->flashdata('message')){
- ?>
- <div class="alert alert-info text-center">
- <?php echo $this->session->flashdata('message'); ?>
- </div>
- <?php
- }
- ?>
- <h3 class="text-center">Signup Form</h3>
- <form method="POST" action="<?php echo base_url().'user/register'; ?>">
- <div class="form-group">
- <label for="email">Email:</label>
- <input type="text" class="form-control" id="email" name="email" value="<?php echo set_value('email'); ?>">
- </div>
- <div class="form-group">
- <label for="password">Password:</label>
- <input type="password" class="form-control" id="password" name="password" value="<?php echo set_value('password'); ?>">
- </div>
- <div class="form-group">
- <label for="password_confirm">Confirm Password:</label>
- <input type="password" class="form-control" id="password_confirm" name="password_confirm" value="<?php echo set_value('password_confirm'); ?>">
- </div>
- <button type="submit" class="btn btn-primary">Register</button>
- </form>
- </div>
- <div class="col-sm-8">
- <h3 class="text-center">Users Table</h3>
- <table class="table table-bordered table-striped">
- <thead>
- <tr>
- <th>UserID</th>
- <th>Email</th>
- <th>Password</th>
- <th>Code</th>
- <th>Active</th>
- </tr>
- </thead>
- <tbody>
- <?php
- foreach($users as $row){
- ?>
- <tr>
- <td><?php echo $row->id; ?></td>
- <td><?php echo $row->email; ?></td>
- <td><?php echo $row->password; ?></td>
- <td><?php echo $row->code; ?></td>
- <td><?php echo $row->active ? 'True' : 'False'; ?></td>
- </tr>
- <?php
- }
- ?>
- </tbody>
- </table>
- </div>
- </div>
- </div>
- </body>
- </html>
Demo
That ends this tutorial. Test your work if it is working. You can also download my working sample source code that I have made for this tutorial. The download button is located below.
Explore on this website for more tutorials and free source code.
Happy Coding :)