CodeIgniter Send Email using SMTP
Submitted by nurhodelta_17 on Friday, February 23, 2018 - 21:43.
Installing CodeIgniter
If you don't have CodeIgniter installed yet, you can use this link to download the latest version of CodeIgniter which is 3.1.7 that I've used in this tutorial. After downloading, extract the file in the folder of your server. Since I'm using XAMPP as my localhost server, I've put the folder in htdocs folder of my XAMPP. Then, you can test whether you have successfully installed codeigniter by typing your app name in your browser. In my case, I named my app as codeigniter_email so I'm using the below code.- localhost/codeigniter_email
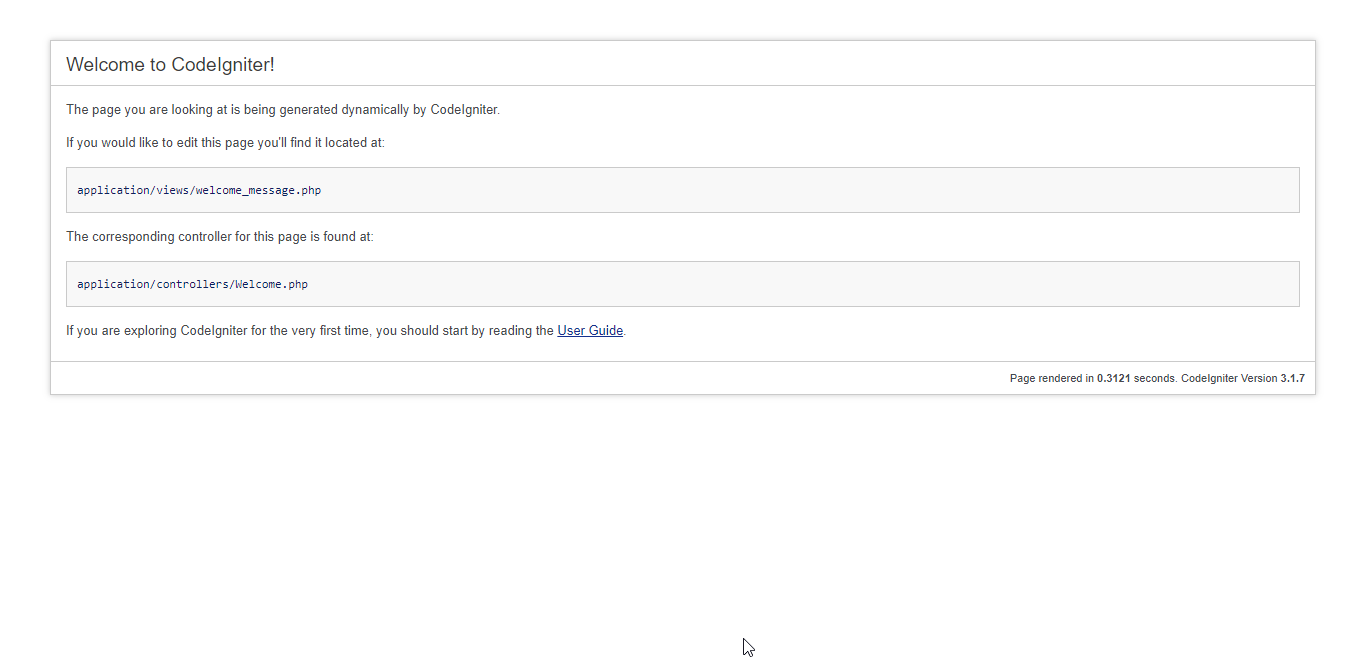
Configuring our Base URL
Next, we configure our base url to tell codeigniter that this is the URL of our site/application. We're gonna be using this a lot that's why we need to configure this. 1. In your codeigniter app folder, open config.php located in application/config folder. 2. Find and edit the ff line:- $config['base_url'] = 'http://localhost/codeigniter_email';
Removing index.php in our Index
Next step is to remove index.php in all of our URLs. To do this, we need an .htaccess file. Please visit my tutorial How to remove index.php in CodeIgniter URL to further understand the steps.Creating our Controller
Next, we are going to create our controller. Create a file named Email.php in application/controllers folder of our app and put the ff codes.- <?php
- class Email extends CI_Controller {
- function __construct(){
- parent::__construct();
- $this->load->helper('url');
- $this->load->library('session');
- }
- public function index(){
- $this->load->view('email_form');
- }
- public function sendemail(){
- $subject = $this->input->post('subject');
- $message = $this->input->post('message');
- $email = $this->input->post('email');
- 'protocol' => 'smtp',
- 'smtp_host' => 'ssl://smtp.googlemail.com',
- 'smtp_port' => 465,
- 'smtp_pass' => 'mysourcepass', // change it to yours
- 'mailtype' => 'html',
- 'charset' => 'iso-8859-1',
- 'wordwrap' => TRUE
- );
- $this->load->library('email', $config);
- $this->email->set_newline("\r\n");
- $this->email->from($config['smtp_user']); // change it to yours
- $this->email->to($email);// change it to yours
- $this->email->subject($subject);
- $this->email->message($message);
- if($this->email->send()){
- $this->session->set_flashdata('message', 'Email sent');
- }
- else{
- $this->session->set_flashdata('message', show_error($this->email->print_debugger()));
- }
- redirect('/');
- }
- }
Defining our Default Controller
Next, we are going to set our default controller so that if no controller is defined, this default controller will be used. Open routes.php located in application/config folder and set the default route to our user controller.- $route['default_controller'] = 'email';
Creating our Email Form
Lastly, we create the form where we get data to include in our email. Create the ff file inside application/views folder. email_form.php- <!DOCTYPE html>
- <html>
- <head>
- <meta charset="utf-8">
- <title>CodeIgniter Sending Email</title>
- <link rel="stylesheet" type="text/css" href="<?php echo base_url(); ?>bootstrap/css/bootstrap.min.css">
- </head>
- <body>
- <div class="container">
- <h1 class="page-header text-center">CodeIgniter Sending Email</h1>
- <div class="row">
- <div class="col-sm-4 col-sm-offset-4">
- <?php
- if($this->session->flashdata('message')){
- ?>
- <div class="alert alert-info text-center">
- <?php echo $this->session->flashdata('message'); ?>
- </div>
- <?php
- }
- ?>
- <form method="POST" action="<?php echo base_url(); ?>email/sendemail">
- <div class="form-group">
- <label>Subject:</label>
- <input type="text" class="form-control" name="subject">
- </div>
- <div class="form-group">
- <label>Message:</label>
- <textarea class="form-control" name="message"></textarea>
- </div>
- <div class="form-group">
- <label>Email:</label>
- <input type="text" class="form-control" name="email">
- </div>
- <button type="submit" class="btn btn-primary">Send</button>
- </form>
- </div>
- </div>
- </div>
- </body>
- </html>