Vue.js User Registration/Sign up with PHP/MySQLi
Submitted by nurhodelta_17 on Wednesday, December 13, 2017 - 15:45.
Getting Started
I've use Bootstrap CDN in this tutorial, so you need internet connection for it to work.Creating our Database
1. Open phpMyAdmin. 2. Click databases, create a database and name it as vuetot. 3. After creating a database, click the SQL and paste the below codes. See image below for detailed instruction.- CREATE TABLE `users` (
- `userid` INT(11) NOT NULL AUTO_INCREMENT,
- `email` VARCHAR(150) NOT NULL,
- `password` VARCHAR(50) NOT NULL,
- PRIMARY KEY(`userid`)
- ) ENGINE=InnoDB DEFAULT CHARSET=latin1;
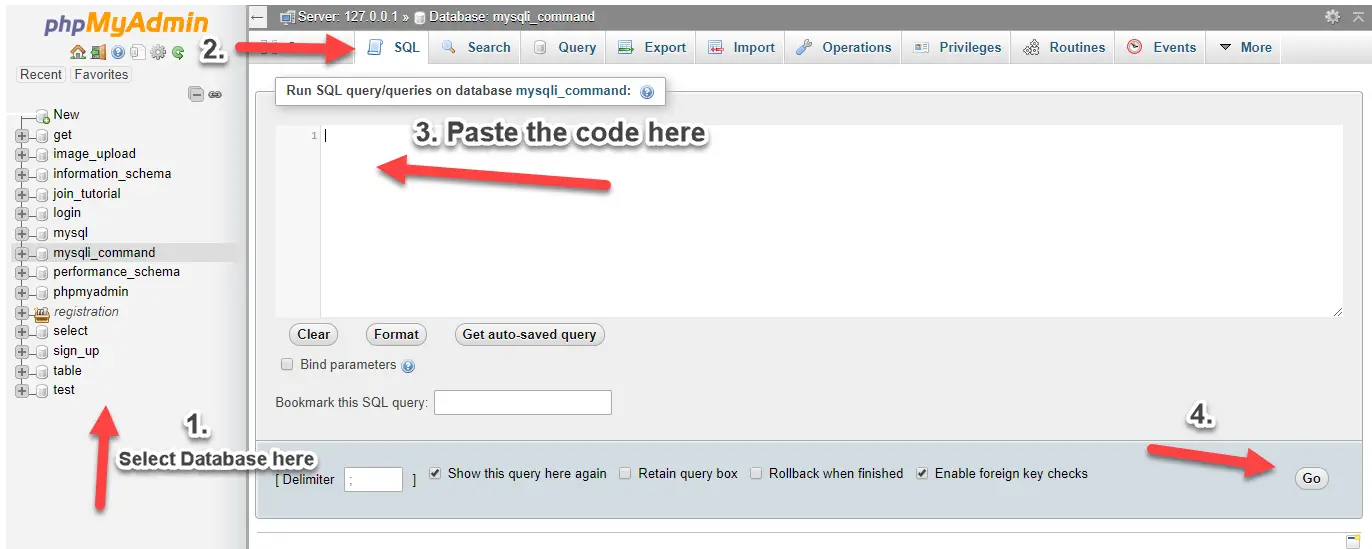
index.php
This is our index which contains our registration form and also our users table for you to check if registration is successful.- <!DOCTYPE html>
- <html>
- <head>
- <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css">
- </head>
- <body>
- <div class="container">
- <div id="register">
- <div class="col-md-4">
- <div class="panel panel-primary">
- <div class="panel-body">
- <input type="text" class="form-control" ref="email" v-model="regDetails.email" v-on:keyup="keymonitor">
- <div class="text-center" v-if="errorEmail">
- </div>
- <input type="password" class="form-control" ref="password" v-model="regDetails.password" v-on:keyup="keymonitor">
- <div class="text-center" v-if="errorPassword">
- </div>
- </div>
- <div class="panel-footer">
- </div>
- </div>
- <div class="alert alert-danger text-center" v-if="errorMessage">
- </div>
- <div class="alert alert-success text-center" v-if="successMessage">
- </div>
- </div>
- <div class="col-md-8">
- <table class="table table-bordered table-striped">
- <thead>
- </thead>
- <tbody>
- <tr v-for="user in users">
- </tr>
- </tbody>
- </table>
- </div>
- </div>
- </div>
- </body>
- </html>
app.js
- var app = new Vue({
- el: '#register',
- data:{
- successMessage: "",
- errorMessage: "",
- errorEmail: "",
- errorPassword: "",
- users: [],
- regDetails: {email: '', password: ''},
- },
- mounted: function(){
- this.getAllUsers();
- },
- methods:{
- getAllUsers: function(){
- axios.get('api.php')
- .then(function(response){
- if(response.data.error){
- app.errorMessage = response.data.message;
- }
- else{
- app.users = response.data.users;
- }
- });
- },
- userReg: function(){
- var regForm = app.toFormData(app.regDetails);
- axios.post('api.php?action=register', regForm)
- .then(function(response){
- console.log(response);
- if(response.data.email){
- app.errorEmail = response.data.message;
- app.focusEmail();
- app.clearMessage();
- }
- else if(response.data.password){
- app.errorPassword = response.data.message;
- app.errorEmail='';
- app.focusPassword();
- app.clearMessage();
- }
- else if(response.data.error){
- app.errorMessage = response.data.message;
- app.errorEmail='';
- app.errorPassword='';
- }
- else{
- app.successMessage = response.data.message;
- app.regDetails = {email: '', password:''};
- app.errorEmail='';
- app.errorPassword='';
- app.getAllUsers();
- }
- });
- },
- focusEmail: function(){
- this.$refs.email.focus();
- },
- focusPassword: function(){
- this.$refs.password.focus();
- },
- keymonitor: function(event) {
- if(event.key == "Enter"){
- app.userReg();
- }
- },
- toFormData: function(obj){
- var form_data = new FormData();
- for(var key in obj){
- form_data.append(key, obj[key]);
- }
- return form_data;
- },
- clearMessage: function(){
- app.errorMessage = '';
- app.successMessage = '';
- }
- }
- });
api.php
This is our PHP API that contains our registration code.- <?php
- $conn = new mysqli("localhost", "root", "", "vuetot");
- if ($conn->connect_error) {
- }
- $action = 'read';
- $action = $_GET['action'];
- }
- if($action == 'read'){
- $sql = "select * from users";
- $query = $conn->query($sql);
- while($row = $query->fetch_array()){
- }
- $out['users'] = $users;
- }
- if($action == 'register'){
- function check_input($data) {
- return $data;
- }
- $email = check_input($_POST['email']);
- $password = check_input($_POST['password']);
- if($email==''){
- $out['email'] = true;
- $out['message'] = "Email is required";
- }
- $out['email'] = true;
- $out['message'] = "Invalid Email Format";
- }
- elseif($password==''){
- $out['password'] = true;
- $out['message'] = "Password is required";
- }
- else{
- $sql="select * from users where email='$email'";
- $query=$conn->query($sql);
- if($query->num_rows > 0){
- $out['email'] = true;
- $out['message'] = "Email already exist";
- }
- else{
- $sql = "insert into users (email, password) values ('$email', '$password')";
- $query = $conn->query($sql);
- if($query){
- $out['message'] = "User Added Successfully";
- }
- else{
- $out['error'] = true;
- $out['message'] = "Could not add User";
- }
- }
- }
- }
- $conn->close();
- ?>