Simple Chat using PHP, MySQLi, AJAX and JQuery
Submitted by nurhodelta_17 on Wednesday, August 30, 2017 - 21:41.
In this tutorial, I'm going to show you how to create a simple chat using PHP, MySQLi, AJAX and JQuery. I have created a sample chat room and sample users to focus this tutorial on creating a simple chat. Also, I have created a simple login, but if you want, you may learn How to Create a Login with Validation.
Creating our Database
First, we're going to create our database to hold our sample data and our chats. 1. Open phpMyAdmin. 2. Click databases, create a database and name it as "chat". 3. After creating a database, click the SQL and paste the below codes. See image below for detailed instruction.- CREATE TABLE `chat` (
- `chatid` INT(11) NOT NULL AUTO_INCREMENT,
- `chat_room_id` INT(11) NOT NULL,
- `chat_msg` VARCHAR(100) NOT NULL,
- `userid` INT(11) NOT NULL,
- `chat_date` datetime NOT NULL,
- PRIMARY KEY(`chatid`)
- ) ENGINE=InnoDB DEFAULT CHARSET=latin1;
- CREATE TABLE `chat_room` (
- `chat_room_id` INT(11) NOT NULL AUTO_INCREMENT,
- `chat_room_name` VARCHAR(50) NOT NULL,
- PRIMARY KEY(`chat_room_id`)
- ) ENGINE=InnoDB DEFAULT CHARSET=latin1;
- CREATE TABLE `user` (
- `userid` INT(11) NOT NULL AUTO_INCREMENT,
- `username` VARCHAR(30) NOT NULL,
- `password` VARCHAR(30) NOT NULL,
- `your_name` VARCHAR(60) NOT NULL,
- PRIMARY KEY(`userid`)
- ) ENGINE=InnoDB DEFAULT CHARSET=latin1;
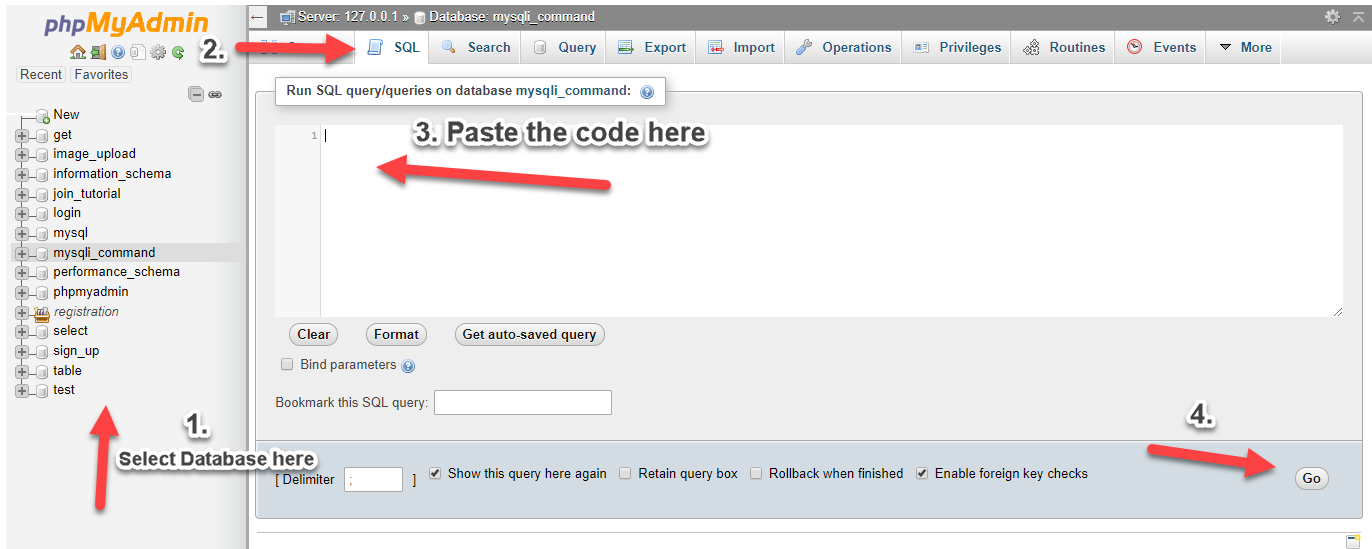
Inserting Data into our Database
Next, we insert data into our database that will serve as our reference in this tutorial. 1. Click our database "chat". 2. Click SQL and paste the below codes.- INSERT INTO `chat_room` (`chat_room_name`) VALUES
- ('Sample Chat Room');
- INSERT INTO `user` (`username`, `password`, `your_name`) VALUES
- ('neovic', 'devierte', 'neovic'),
- ('lee', 'ann', 'lee');
Creating our Connection
Next step is to create a database connection and save it as "conn.php". This file will serve as our bridge between our form and our database. To create the file, open your HTML code editor and paste the code below after the tag.- <?php
- // Check connection
- {
- }
- ?>
Creating our Login Page
Next, we create a login page to determine our user that is needed when chatting. We name this as "index.php".- <!DOCTYPE html>
- <html>
- <head>
- <title>Simple Chat using PHP/MySQLi, Ajax/JQuery</title>
- </head>
- <body>
- <h2>Login Here</h2>
- <form method="POST" action="login.php">
- Username: <input type="text" name="username">
- Password: <input type="password" name="password"> <br><br>
- <input type="submit" value="Login">
- </form><br>
- <?php
- echo $_SESSION['message'];
- }
- ?>
- </body>
- </html>
Creating our Login Code
Next, we create the code for our login. We name this as "login.php".- <?php
- include('conn.php');
- $username=$_POST['username'];
- $password=$_POST['password'];
- $query=mysqli_query($conn,"select * from `user` where username='$username' and password='$password'");
- $_SESSION['message']="Login Error. Please Try Again";
- }
- else{
- $_SESSION['userid']=$row['userid'];
- }
- ?>
Creating our Homepage
This page will serve as a goto page after successful login. This is the page that the user can chat in the sample chat room. We name this as "home.php". This page also has our jquery script for chatting.- <?php
- include('conn.php');
- }
- ?>
- <!DOCTYPE html>
- <html>
- <head>
- <title>Simple Chat using PHP/MySQLi, Ajax/JQuery</title>
- </head>
- <body>
- <div>
- <h4>Welcome, <?php echo $urow['your_name']; ?> <a href="logout.php">Logout</a></h4>
- <?php
- ?>
- <div>
- Chat Room Name: <?php echo $row['chat_room_name']; ?><br><br>
- </div>
- <div id="result" style="overflow-y:scroll; height:300px;"></div>
- <form>
- <input type="text" id="msg">
- <input type="hidden" value="<?php echo $row['chat_room_id']; ?>" id="id">
- <button type="button" id="send_msg">Send</button>
- </form>
- <?php
- }
- ?>
- </div>
- <script src = "jquery-3.1.1.js"></script>
- <script type = "text/javascript">
- $(document).ready(function(){
- displayResult();
- /* Send Message */
- $('#send_msg').on('click', function(){
- if($('#msg').val() == ""){
- alert('Please write message first');
- }else{
- $msg = $('#msg').val();
- $id = $('#id').val();
- $.ajax({
- type: "POST",
- url: "send_message.php",
- data: {
- msg: $msg,
- id: $id,
- },
- success: function(){
- displayResult();
- }
- });
- }
- });
- /***** *****/
- });
- function displayResult(){
- $id = $('#id').val();
- $.ajax({
- url: 'send_message.php',
- type: 'POST',
- async: false,
- data:{
- id: $id,
- res: 1,
- },
- success: function(response){
- $('#result').html(response);
- }
- });
- }
- </script>
- </body>
- </html>
Creating our Send Message Code
Next step is to create our code for sending our chat message. We name this code as "send_message.php".- <?php
- include ('conn.php');
- $id = $_POST['id'];
- mysqli_query($conn,"insert into `chat` (chat_room_id, chat_msg, userid, chat_date) values ('$id', '$msg' , '".$_SESSION['userid']."', NOW())") or die(mysqli_error());
- }
- ?>
- <?php
- $id = $_POST['id'];
- ?>
- <?php
- $query=mysqli_query($conn,"select * from `chat` left join `user` on user.userid=chat.userid where chat_room_id='$id' order by chat_date asc") or die(mysqli_error());
- ?>
- <div>
- <?php echo $row['your_name']; ?>: <?php echo $row['chat_msg']; ?><br>
- </div>
- <br>
- <?php
- }
- }
- ?>
Creating our Logout
Lastly, we create our logout code.- <?php
- ?>