MySQL Edit & Delete Live Update
Submitted by rinvizle on Friday, September 9, 2016 - 23:17.
In this tutorial we will create a MySQL Edit & Delete Live Update in PHP. This project is written in PHP and MySQL. Its a real-time process of adding, deleting and update the data of each users. The system connects to the database to pull the result and display the data to the page so the users will see their detail's in front of them.
Edit.php - This script is for the edit of the existing users by their users id.
Dbcon.php - Is for the connection of database.
For more programming tutorials don't forget to Like & Share for more update's. Hope that you learn from this tutorial. Enjoy Coding!
Sample Code
Index.php - This file is for the display of form and UI for the users.- <?php
- include('dbcon.php');
- ?>
- <!DOCTYPE html>
- <html>
- <head>
- <title>MySQL Edit & Delete Live Update</title>
- <link rel="stylesheet" type="text/css" href="style.css" />
- </head>
- <body>
- <center><h1 class="header-text">MySQL Edit & Delete Live Update</h1></center>
- <form method="post" action="add.php">
- <div>
- <label for="fname">First Name</label>
- <input type="text" id="fname" name="Firstname">
- <label for="mname">Middle Name</label>
- <input type="text" id="mname" name="Maiden">
- <label for="lname">Lastname</label>
- <input type="text" id="lname" name="Lastname">
- <label for="email">Email Address</label>
- <input type="text" id="email" name="Emailadd">
- <input type="submit" name="submit" value="ADD">
- </div>
- </form>
- </body>
- </html>
For the query of data from the database through the php form.
- <table>
- <?php
- ?>
- <tr>
- <th>Firstname</th>
- <th>Middle Name</th>
- <th>Lastname</th>
- <th>Email Address</th>
- <th>Update</th>
- </tr>
- <tr>
- <td><?php echo $user_rows['Firstname'] ; ?></td>
- <td><?php echo $user_rows['Maiden'] ; ?></td>
- <td><?php echo $user_rows['Lastname'] ; ?></td>
- <td><?php echo $user_rows['Emailadd'] ; ?></td>
- <td><a href="delete.php<?php echo '?id='.$user_rows['UserID']; ?>"><button class="button1">DELETE</button></a>
- <a href="edit.php<?php echo '?id='.$user_rows['UserID']; ?>"><button class="button2">EDIT</button></a>
- </td>
- </tr>
- <?php }?>
- </table>
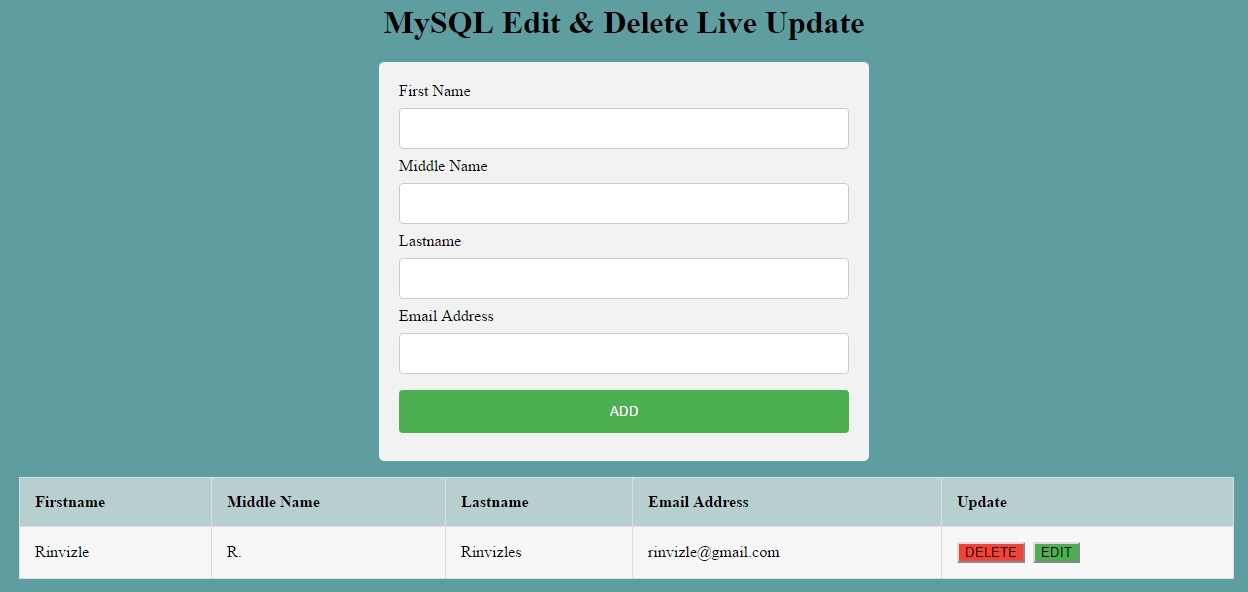
- <?php
- $Firstname=$_POST['Firstname'];
- $Maiden=$_POST['Maiden'];
- $Lastname=$_POST['Lastname'];
- $Emailadd=$_POST['Emailadd'];
- mysql_query("update users_name set Firstname='$Firstname',Maiden='$Maiden',Lastname='$Lastname',Emailadd='$Emailadd' where UserID='$id'");
- }
- ?>
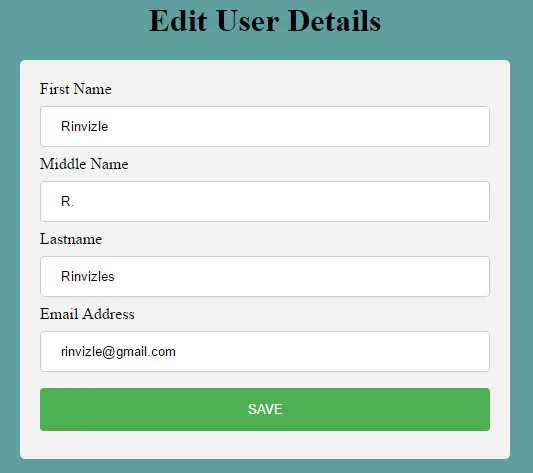
- <?php
- ?>