Captcha Generator
Submitted by rinvizle on Wednesday, July 27, 2016 - 16:14.
This tutorial we will create a Capthcha Generator. And this simple project would be very helpful for your programming projects. Captcha Generator or a full form of CAPTCHA is telling humans and computers apart automatically. Captcha is a program which helps to protect your website from bots and determine the user is human or not. So, you need to add Captcha for protecting contact form, comment form and any other forms from spam. But many times you need to add a simple PHP captcha in form. This tutorial helps you to implement Captcha functionality using PHP in your website.
Index.php this file is used to display the form and has a PHP Captcha script to provide and validate the captcha code.
Hope that you learn in this project and enjoy coding. Don't forget to LIKE & SHARE this website.
Sample Code
Captchagenerator.php we have created a Captcha PHP class which provides you highly customization option for PHP captcha. Using Captchagenerator script you can easily add captcha to the form. In the main Captchagenerator class contains 2 functions createCaptcha() and hexToRgb(). hexToRgb() function is used by createCaptcha() function, so you should need to call only createCaptcha() function.- <?php
- class Captchagenerator
- {
- var $word = '0123456789abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ';
- var $length = 5;
- var $img_width = 160;
- var $img_height = 50;
- var $font_path = '';
- var $font_size = 25;
- var $expiration = 7200;
- var $bg_color = '#ffffff';
- var $border_color = '#996666';
- var $text_color = '#cc9999';
- var $grid_color = '#ffb6b6';
- var $shadow_color = '#fff0f0';
- foreach ($config as $key => $val){
- $method = 'set_'.$key;
- $this->$method($val);
- }else{
- $this->$key = $val;
- }
- }
- }
- }
- return FALSE;
- }
- }
- public function createCaptcha(){
- $str = '';
- for ($i = 0; $i < $this->length; $i++){
- }
- $word = $str;
- }else{
- }
- $bgColorRgb = $this->hexToRgb($this->bg_color);
- $borderColorRgb = $this->hexToRgb($this->border_color);
- $textColorRgb = $this->hexToRgb($this->text_color);
- $gridColorRgb = $this->hexToRgb($this->grid_color);
- $shadowColorRgb = $this->hexToRgb($this->shadow_color);
- $border_color = imagecolorallocate ($im, $borderColorRgb[0], $borderColorRgb[1], $borderColorRgb[2]);
- $shadow_color = imagecolorallocate($im, $shadowColorRgb[0], $shadowColorRgb[1], $shadowColorRgb[2]);
- $theta = 1;
- $thetac = 7;
- $radius = 16;
- $circles = 20;
- $points = 32;
- for ($i = 0; $i < ($circles * $points) - 1; $i++){
- $theta = $theta + $thetac;
- $rad = $radius * ($i / $points );
- $theta = $theta + $thetac;
- $rad1 = $radius * (($i + 1) / $points);
- $theta = $theta - $thetac;
- }
- $use_font = ($this->font_path != '' AND file_exists($this->font_path) AND function_exists('imagettftext')) ? TRUE : FALSE;
- $y = $this->font_size+2;
- {
- if ($use_font == FALSE){
- $x += ($this->font_size);
- }else{
- imagettftext($im, $this->font_size, $angle, $x, $y, $text_color, $this->font_path, substr($word, $i, 1));
- $x += $this->font_size;
- }
- }
- $_SESSION['captchaCode'] = $word;
- }
- public function hexToRgb($hex){
- } else {
- }
- return $rgb;
- }
- }
- ?>
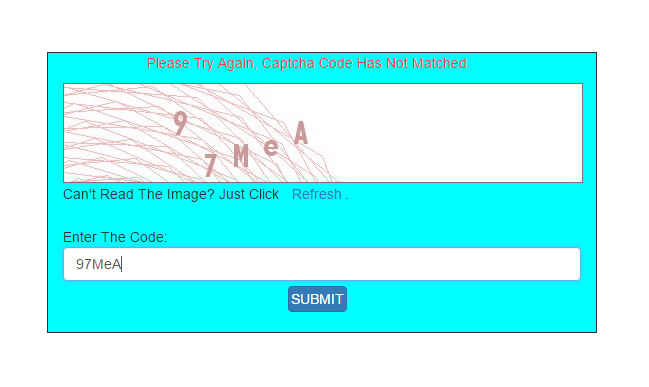
- <?php
- $captchaCode = $_SESSION['captchaCode'];
- $enteredcaptchaCode = $_POST['captcha_code'];
- if($enteredcaptchaCode === $captchaCode){
- $succMsg = '                                         Captcha Code Has Matched.';
- }else{
- $errMsg = '                     Please Try Again, Captcha Code Has Not Matched.';
- }
- }else{
- $errMsg = 'Please Enter The Captcha Code.';
- }
- }
- ?>
- <!DOCTYPE html>
- <html>
- <head>
- <meta charset="UTF-8" />
- <title>Captcha Generator</title>
- <link rel="stylesheet" href="bootstrap/css/bootstrap.min.css">
- <link rel="stylesheet" href="bootstrap/css/bootstrap.css">
- <script src="js/jquery.min.js"></script>
- </head>
- <body>
- <div class="container">
- <div>
- <img src="captcha.php" id="capImage"/>
- <br/>Can't Read The Image? Just Click<a href="javascript:void(0);" onclick="javascript:$('#capImage').attr('src','captcha.php');"><button class="btn btn-link">Refresh</button></a>.
- <form method="post">
- <br/>Enter The Code:<input class="form-control" name="captcha_code" type="text" value="">
- <input type="submit" class="btn btn-primary" name="submit" value="SUBMIT">
- </form><br/>
- </div>
- </body>
- </html>
Add new comment
- 611 views