In this tutorial, we are going to learn on
How To Display Data From Database Table In PHP/MySQL Using PDO Query. You can use this source code to merge the last tutorial that I made and it's called
Registration Form In PHP/MySQL Using PDO Query.
This source code will help us on how to show data from the Database using PDO Query.
Let's start with:
Creating our Table
We are going to make our database.
To create a database:
- Open the PHPMyAdmin.
- Create a database and name it as "registration_pdo".
- After creating a database name, then we are going to create our table. And name it as "user_registration".
Kindly copy the code below.
--
-- Table structure for table `user_registration`
--
CREATE TABLE `user_registration` (
`user_id` INT(11) NOT NULL,
`first_name` VARCHAR(100) NOT NULL,
`last_name` VARCHAR(100) NOT NULL,
`user_name` VARCHAR(100) NOT NULL,
`password` VARCHAR(100) NOT NULL
) ENGINE=InnoDB DEFAULT CHARSET=latin1;
Data in the Database.
--
-- Dumping data for table `user_registration`
--
INSERT INTO `user_registration` (`user_id`, `first_name`, `last_name`, `user_name`, `password`) VALUES
(1, 'Jane', 'Doe', 'jane24', 'doe23jd'),
(2, 'John', 'Doe', 'john43', 'john_doe325kh'),
(3, 'Sarah', 'Geronimow', 'sarahGero', 'geronimow243'),
(4, 'Super', 'Man', 'returns', 'superman_returns');
We are going to make our database connection.
Database Connection
Copy and paste this then save it as
"connection.php".
<?php
$db_server = "localhost";
$db_username = "root";
$db_password = "";
$db_database = "registration_pdo";
$conn = new PDO("mysql:host=$db_server;dbname=$db_database", $db_username, $db_password);
$conn->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);
?>
Then, we are creating a new page where our data is to display.
Display Page
This source code for displaying data in the database table and save it as
"index.php".
<table border="1" cellspacing="5" cellpadding="5" width="100%">
<thead>
<tr>
<th>No.</th>
<th>First Name</th>
<th>Last Name</th>
<th>User Name</th>
<th>Password</th>
</tr>
</thead>
<tbody>
<?php
require_once('connection.php');
$result = $conn->prepare("SELECT * FROM user_registration ORDER BY user_id ASC");
$result->execute();
for($i=0; $row = $result->fetch(); $i++){
?>
<tr>
<td><label><?php echo $row['user_id']; ?></label></td>
<td><label><?php echo $row['first_name']; ?></label></td>
<td><label><?php echo $row['last_name']; ?></label></td>
<td><label><?php echo $row['user_name']; ?></label></td>
<td><label><?php echo $row['password']; ?></label></td>
</tr>
<?php } ?>
</tbody>
</table>
And, this is the style.
<style type="text/css">
body {
width:800px;
border:red 1px solid;
border-style:dashed;
margin:auto;
padding:10px;
}
td {
text-align:center;
padding:10px;
}
table {
margin:auto;
border:blue 1px solid;
}
label {
font-size:18px;
color:blue;
font-weight: bold;
font-family: cursive;
}
h2 {
color:red;
text-align:center;
}
th {
color:red;
font-size:20px;
font-family: cursive;
}
</style>
Output:
You can see in the image lists of data in the Database.
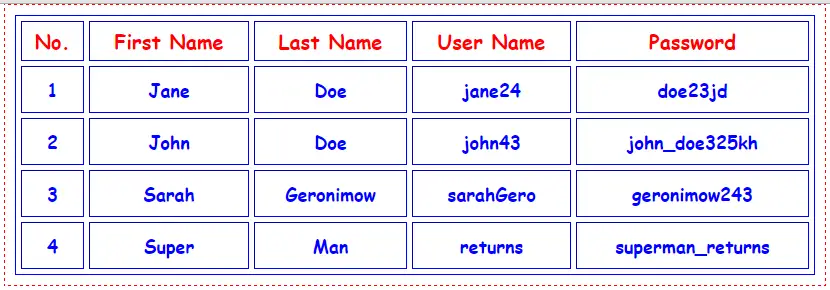
This is all the steps on
How To Display Data From Database Table In PHP/MySQL Using PDO Query. So, this is it, or you can download the full source code below by clicking the
"Download Code" button below.
Share us your thoughts and comments below. Thank you so much for dropping by and reading this tutorial post. For more updates, don’t hesitate and feel free to visit this website more often and please share this with your friends or email me at
[email protected]. Practice Coding. Thank you very much.