Getting Started
First we need Bootstrap 4 and jQuery. These are included in the downloadable of this tutorial but if you want, you can download them yourself using the links below.
For Bootstrap 4
For jQuery 3.3.1
Creating our Form
Next, we create the form which contains our password field.
Create a new file, name it as
index.html and paste the codes below.
<!DOCTYPE html>
<title>How to Create a Password Strength Meter using jQuery
</title>
<link rel="stylesheet" type="text/css" href="bootstrap4/css/bootstrap.min.css">
<link rel="stylesheet" type="text/css" href="assets/strength.css">
<h1 class="text-center" style="margin-top:30px;">Password Strength Meter using jQuery
</h1>
<div class="row justify-content-center">
<input type="password" name="password" id="password" class="form-control" placeholder="input Password">
<div id="progress" class="progress-bar" role="progressbar" aria-valuemin="0" aria-valuemax="100"></div>
Creating our CSS
Next, we create our custom CSS for the strength of our password.
Create a new file, name it as
strength.css and paste the codes below.
.short{
color:#d9534f;
}
.weak{
color:#5bc0de;
}
.good{
color:#428bca;
}
.strong{
color:#5cb85c;
}
#strength{
font-weight:bold;
font-size:larger;
}
Creating our jQuery Scripts
Lastly, we create our jquery scripts that contains our code that checks the strength of the inputted password.
Create a new file, name it as
strength.js and paste the codes below.
$(function(){
$('#password').keyup(function(){
var password = $('#password').val();
if(password == ''){
$('#progress').removeClass().addClass('progress-bar');
$('#strength').html('');
}
else{
var meter = checkStrength(password);
$('#strength').html(meter);
}
});
});
function checkStrength(password){
var strength = 0
if (password.length < 6) {
$('#progress').removeClass().addClass('progress-bar').addClass('w-25').addClass('bg-danger');
$('#strength').removeClass().addClass('short');
return 'Too short';
}
if (password.length > 7) strength += 1
// If password contains both lower and uppercase characters, increase strength value.
if (password.match(/([a-z].*[A-Z])|([A-Z].*[a-z])/)) strength += 1
// If it has numbers and characters, increase strength value.
if (password.match(/([a-zA-Z])/) && password.match(/([0-9])/)) strength += 1
// If it has one special character, increase strength value.
if (password.match(/([!,%,&,@,#,$,^,*,?,_,~])/)) strength += 1
// If it has two special characters, increase strength value.
if (password.match(/(.*[!,%,&,@,#,$,^,*,?,_,~].*[!,%,&,@,#,$,^,*,?,_,~])/)) strength += 1
// Calculated strength value, we can return messages
// If value is less than 2
if (strength < 2){
$('#progress').removeClass().addClass('progress-bar').addClass('w-50').addClass('bg-info');
$('#strength').removeClass().addClass('weak');
return 'Weak';
}
else if (strength == 2){
$('#progress').removeClass().addClass('progress-bar').addClass('w-75').addClass('bg-primary');
$('#strength').removeClass().addClass('good');
return 'Good';
}
else{
$('#progress').removeClass().addClass('progress-bar').addClass('w-100').addClass('bg-success');
$('#strength').removeClass().addClass('strong');
return 'Strong';
}
}
Our app's file structure should look like this:
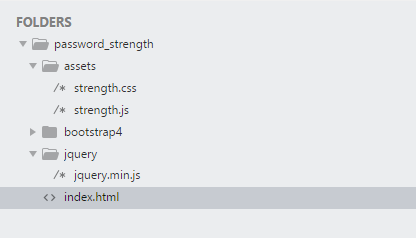
That ends this tutorial. Happy Coding :)