Angular JS Simple Login using Ui-Router
Submitted by nurhodelta_17 on Thursday, January 25, 2018 - 15:51.
Getting Started
I've used CDN for Bootstrap, Angular JS and Ui-Router so you need internet connection for them to work.Creating our Database
First we are going to create our MySQL Database and insert user data that we are going to use in this tutorial. 1. Open phpMyAdmin. 2. Click databases, create a database and name it as angular. 3. After creating a database, click the SQL and paste the below codes. See image below for detailed instruction.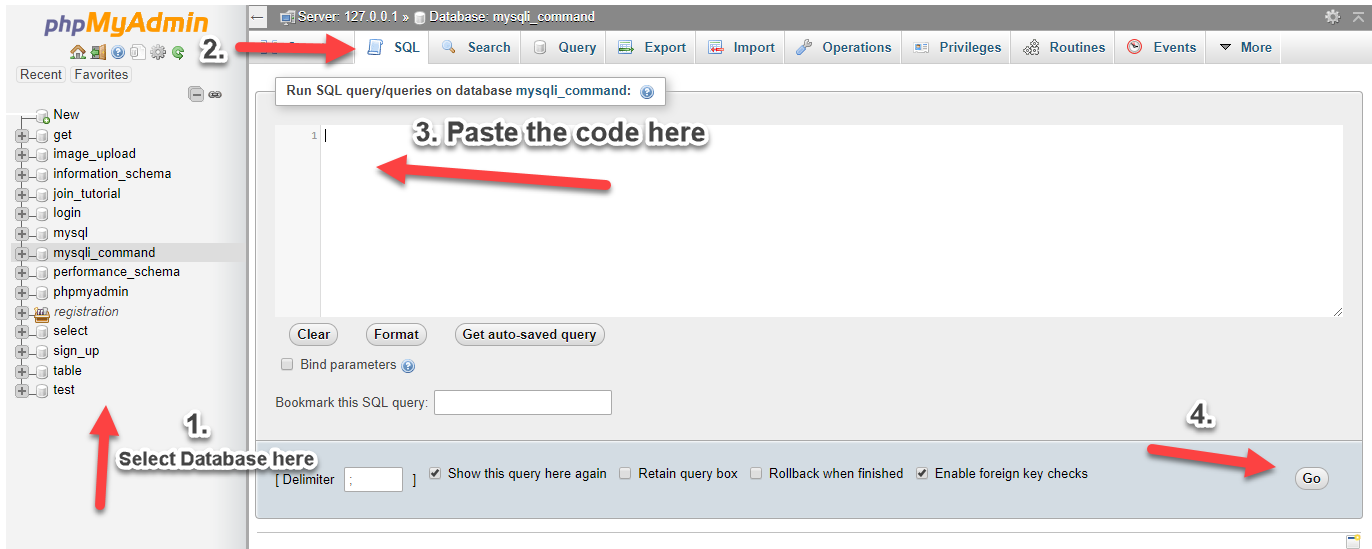
index.html
This is the main view of our app. In here, we have declared all our dependencies and the javascripts that we have created.login.html
This template contains our login form and can be considered as the index view of our app.- <div class="col-md-4 col-md-offset-4">
- <div class="login-panel panel panel-primary">
- <div class="panel-heading">
- </h3>
- </div>
- <div class="panel-body">
- <form name="logform" ng-submit="login()">
- <fieldset>
- <div class="form-group">
- <input class="form-control" placeholder="Username" type="text" autofocus ng-model="user.username" required>
- </div>
- <div class="form-group">
- <input class="form-control" placeholder="Password" type="password" ng-model="user.password" required>
- </div>
- </fieldset>
- </form>
- </div>
- </div>
- <div class="alert alert-danger text-center" ng-show="errorLogin">
- {{ errorMsg }}
- </div>
- </div>
home.html
This is our landing page for a successful login and shows the user that logged into our app.app.js
This is the main angular js script of our app where we assign our app name and the routes of our app.- var app = angular.module('app', ['ui.router']);
- app.config(function($stateProvider, $urlRouterProvider) {
- $urlRouterProvider.otherwise('/login');
- $stateProvider
- .state('login', {
- url: '/login',
- templateUrl: 'login.html',
- controller: 'loginCtrl'
- })
- .state('home', {
- url: '/home',
- templateUrl: 'home.html',
- controller: 'homeCtrl'
- })
- });
loginCtrl.js
This is the controller for our login.html template.- 'use strict';
- app.controller('loginCtrl', function($scope, loginService, $location){
- //redirect back to home if user is logged in
- var connected = loginService.islogged();
- connected.then(function(response){
- if(response.data == 'true'){
- $location.path('/home');
- }
- });
- $scope.errorLogin = false;
- $scope.login = function(){
- loginService.login($scope.user, $scope);
- }
- $scope.clearMsg = function(){
- $scope.errorLogin = false;
- }
- });
homeCtrl.js
This is the controller for our home.html template.- 'use strict';
- app.controller('homeCtrl', function($scope, loginService, $location){
- //redirect user if not loggedin
- var connected = loginService.islogged();
- connected.then(function(response){
- if(response.data == 'false'){
- $location.path('/login');
- }
- });
- //logout
- $scope.logout = function(){
- loginService.logout();
- }
- //fetch login user
- var userrequest = loginService.fetchuser();
- userrequest.then(function(response){
- $scope.user = response.data[0];
- });
- });
loginService.js
This is our service that is responsible for our request relating to login members.- 'use strict';
- app.factory('loginService', function($http, $location, sessionService){
- return{
- login: function(user, $scope){
- var validate = $http.post('login.php', user);
- validate.then(function(response){
- var uid = response.data.user;
- if(uid){
- sessionService.set('user',uid);
- $location.path('/home');
- }
- else{
- $scope.successLogin = false;
- $scope.errorLogin = true;
- $scope.errorMsg = response.data.message;
- }
- });
- },
- logout: function(){
- sessionService.destroy('user');
- $location.path('/login');
- },
- islogged: function(){
- var checkSession = $http.post('session.php');
- return checkSession;
- },
- fetchuser: function(){
- var user = $http.get('fetch.php');
- return user;
- }
- }
- });
sessionService.js
This is our service that is responsible for the sessions in our app both for local storage session and PHP session.- 'use strict';
- app.factory('sessionService', function($http){
- return{
- set: function(key, value){
- return sessionStorage.setItem(key, value);
- },
- get: function(key){
- return sessionStorage.getItem(key);
- },
- destroy: function(key){
- $http.post('logout.php');
- return sessionStorage.removeItem(key);
- }
- };
- });
login.php
This is our PHP api that checks the user that logged into our app.- <?php
- $conn = new mysqli("localhost", "root", "", "angular");
- if ($conn->connect_error) {
- }
- $username = $user->username;
- $password = $user->password;
- $sql = "SELECT * FROM members WHERE username='$username' AND password='$password'";
- $query = $conn->query($sql);
- if($query->num_rows>0){
- $row = $query->fetch_array();
- $out['message'] = 'Login Successful';
- $_SESSION['user'] = $row['memid'];
- }
- else{
- $out['error'] = true;
- $out['message'] = 'Invalid Login';
- }
- ?>
session.php
This is our PHP api that checks for PHP session.- <?php
- echo 'true';
- }
- else{
- echo 'false';
- }
- ?>
fetch.php
This is our PHP api that fetches logged in user details.- <?php
- $conn = new mysqli("localhost", "root", "", "angular");
- $sql = "SELECT * FROM members WHERE memid = '".$_SESSION['user']."'";
- $query=$conn->query($sql);
- while($row=$query->fetch_array()){
- $output[] = $row;
- }
- ?>
logout.php
Lastly, this is our PHP api that destroys our current user PHP session.- <?php
- ?>