Instagram Status Post
Submitted by rinvizle on Thursday, July 14, 2016 - 14:55.
In this tutorial we will create a instagram status script post in PHP and Javascript. This project creates a post message that you can comment or reply in every statuses that the user posted. And their are features that you will like delete post or you can comment to the other people post and it is real time process of the data so the user will know what day and what time did the user posted or created the status. This project is related to all SNS system that creates and shows the data to other user of that system. I will show you the sample code and images below.
And for the Login Script
Sample Image
For more tutorials just visit this website @www.sourcecodester.com and don't forget to Like and Share. Enjoy Coding.
Sample Code
Database PHP Script- <?php
- require_once(LIB_PATH.DS."config.php");
- class Database {
- var $data;
- var $sql_string = '';
- var $error_no = 0;
- var $error_msg = '';
- private $conn;
- public $last_query;
- private $magic_quotes_active;
- private $real_escape_string_exists;
- function __construct() {
- $this->open_connection();
- }
- public function open_connection() {
- if(!$this->conn){
- echo "Problem in database connection! Contact administrator!";
- }else{
- if (!$db_select) {
- echo "Problem in selecting database! Contact administrator!";
- }
- }
- }
- function setQuery($sql='') {
- $this->sql_string=$sql;
- }
- function executeQuery() {
- $this->confirm_query($result);
- return $result;
- }
- private function confirm_query($result) {
- if(!$result){
- return false;
- }
- return $result;
- }
- function loadResultList( $key='' ) {
- $cur = $this->executeQuery();
- if ($key) {
- $array[$row->$key] = $row;
- } else {
- $array[] = $row;
- }
- }
- return $array;
- }
- function loadSingleResult() {
- $cur = $this->executeQuery();
- return $data = $row;
- }
- }
- function getFieldsOnOneTable( $tbl_name ) {
- $this->setQuery("DESC ".$tbl_name);
- $rows = $this->loadResultList();
- $f[] = $rows[$x]->Field;
- }
- return $f;
- }
- public function fetch_array($result) {
- }
- public function num_rows($result_set) {
- }
- public function insert_id() {
- }
- public function affected_rows() {
- }
- public function escape_value( $value ) {
- if( $this->real_escape_string_exists ) {
- } else {
- }
- return $value;
- }
- public function close_connection() {
- }
- }
- }
- $mydb = new Database();
- ?>
- <?php
- if($email == ''){
- echo 'Username or Password Not Registered! Contact Your administrator...';
- }elseif($upass == ''){
- echo 'Username or Password Not Registered! Contact Your administrator...';
- }else{
- $sql = "SELECT * FROM `user_info` WHERE `email`='". $email ."' and `pword`='". $h_upass ."'";
- if ($numrows == 1){
- $_SESSION['member_id'] = $found_user['member_id'];
- $_SESSION['fName'] = $found_user['fName'];
- $_SESSION['lName'] = $found_user['lName'];
- $_SESSION['email'] = $found_user['email'];
- $_SESSION['pword'] = $found_user['pword'];
- $_SESSION['gender'] = $found_user['gender'];
- ?> <script type="text/javascript">
- window.location = "home.php";
- </script>
- <?php
- }else{
- ?> <script type="text/javascript">
- alert("Username or Password Not Registered! Contact Your administrator.");
- window.location = "home.php";
- </script>
- <?php
- }
- }
- }else{
- $email = "";
- $upass = "";
- $utype = "";
- }
- ?>
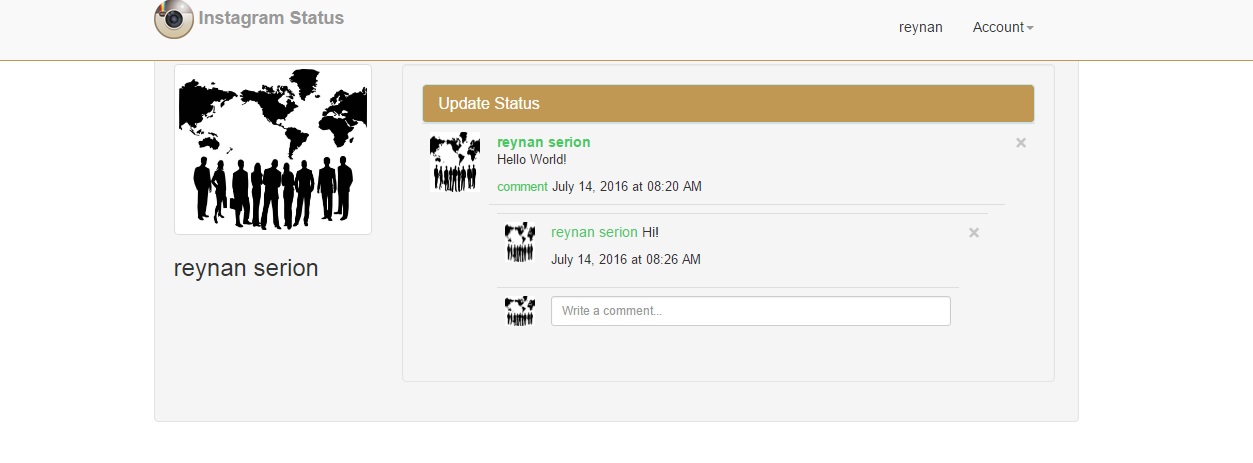