JSpinner Component in Java
Submitted by donbermoy on Friday, November 14, 2014 - 08:05.
This is a tutorial in which we will going to create a program that will have a JSpinner Component in Java.
A JSpinner Component is similar to a JComboBox and JList in java that it has object options to choose but this component has only a single line input field that lets the user select an object value from an ordered sequence.
It will have the SpinnerModel to have the JSpinner's sequence.
So, now let's start this tutorial!
1. Open JCreator or NetBeans and make a java program with a file name of jSpinnerComponent.java.
2. Import the following packages:
3. Initialize your variable in your Main, variable months for the String array, variable spinModel for SpinnerModel class, variable spinner for JSpinner component, and variable frame for the JFrame class.
4. Lastly, set the size and visibility of the Frame.
Output:
Here's the full code of this tutorial:
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
If you have some queries, feel free to contact the number or e-mail below.
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
- import java.awt.*; //used to access the flowlayout
- import javax.swing.*; // used to access the JSpinner, SpinnerListModel, SpinnerModel, and the JFrame Class
We have created a String array of months to display these elements in the spinner. But before putting the array value to the JSpinner, we will put it first to the SpinnerModel class and then put the spinModel variable of this class to the JSpinner.
Then we have the JFrame component that will serve as the container of the objects and has the flowlayout as the layout used as we have this code below:
- frame.getContentPane().add(spinner);
- frame.setSize(300, 100);
- frame.setVisible(true);
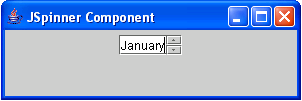
- import java.awt.*; //used to access the flowlayout
- import javax.swing.*; // used to access the JSpinner, SpinnerListModel, SpinnerModel, and the JFrame Class
- public class jSpinnerComponent {
- String months[] = {"January","February","March","April","May","June","July","August","September","October","November","December"};
- SpinnerModel spinModel = new SpinnerListModel(months);
- JSpinner spinner = new JSpinner(spinModel);
- frame.getContentPane().add(spinner);
- frame.setSize(300, 100);
- frame.setVisible(true);
- }
- }