How to get the extension of a file using Java
Submitted by donbermoy on Monday, May 26, 2014 - 23:23.
Today, I will teach you how to create a program that get the extension of a file using java. For example if we input a text file of .txt file extension then the output will be .txt.
So, now let's start this tutorial!
1. Open JCreator or NetBeans and make a java program with a file name of extensionFile.java.
2. Import java.io package. Hence we will use an input/output in creating files.
3. Also in your program, create a variable for the separator as it will separate the extension file of dot(.). Make this as a public static String variable.
4. To get the extension of a file we will create a method named getFileExtension with a File paramater as String.
If the file inputted is empty, then it will return as null.
Separate the file name and the file extension.
5. In your main, instantiate the File class having the file variable. For example you want to have a file name of data.docx, READ FIRST.txt, extensionFile.java, and extensionFile.class. Note: you can change the file name of this file.
To get the extension of the file, i simply use the getFileExtension method that we have created earlier with the extensionFile class and its inputed file. Then, we displayed the output using System.out.println().
Output:
Hope this helps! :)
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
If you have some queries, feel free to contact the number or e-mail below.
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
- import java.io.*;
- int extIndex = extName.lastIndexOf(extensionFile.separator);
- if (extIndex == -1) {
- return "";
- } else {
- return extName.substring(extIndex + 1);
- }
- extension = extensionFile.getFileExtension(file);
- extension = extensionFile.getFileExtension(file);
- extension = extensionFile.getFileExtension(file);
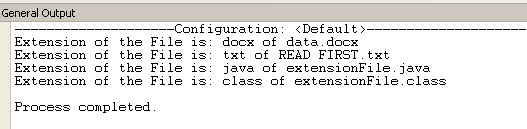