How to Display the Current Row Into the TextBoxes Using C#
Submitted by janobe on Saturday, October 5, 2019 - 06:18.
Passing data from the datagridview into the textbox is a common problem if you are a beginner in programming. So, in this tutorial, I will teach you how to display the current row into the textboxes using c#. This program is composed by adding column and rows in the datagridview programmatically and it will automatically display the data in the textbox when the datagridview cell is clicked. This is a big help for you to solve your current problem in programming and you can do it in no time. Follow the step by step guide to know how it works.
The complete source code is included. You can download it and run it on your computer.
For any questions about this article. You can contact me @
Email – [email protected]
Mobile No. – 09305235027 – TNT
Or feel free to comment below.
Creating Application
Step 1
Open Microsoft Visual Studio 2015 and create a new windows form application for c#.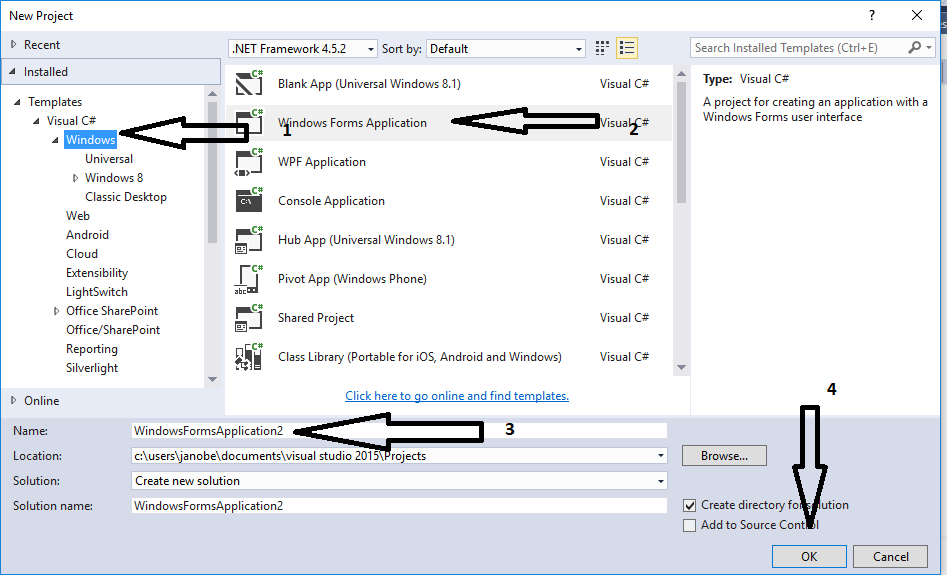
Step 2
Do the form just like shown below.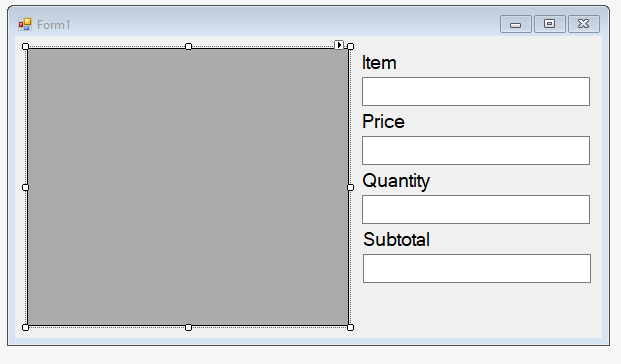
Step 3
Double click the form and to the following codes for adding columns and rows automatically in the first load of the form.- private void Form1_Load(object sender, EventArgs e)
- {
- //setup the columns to be added.
- dataGridView1.ColumnCount = 4;
- //Set the columns name
- dataGridView1.Columns[0].Name = "Item";
- dataGridView1.Columns[1].Name = "Price";
- dataGridView1.Columns[2].Name = "Quantity";
- dataGridView1.Columns[3].Name = "Sub-Total";
- //Set a value to be added in a row
- //adding rows in the datagridview
- dataGridView1.Rows.Add(row);
- //Set a value to be added in a row
- //adding rows in the datagridview
- dataGridView1.Rows.Add(row);
- //Set a value to be added in a row
- //adding rows in the datagridview
- dataGridView1.Rows.Add(row);
- //Set a value to be added in a row
- //adding rows in the datagridview
- dataGridView1.Rows.Add(row);
- }
Step 4
Write the following code for passing the data from the datagridview to the textboxes when the current row is selected.- private void dataGridView1_CellClick(object sender, DataGridViewCellEventArgs e)
- {
- dtg = dataGridView1;
- txtProduct.Text = dtg.CurrentRow.Cells[0].Value.ToString();
- txtPrice.Text = dtg.CurrentRow.Cells[1].Value.ToString();
- txtQauntity.Text = dtg.CurrentRow.Cells[2].Value.ToString();
- txtSubtotal.Text = dtg.CurrentRow.Cells[3].Value.ToString();
- }