Fill Data Based on DataGridViewComboBoxColumn in C# and MySQL Database
Submitted by janobe on Monday, March 25, 2019 - 18:58.
In this tutorial, I will teach you how to fill the data in the DataGridViewComboBoxColumn using C# and MySQL database. This method has the ability to add a combobox column in the datagridview. It also has the capacity to fill the combobox with data from the database. This method is very useful when you are dealing with datagridview control.
The complete source code is included you can download it and run it on your computer.
For any questions about this article. You can contact me @
Email – [email protected]
Mobile No. – 09305235027 – TNT
Or feel free to comment below.
Creating Application
Step 1
Open Microsoft Visual Studio 2015 and create a new windows form application for c#.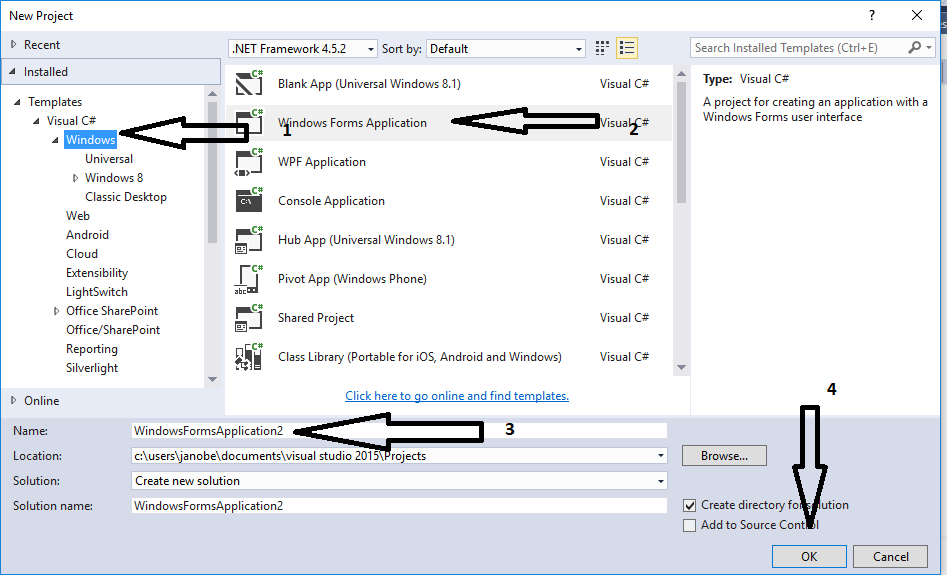
Step 2
Do the form just like shown below.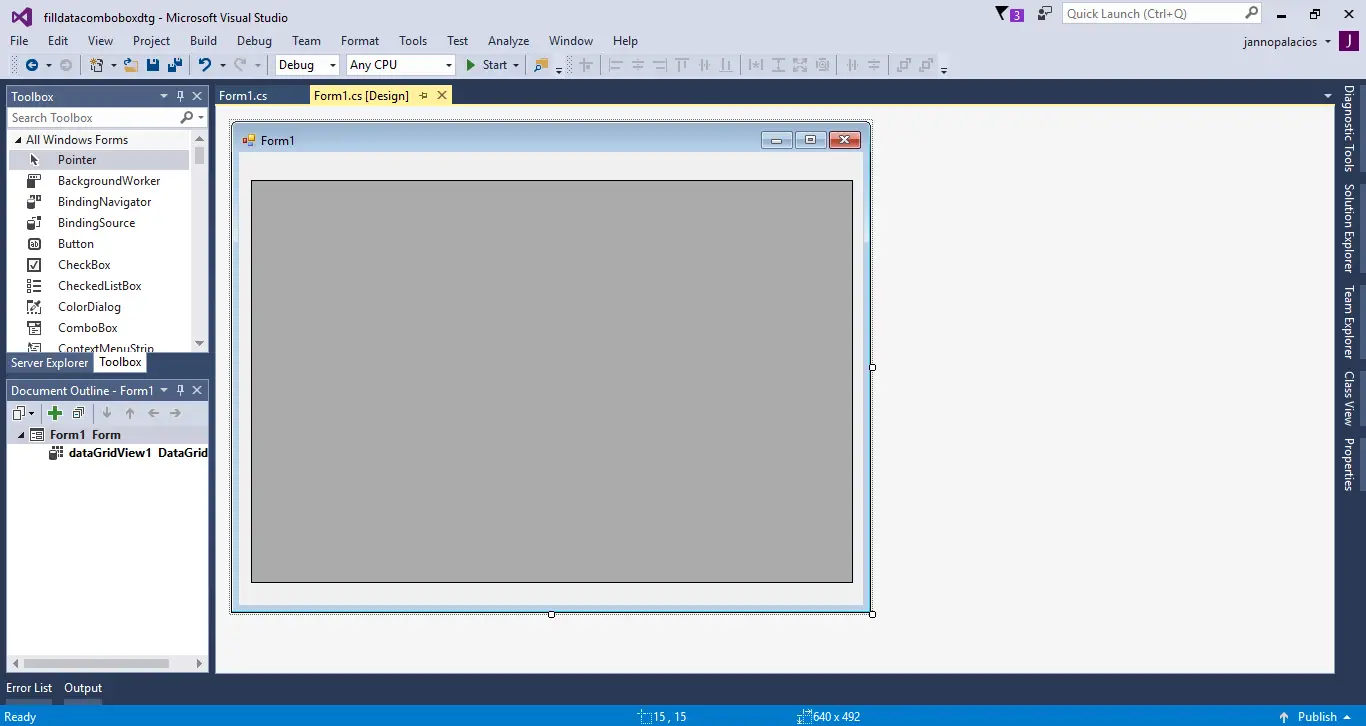
Step 3
Press F7 to open the code editor. In the code editor, add a namespace to accessMySQL
libraries
- using MySql.Data.MySqlClient;
Step 4
Establish a connection between C# and MySQL database. After that, declare all the classes and variables that are needed.Step 5
Create a method for adding and filling data in the combobox.- private void fill_combo(string sql)
- {
- try
- {
- con.Open();
- cmd.Connection = con;
- cmd.CommandText = sql;
- da.SelectCommand = cmd;
- da.Fill(dt);
- foreach (DataRow dr in dt.Rows)
- {
- cbo.Items.Add(dr.Field<string>("SUBJ_CODE"));
- cbo.Name = "Subject Code";
- }
- //add the combobox in the column in the datagridview
- dataGridView1.Columns.Add(cbo);
- }
- catch(Exception ex)
- {
- MessageBox.Show(ex.ToString());
- }
- finally
- {
- con.Close();
- da.Dispose();
- }
- }
Step 6
Writ the following codes to execute the method in the first load of the form.- private void Form1_Load(object sender, EventArgs e)
- {
- sql = "SELECT * FROM subject";
- fill_combo(sql);
- }
Add new comment
- 956 views