How to Search Data Between Two Dates Using C#
Submitted by janobe on Wednesday, November 21, 2018 - 19:14.
This time, I will teach you how to search data between two dates Using C#. This method has the ability to find all the records in the database between two inclusive dates. In this way, you can filter the data to be displayed in the datagridview. Hope this tutorial will help you to solve your problem in filtering data between two dates. See the procedure below.
Note:
First, add MySQL.Data.dll for the references.
Second, add this
Creating Database
Create a database named “db_payroll”. Write this query to create a table and add the data in the table.- --
- -- Dumping data for table `tblpayroll`
- --
- (1, 'Janobe Palacios', 'IT Department', 12000, '2018-10-31'),
- (2, 'Jeanniebe Nillos', 'Accounting Department', 10000, '2018-10-31'),
- (3, 'Janobe Palacios', 'IT Department', 15000, '2018-11-30'),
- (4, 'Jeanniebe Nillos', 'Accounting Department', 12000, '2018-11-30'),
- (5, 'Janobe Palacios', 'IT Department', 9000, '2018-09-30'),
- (6, 'Jeanniebe Nillos', 'Accounting Department', 8000, '2018-09-30');
Creating Application
Step 1
Open Microsoft Visual Studio 2015 and create a new windows form application for c#.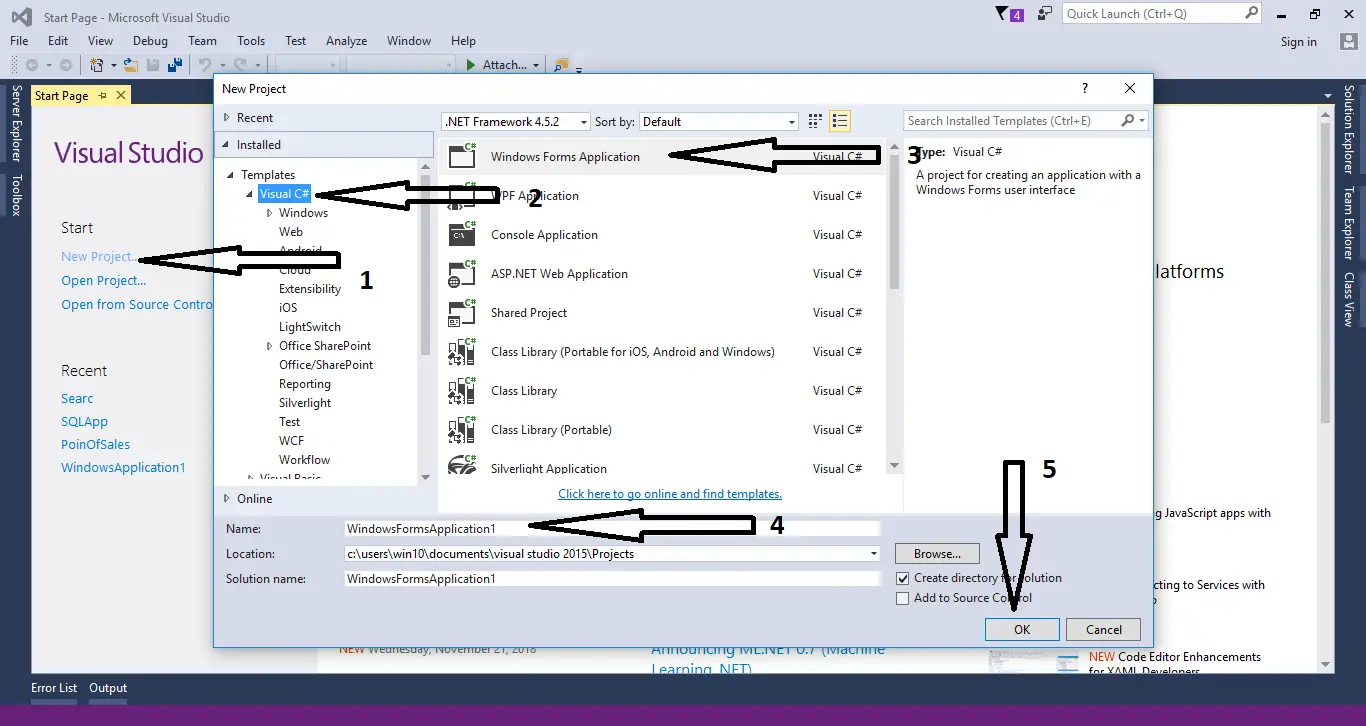
Step 2
Do the Form just like show below.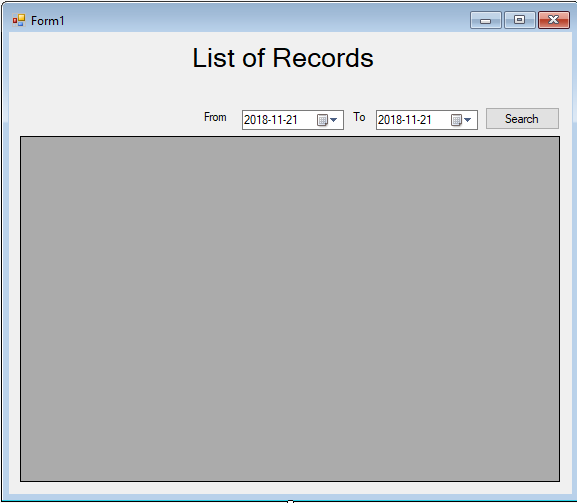
Step 3
Open the code editor and create a connection between c# and mysql database. After that, initialize all the classes that are needed.Step 4
Create a method for retrieving data in the database.- private void loadData(string sql,DataGridView dtg)
- {
- try
- {
- con.Open();
- cmd.Connection = con;
- cmd.CommandText = sql;
- da.SelectCommand = cmd;
- da.Fill(dt);
- dtg.DataSource = dt;
- }
- catch (Exception ex)
- {
- MessageBox.Show(ex.Message);
- }
- finally
- {
- con.Close();
- da.Dispose();
- }
- }
Step 5
Double click the form and call the method that you have created to display the data into the datagridview in the first load of the form.- string sql;
- sql = "SELECT * FROM tblpayroll";
- loadData(sql, dataGridView1);
Step 6
Double click the button and do the following codes for searching data between two dates.- string sql;
- DateTime from = dateTimePicker1.Value;
- DateTime to = dateTimePicker2.Value;
- sql = "SELECT * FROM tblpayroll WHERE DATE(PayrollDate) BETWEEN '" + from.ToString("yyyy-MM-dd") + "' AND '" + to.ToString("yyyy-MM-dd") + "'";
- loadData(sql, dataGridView1);
using MySql.Data.MySqlClient;
above the namespace to access MySQL library.
The complete source code is included. You can download it and run it on your computer.
For more question about this article. You can contact me @
Email – [email protected]
Mobile No. – 09305235027 – TNT
FB Account – https://www.facebook.com/onnaj.soicalap
Or feel free to comment below.