Auto Search Word Generator
Submitted by rinvizle on Friday, July 15, 2016 - 16:14.
In this tutorial we will create a game in C# called Auto Search Word Generator. This game creates a way of searching the word in the display letters in the form. And this game has a features to add, remove, generate, and show the answer of the list of words given or added by the user. Each word will be automatically generate and mix in the random letters and you will find out if what word will you want it to search and it will automatically show the exact word that you give. I will show you the sample code and images below.
And for the Random Code
If you want to run this game in .exe application you must build and debug it again using Visual Studio. Don't forget to Like and Share. Enjoy Coding.
Sample Code
Generating Script- function generate(Words)
- {
- gridlength := maxlength(listofwords);
- while (successful)
- {
- notfound := false;
- foreach (word in list)
- {
- word->Location := Calculate(wordsplaced, word);
- if (Location == null)
- {
- notfound := true;
- break;
- }
- if (!notfound)
- {
- return FormGrid(words, locations);
- }
- else
- {
- gridlength++;
- }
- }
- }
- }
- private Letter[] Next(Letter[][] letters, string word, int length)
- {
- List<Point> all = new List<Point>(AllPoints(length));
- while (all.Count > 0)
- {
- List<Direction> dirs = new List<Direction>(AllDirections());
- while (dirs.Count > 0)
- {
- Direction current = dirs[index2];
- Letter[] c = Construct(all[index], current, word, length);
- bool legal = true;
- for (int i = 0; i < c.Length; i++)
- {
- if (c[i].X < 0 || c[i].X >= length || c[i].Y < 0 || c[i].Y >= length)
- {
- legal = false;
- break;
- }
- else
- {
- for (int j = 0; j < letters.Length; j++)
- {
- Point[] pts = letters[j].Select<Letter, Point>(t => new Point(t.X, t.Y)).ToArray();
- int _index2 = pts.ToList().FindIndex(t => t.X == c[i].X && t.Y == c[i].Y);
- if (_index2 != -1)
- if (c[i]._Letter != letters[j][_index2]._Letter)
- legal = false;
- }
- }
- }
- if (legal)
- return c;
- dirs.RemoveAt(index2);
- }
- all.RemoveAt(index);
- }
- return null;
- }
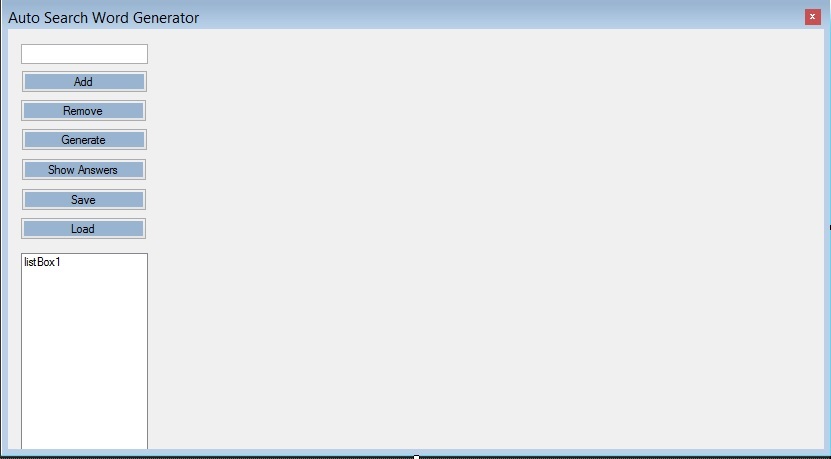