Drag/Drop and Copy Control in Visual Basic 2008
Submitted by janobe on Friday, April 25, 2014 - 20:08.
In this tutorial I will teach you how to create Drag/Drop and Copy Control using Visual Basic 2008. With this, you can drag and drop the picture that’s inside the Picture box and you can also copy it to another Picture Box by pressing the Ctrl key control.
Let's begin:
Open Visual Basic 2008, create a new Windows Application and drag the two PictureBoxes in the Form. Then, name the first PictureBox “leftpic” and the other one is “rightpic”.
Double click the Form, and do the following code for declaring a constant variable above the
In the
Go back to the Design Views, click the two PictureBoxes and go to the properties. After that, click the events on the top that looks like a lightning. Choose the
In the Method Name, choose the
Go back to the Method Name again,choose
Note: Put an image in one of the two PictureBoxes.
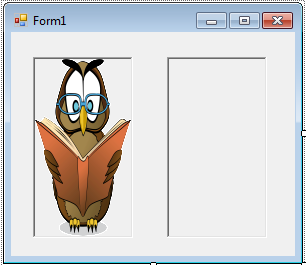
Form_Load
.
- ' DECLARE A CONSTANT VARIABLE
- ' IT IS USED FOR DETECTING THE CTRL KEY WHETHER IT
- ' WAS PRESSED OR NOT DURING THE DRAG OPERATION
- Const maskCtrl As Byte = 8
Form_Load
do this following code for activating the AllowDrop property.
- Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
- 'Set the AllowDrop property to the PictureBox
- leftpic.AllowDrop = True
- rightpic.AllowDrop = True
- End Sub
MouseDown
event, then double click it and do this following code.
- Private Sub PictureBox_MouseDown(ByVal sender As System.Object, ByVal e As System.Windows.Forms.MouseEventArgs) Handles leftpic.MouseDown, rightpic.MouseDown
- If e.Button = Windows.Forms.MouseButtons.Left Then
- Dim pictures As PictureBox = CType(sender, PictureBox)
- 'ACTIVATES THE DRAG AND DROP OPERATION
- If Not pictures.Image Is Nothing Then
- pictures.DoDragDrop(pictures.Image, DragDropEffects.Move Or DragDropEffects.Copy)
- End If
- End If
- End Sub
DragEnter
for the events and do this following code.
- Private Sub PictureBox_DragEnter(ByVal sender As System.Object, ByVal e As System.Windows.Forms.DragEventArgs) Handles leftpic.DragEnter, rightpic.DragEnter
- 'CONDITIONING THE DRAG CONTENT TO MAKE SURE THAT THE TYPE IS ACCURATE FOR THIS CONTROL
- If (e.Data.GetDataPresent(DataFormats.Bitmap)) Then
- If (e.KeyState And maskCtrl) = maskCtrl Then 'CHECKING THE CTRL KEY WAS PRESSED.
- 'RESULT TRUE
- e.Effect = DragDropEffects.Copy 'PERFORM A COPY.
- Else
- 'RESULT FALSE
- e.Effect = DragDropEffects.Move 'PERFORM A MOVE
- End If
- Else
- 'REJECT THE DROP
- e.Effect = DragDropEffects.None
- End If
- End Sub
DragDrop
for the events and do this following code.
- Private Sub PictureBox_DragDrop(ByVal sender As System.Object, ByVal e As System.Windows.Forms.DragEventArgs) Handles leftpic.DragDrop, rightpic.DragDrop
- 'IMAGE WILL DISPLAY TO THE SELECTED PICTUREBOX CONTROL.
- Dim pictures As PictureBox = CType(sender, PictureBox)
- pictures.Image = CType(e.Data.GetData(DataFormats.Bitmap), Bitmap)
- 'IF THE PICTURE WAS MOVED IN THE PICTUREBOX THE OTHER BOX BECOMES EMPTY.
- If (e.KeyState And maskCtrl) <> maskCtrl Then 'CHECKING THE CTRL KEY WAS NOT PRESSED.
- If sender Is leftpic Then
- rightpic.Image = Nothing
- Else
- leftpic.Image = Nothing
- End If
- End If
- End Sub
Add new comment
- 56 views