How to Dynamically Add new Textbox using jQuery in JavaScript
How to Dynamically Add new Textbox using jQuery in JavaScript
Introduction
In this tutorial we will create a How to Dynamically Add new Textbox using jQuery in JavaScript. This tutorial purpose is to teach you how to add new textboxes dynamically. This will thoroughly make use basic functionality of jQuery . I will provide a sample program to show the actual coding of this tutorial.
This tutorial is simple and easy to understand just follow the instruction I provided and you can do it without a problem. This program can be use to any system or application that uses jQuery plugin to enhance the interact content of your website. I will give my best to provide you the easiest way of creating this program Dynamically add new textbox. So let's do the coding.
Before we get started:
First you have to download the jQuery plugin.
Here is the link for the jQuery that i used in this tutorial https://jquery.com/.
Lastly, this is the link for the template that i used for the layout design https://getbootstrap.com/.
Creating The Interface
This is where we will create a simple interface for our application. This code will only display the html form and button. To create this simply copy and write it into your text editor, then save it as index.html.- <!DOCTYPE html>
- <html lang="en">
- <head>
- <meta charset="UTF-8" name="viewport" content="width=device-width, initial-scale=1"/>
- <link rel="stylesheet" type="text/css" href="css/bootstrap.css"/>
- </head>
- <body>
- <nav class="navbar navbar-default">
- <div class="container-fluid">
- </div>
- </nav>
- <div class="col-md-6 well">
- <hr style="border-top:1px dotted;"/>
- <div class="col-md-4">
- <form>
- <div class="form-group">
- <input type="text" id="input" class="form-control"/>
- </div>
- </form>
- </div>
- </div>
- </body>
- </html>
Creating JavaScript Function
This is where the main function of the application is. This code will add new textbox dynamically when the buttons is clicked. To do this just copy and write these block of codes inside the text editor and save it as script.js.- $(document).ready(function(){
- $('#add').on('click',function(){
- var input = $('#input').val();
- if(input != ""){
- $('#submit').show();
- var newInput = $(
- '<div class="form-group">'
- +'<input type="text" placeholder="'+input+'" class="form-control newInput" required="required"/>'
- +'</div>'
- );
- newInput.appendTo('#input_container');
- $('#input').val('');
- }else{
- alert("Please enter something");
- }
- });
- $('#submit').on('click', function(){
- var list=[];
- $('.newInput').each(function(){
- list.push($(this).val());
- });
- alert(list.join(', '));
- });
- });
In the given code we first call the basic jQuery ready event to signal that the DOM of the page is now ready to be use. In this code we just simply bind the button to trigger the event for adding new textbox. The function includes some variables and a certain function that will add new textbox using the append function of jQuery.
Output:
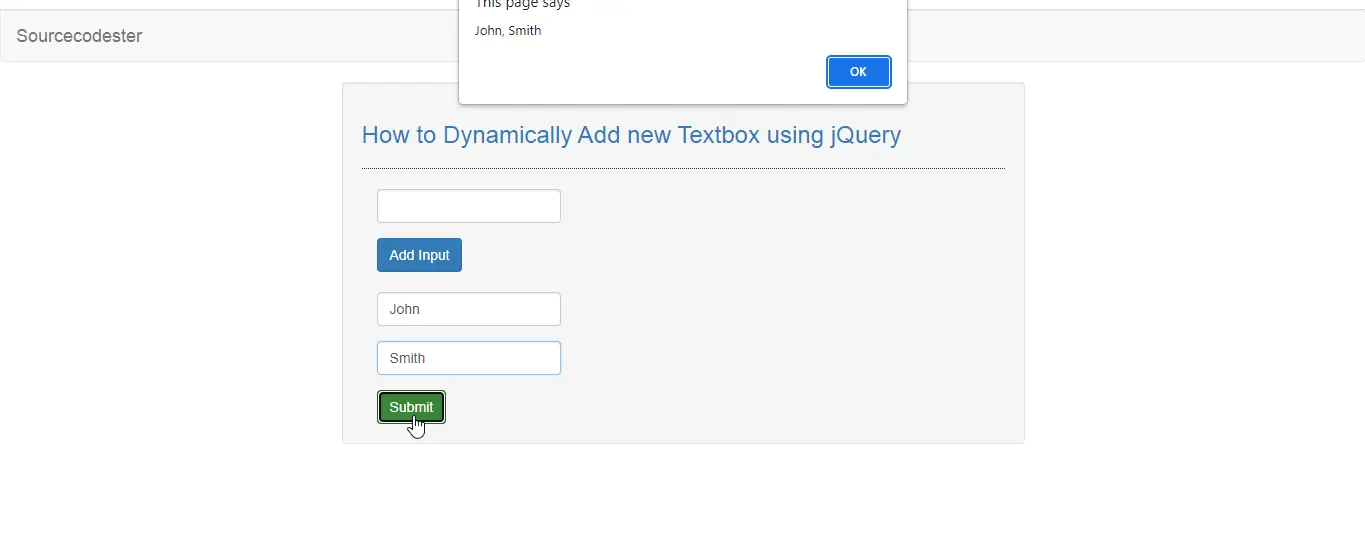
The How to Dynamically Add new Textbox using jQuery in JavaScript source code that I provide can be download below. Please kindly click the download button.
There you have it we successfully created How to Dynamically Add new Textbox using jQuery in JavaScript. I hope that this simple tutorial help you to what you are looking for. For more updates and tutorials just kindly visit this site. Enjoy Coding!
More Tutorials for JavaScript Language