How to Upload multiple files into Database in PHP/MySQLi
Submitted by nurhodelta_17 on Tuesday, January 19, 2021 - 13:19.
In this tutorial. I'm going to show you how to upload multiple files using PHP/MySQLi.
Creating our Database
First step is to create our database.- Open phpMyAdmin.
- Click databases, create a database and name it as "upload".
- After creating a database, click the SQL and paste the below code. See image below for detailed instruction.
- CREATE TABLE `photo` (
- `photoid` INT(11) NOT NULL AUTO_INCREMENT,
- `location` VARCHAR(150) NOT NULL,
- PRIMARY KEY(`photoid`)
- ) ENGINE=InnoDB DEFAULT CHARSET=latin1;
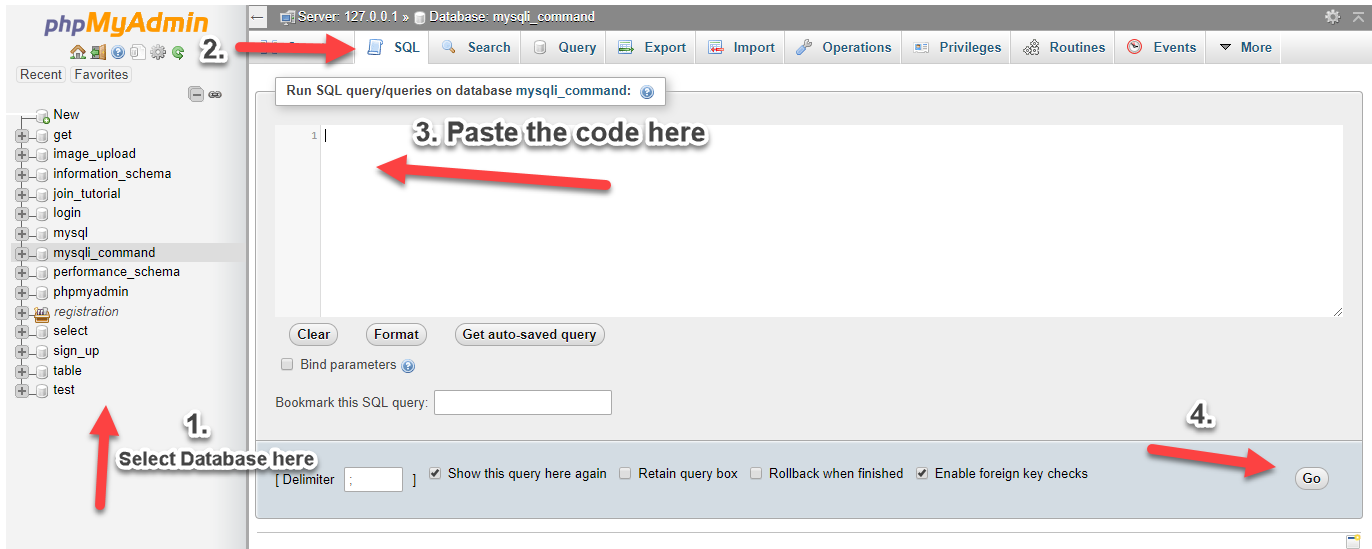
Creating our Connection
Next, we create our connection to our database. This will serve as the bridge between our forms and database. We name this as conn.php.
- <?php
- //MySQLi Procedural
- if (!$conn) {
- }
- ?>
Creating our Output Folder
Next step is to create our output folder. This will serve as storage of uploaded files. We name the folder upload.
index.php
We create our Upload Form and show the files that we have uploaded. In the case of this tutorial, I've shown files uploaded as images.
- <!DOCTYPE html>
- <html>
- <head>
- <title>Uploading Multiple Files using PHP</title>
- </head>
- <body>
- <div style="height:50px;"></div>
- <div style="margin:auto; padding:auto; width:80%;">
- <span style="font-size:25px; color:blue"><center><strong>Uploading Multiple Files into MySQL Database using PHP/MySQLi</strong></center></span>
- <hr>
- <div style="height:20px;"></div>
- <form method="POST" action="upload.php" enctype="multipart/form-data">
- <input type="file" name="upload[]" multiple>
- <input type="submit" value="Upload">
- </form>
- </div>
- <div style="margin:auto; padding:auto; width:80%;">
- <h2>Output:</h2>
- <?php
- include('conn.php');
- ?>
- <img src="<?php echo $row['location']; ?>" height="150px;" width="150px;">
- <?php
- }
- ?>
- </div>
- </body>
- </html>
upload.php
Lastly, we create the code in uploading multiple files to our database.
- <?php
- include('conn.php');
- foreach ($_FILES['upload']['name'] as $key => $name){
- $location = 'upload/' . $newFilename;
- }
- ?>
And that ends this tutorial. If you have any comments or questions, feel free to comment below or message me here at sourcecodester. Hope this helps.
Happy Coding :)