Overriding function
In this part you will learn:
1. What are overriding functions?
2. What are the benefits of using overriding functions?
3. How to override a function
4. Program
5. Output
Overriding function
A function of base class defined again in the derived class is known as overriding function. In many c++ programs, there comes a case when we want to add extra functionality in the base class. So we override the function. In function overriding if the object is of base class then the member function of base class is called, if the object is of derived class then the member function (overriding function) is called.
How to override a function
There are some rules that must be followed while overriding a function. Overriding of function can only happen when there are two or more classes and they have inheritance between them. The most important thing to keep in mind while overriding a function is that the declaration of the function which we are going to make overriding function should have same return type, same name and same parameters as of the function in the base class. Even a little difference will make it a separate function of derived class.
Syntax
Following is the syntax of defining a member function outside the class.
class Class_Name1
{
//other members
return_type Function_name(parameters); //function we want to override in derived class
}
class Class_name2:type_of_inheritance Class_Name1
{
//other members
return_type Function_name(parameters); //overriding function
}
In the above syntax the most important thing to note is that in the derived class, the function will have the same return type, same function name and same parameters as of in the base class.
Program with overriding function
I have explained the usage of overriding function by the example of a bakery cake order system, I have divided the cakes in two types cake and special cake, where cake is the base class of special cake. The special cake has a member variable named “note” which stores the customer demand of writing a note on the cake.
The overriding function is cake_info. Following is the program for this.
Basic Step:
#include<iostream>
#include<conio.h>
#include<string.h>
using namespace std;
These two are the most common and basic lines of a c++ program. The first one iostream is used to grant us most of the basic functions of C++ like Input and Output functions. The second one conio.h means console input output, the black screen we see is the console. Using namespace std; provides us an ease of using all the library functions without specifying that this function belongs to standard library. Without including it, we have to write ‘std’ with all the built-in functions.
I have added another library file known as string.h, since we are using string(array of characters) so we have included the library of it before using it in the example given below.
Classes
class cake
{
public: //access specifier
int size;
string flavour;
string type;
cake(int s, string f, string t) :size(s), flavour(f), type(t){
}
void cake_info(){
cout << "It is a " << type << " cake of " << flavour << " flavour. It is of " << size << " pound(s)";
cout << endl;
}
};
class special_cake:private cake
{
public: //access specifier
string note;
special_cake(int s, string f, string t, string n) :cake(s, f, t), note(n){
}
void cake_info(){
cout << "It is a " << type << " cake of " << flavour << " flavour. It is of " << size << " pound(s)";
cout << endl;
cout << "with a special note \"" << note << "\" on it";
}
};
The thing to focus on the most is the overriding function. In the derived class I have defined that function with the same declaration as of in the base class, i.e. the return type is void in both, the function name is cake_info in both and both of them have no parameters passing in them. So now we have a overriding function of cake_info which prints on the screen the note in case of object of special_cake and the just the other information if the object is of class cake.
Let us take a look at the main function of this.
Main
int main()
{
cake c1(2, "chocolate", "icecream");
special_cake c2(1, "vanilla", "cream", "love you mom");
c1.cake_info();
cout << endl;
c2.cake_info();
cout << endl;
}
Output
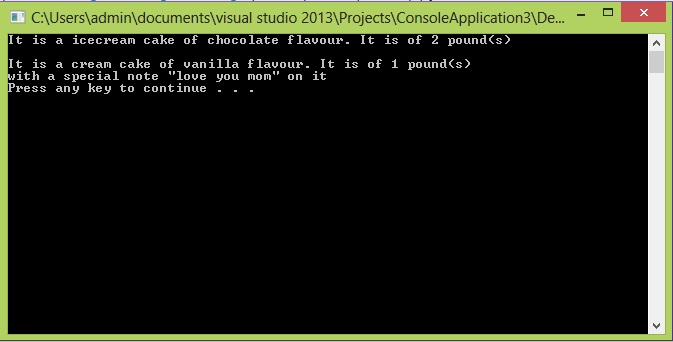