Operator overloading
In this part you will learn:
1. What is meant by operator overloading
2. Why we need to overload operators
3. Syntax
4. Program
5. Output
Operator Overloading
As the name suggests, operator overloading means to make overloaded function for an operator of a class. There are many operators in programming the basic ones are given below
• logical operators(,=,==,!=)
• Arithmetic operators(+,-,*,/,%)
• unary operators (++,--)
• assignment operator (=) etc.
We can even overload console input and output operators. In case of classes, the built-in operators are not useable directly on the objects, so we have to overload operators for our classes to use them on the objects made by that class.
In general we make the console input, output overloaded operators as friend function. The reason behind this is that the console input, output functions don’t have any caller object. So they are made as a friend function of a class. Moreover other operators like assignment, arithmetic etc would be made directly, this is because they are binary operators, the left object will be considered as the caller object of the overloaded operator.
As an example I will discuss a console input overloaded operator function and some other operator function which won’t be using a friend function.
Syntax
Following is the syntax of overloading an operator of a class.
return_type Class_Name_to_which_Function_belongs :: operator Operator_symbol(+,-,= etc) (parameters)
{
//overloaded operator function definition
}
return_type will decide what the operator will return when the operator will be called. Then we will access class using its name and scope resolution operator. After that we will write the keyword “operator” and then write the symbol of the operator which we are overloading and then the parameter.
The overloading operator will further be more clear in the examples given below.
Program with operator overloading
Consider the following example of class named “fraction”. As from the name, this class stores numerator and denominator of a fraction in it. Suppose we want to input a fraction from the user. What we can do is use cout in the main asking the user to enter a fraction and then use setter function to assign the values to the object. Won’t it make our program tidy? The smart way is to overload the operator Basic Step:
#include<iostream>
#include<conio.h>
using namespace std;
These two are the most common and basic lines of a c++ program. The first one iostream is used to grant us most of the basic functions of C++ like Input and Output functions. The second one conio.h means console input output, the black screen we see is the console. Using namespace std; provides us an ease of using all the library functions without specifying that this function belongs to standard library. Without including it, we have to write ‘std’ with all the built-in functions.
Class
class fraction
{
private: //access specifier
int num;
int denom;
public:
void getfraction();
fraction operator + (fraction f);
friend istream& operator >> (istream &input, fraction &f);
};
void fraction::getfraction()
{
cout << "Fraction is: " << num << "/" << denom << endl;
}
istream& operator >> (istream &input, fraction &f)
{
cout << "Enter the numerator: ";
input >> f.num;
cout << "Enter the denominator: ";
input >> f.denom;
return input;
}
fraction fraction :: operator + (fraction f)
{
fraction sum;
sum.num = (this->num*f.denom) + (this->denom*f.num);
sum.denom = this->denom*f.denom;
return sum;
}
In the above definition of class and its member functions, I have overloaded the console input operator and + operator. The rest of the code is just normal. First in input operator overloading, I have defined the operator outside and declared it as a friend of class “fraction”. The reason behind this is that we know that the input operator doesn’t have any caller, so it must be defined separately. In the function prototype “istream& operator >> (istream &input, fraction &f)”, we are returning istream as a reference this is because a return by reference can be placed on the left side. Moreover it is for the case when we will be using cascading.
For example: cin>>a>>b>>c;
In the above example we can have three variables entered by the user in a single statement.We can do it in overloaded function of input too by simply returning reference to the istream.
istream means input stream, it is used to gain access of console input. Then I have used the keyword “operator”, given the symbol of it. In parameters, I have passed istream as variable “input”, now each time we want to enter data from user we will write input>> instead of cin>>. We can use any other variable as we want, then the object for which we want to enter data.
The operator overloading of + is pretty simple. We will have a caller object as our first operand which will be used using “this” pointer and an object “f” of class fraction will be passed as second operand. Addition of these two fractions will be performed and their sum will be returned.
Now let us take a look at the main function.
Main
int main()
{
fraction f1; //initializing a variable f1 of type fraction
fraction f2; //initializing a variable f2 of type fraction
cin >> f1;
cin >> f2;
fraction f3;
f3 = f1 + f2;
f3.getfraction();
}
In the main function we are getting the values of f1 and f2 from the user using the overloaded operator of console input. Then we are performing the addition of these two fractions by again using an overloaded operator of +. Their sum is being stored in f3 which is later printed on the screen.
Output
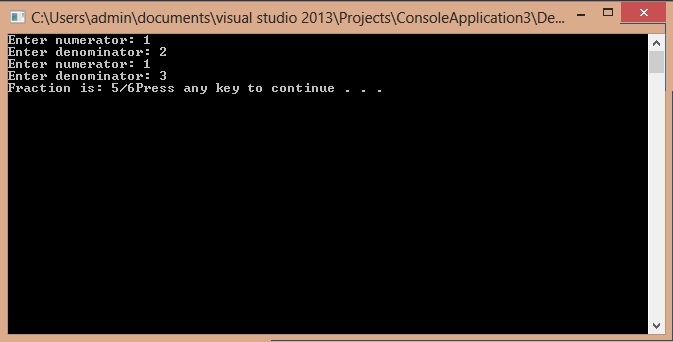