Compute General Weighted Average (GWA) using VB6
Submitted by donbermoy on Wednesday, March 26, 2014 - 04:00.
The General Weighted Average is a representation (often numerical) of the overall scholastic standing of students used for evaluation. GWA is based on the grades in all subjects taken at a particular level including subjects taken outside of the curriculum.
The Philippines has varied university grading systems. Most universities, particularly public institutions, follow the grade point system scale of 5.00 - 1.00, in which 1.00 is the highest grade and 5.00 is the lowest possible grade.
In this tutorial, we will make a program that can compute a GWA of a student with a corresponding letter grade. We will make this through computing 20% of prelim, midterm, and prefinal grade and 40% final grade to make it 100%.
Now, let's start this tutorial!
1.Let's start this tutorial by following the following steps in Microsoft Visual Basic 6.0: Open Microsoft Visual Basic 6.0, click Choose Standard EXE, and click Open.
2.Next, add only one Button named Command1 and labeled it as "Compute". Insert four textboxes named txtPre for inputting the prelim grade, txtMid for inputting the midterm grade, txtPref for inputting the prefinal grade, txtFin for inputting the Final grade. Add also Label named lblGrade for the GWA result and lblLetter for the letter grade view. You must design your interface like this:
3. Now put this code for your code module.
We have initialized variable preGrade that holds the value of txtPre for grade in prelim, variable midGrade holds the value of txtMid for midterm grade, variable prefGrade holds the value of txtPref for prefinal grade, and variable finGrade holds the value of txtFin for Final grade. Next our variable gwa holds the summation of 20% grade for prelim, 20% grade for midterm, 20% grade for prefinal, and 40% grade for final.
We have also initialized the letter grade that will remark the student if they passed or failed in a subject. From 90-100 will be marked as "A", 80-88 has a letter grade of "B", 75-79 as "C", and 65-74 will marked as "D" and it will have a red forecolor in the label which means failed.
We make also the code for textchange for textboxes to filter the input as numbers only, and will have no grade of 101 and above.
Download the source code and try it! :)
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below.
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
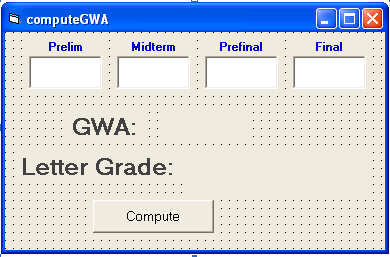
- Option Explicit
- Dim preGrade As Integer
- Dim midGrade As Integer
- Dim prefGrade As Integer
- Dim finGrade As Integer
- Dim gwa As Double
- Private Sub cmdCompute_Click()
- preGrade = Val(txtPre.Text)
- midGrade = Val(txtMid.Text)
- prefGrade = Val(txtPref.Text)
- finGrade = Val(txtFin.Text)
- gwa = (preGrade * 0.2) + (midGrade * 0.2) + (prefGrade * 0.2) + (finGrade * 0.4)
- lblGrade.Caption = gwa
- If txtPre.Text = "" Then
- MsgBox "Please fill the missing Grade"
- ElseIf txtMid.Text = "" Then
- MsgBox "Please fill the missing Grade"
- ElseIf txtPref.Text = "" Then
- MsgBox "Please fill the missing Grade"
- ElseIf txtFin.Text = "" Then
- MsgBox "Please fill the missing Grade"
- Else
- If ((gwa >= 90) And (gwa <= 100)) Then
- lblLetter.Caption = "A"
- ElseIf ((gwa >= 80) And (gwa <= 89)) Then
- lblLetter.Caption = "B"
- ElseIf ((gwa >= 75) And (gwa <= 79)) Then
- lblLetter.Caption = "C"
- ElseIf ((gwa >= 65) And (gwa <= 74)) Then
- lblLetter.ForeColor = vbRed
- lblLetter.Caption = "D"
- Else
- lblLetter.ForeColor = vbBlue
- lblLetter.Caption = "Out of Range"
- End If
- End If
- End Sub
- Private Sub txtPre_Change()
- If IsNumeric(txtPre.Text) = False Then
- txtPre.Text = ""
- MsgBox "Only accept numbers.", vbOKOnly, "Input Error!"
- End If
- If Val(txtPre.Text) >= 101 Then
- MsgBox "Grade out of range.", vbOKOnly, "Error"
- End If
- End Sub
- Private Sub txtPref_Change()
- If IsNumeric(txtPref.Text) = False Then
- txtPref.Text = ""
- MsgBox "Only accept numbers.", vbOKOnly, "Input Error!"
- End If
- If Val(txtPref.Text) >= 101 Then
- MsgBox "Grade out of range.", vbOKOnly, "Error"
- End If
- End Sub
- Private Sub txtFin_Change()
- If IsNumeric(txtFin.Text) = False Then
- txtFin.Text = ""
- MsgBox "Only accept numbers.", vbOKOnly, "Input Error!"
- End If
- If Val(txtFin.Text) >= 101 Then
- MsgBox "Grade out of range.", vbOKOnly, "Error"
- End If
- End Sub
- Private Sub txtMid_Change()
- If IsNumeric(txtMid.Text) = False Then
- txtMid.Text = ""
- MsgBox "Only accept numbers.", vbOKOnly, "Input Error!"
- End If
- If Val(txtMid.Text) >= 101 Then
- MsgBox "Grade out of range.", vbOKOnly, "Error"
- End If
- End Sub
Output:
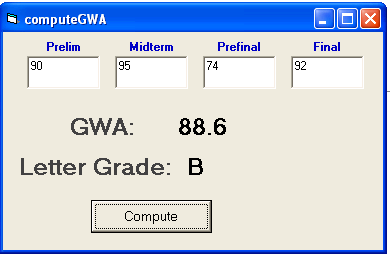
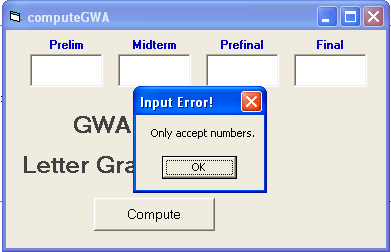
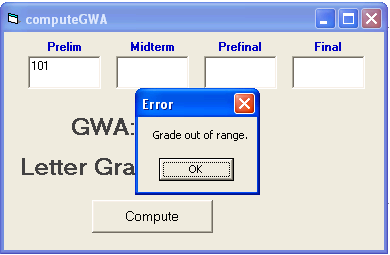