Navigation of a Student Registration Form
Submitted by janobe on Saturday, December 28, 2013 - 09:17.
In my previous tutorial I created a Student's Registration Form in Visual Basic 2008 and MySQL Database. Now, in this tutorial I will teach you how to navigate and check if how many records saved in the MySQL Database.
Lets’ begin:
1. Open the Visual Basic 2008.
2. Open the File of a Student's Registration Form.
3. Add the Five Buttons and 3 Labels in a Form. And it will look like this.
4. Add a Module named "navigation". Then, declares all the variables that you needed.
5. After that, create a Sub procedure for retrieving all the records in MySQL Database.
6. And then, create again a Sub procedure to navigate the records in MySQL Database.
7. Now, double click the Form and do following codes under the
8. Do the following codes for the First, Last, Next, Previous and New Records :
This is for the First Records.
This is for the Last Records.
This is for the Next Records.
This is for the Previous Records.
And this is for the New Records.
Download the complete Source Code and run it on your computer.
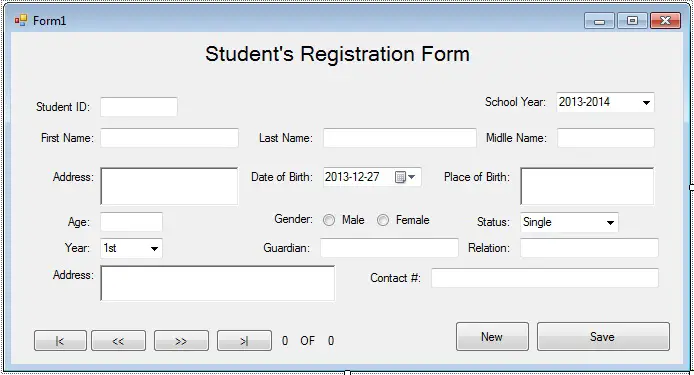
- Imports MySql.Data.MySqlClient
- Module navigation
- Public maxrows As Integer 'declare a variable maxrows as integer
- Public inc As Integer = 0 'declare a variable inc as integer and store it the value which is one.
- End Module
- 'create a sub procedure in retieving all the records in the database for the navigation.
- Public Sub selectRecords()
- With cmd
- .Connection = con
- .CommandText = "SELECT * FROM student"
- End With
- da = New MySqlDataAdapter("SELECT * FROM student", con)
- dt = New DataTable
- da.Fill(dt)
- 'stores the total number of rows in the table of the database
- maxrows = dt.Rows.Count
- End Sub
- 'create a sub procedure for the navigation of records with a parameters type is Form1
- Public Sub navigateRecords(ByVal frm As Form1)
- With frm 'frm represent as a Form1
- 'Variable inc represents the value of rows in the table.
- 'whatever the value of the inc, this will be the row of records that will retrieve in the database.
- .txtid.Text = dt.Rows(inc).Item("s_id")
- .txtFname.Text = dt.Rows(inc).Item("s_fname")
- .txtLname.Text = dt.Rows(inc).Item("lastname")
- .txtMname.Text = dt.Rows(inc).Item("middlename")
- .rchAddress.Text = dt.Rows(inc).Item("s_address")
- .dtpDbirth.Text = dt.Rows(inc).Item("s_bday")
- .rchPbirth.Text = dt.Rows(inc).Item("s_bplace")
- .txtAge.Text = dt.Rows(inc).Item("s_age")
- If dt.Rows(inc).Item("s_gender") = "Female" Then 'check if the gender is male or female.
- .rdoFemaleMale.Checked = True
- Else
- .rdioMale.Checked = True
- End If
- .cboStatus.Text = dt.Rows(inc).Item("s_status")
- .cboYear.Text = dt.Rows(inc).Item("yr")
- .cbosy.Text = dt.Rows(inc).Item("sy")
- .txtGuardian.Text = dt.Rows(inc).Item("s_guardian")
- .txtRelation.Text = dt.Rows(inc).Item("s_guardian_relation")
- .rchGaddress.Text = dt.Rows(inc).Item("s_guardian_add")
- .txtContact.Text = dt.Rows(inc).Item("s_guardian_contact")
- End With
- End Sub
Form_Load
.
- selectRecords() 'A SubName in rettrieving records in the database.
- lbl_Increment.Text = inc 'Set the variable inc is equal to the Label.
- lbl_MaxRecord.Text = maxrows - 1 'Set the total numbers of rows in the table - 1 is equal to the Label.
- Private Sub btn_First_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btn_First.Click
- Try
- selectRecords() 'A SubName in retrieving all the records in the database
- inc = 0 'store the value in the variable inc which is 0 for the first row.
- lbl_Increment.Text = inc 'Pass the value of a variable inc into the Label.
- navigateRecords(Me) 'A SubName for setting up the navigation of the records
- Catch ex As Exception
- End Try
- End Sub
- Private Sub btn_Last_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btn_Last.Click
- Try
- selectRecords() 'A SubName in retrieving all the records in the database
- If inc <> maxrows - 1 Then 'Check if the variable inc is not equal to the total number of rows in the table.
- inc = maxrows - 1 'The total number of rows in the table minus one is equal to the variable inc
- navigateRecords(Me) 'A SubName for setting up the navigation of the records
- lbl_Increment.Text = maxrows - 1 'The total number of rows in the table minus one is equal to the Label.
- End If
- Catch ex As Exception
- End Try
- End Sub
- Private Sub btn_Next_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btn_Next.Click
- selectRecords() 'A SubName in retrieving all the records in the database
- If inc <> maxrows - 1 Then 'Check if the variable inc is not equal to the total number of rows in the table.
- inc = inc + 1 'The variable inc + 1 is equal to variable inc
- 'Every click the button the rows will increment by 1
- lbl_Increment.Text = inc ''Set your variable inc is equal to the Label
- navigateRecords(Me) 'A SubName for setting up the navigation of the records
- Else
- If maxrows + 1 Then 'check if the total numbers of rows is higher of 1.
- MsgBox("No more rows", MsgBoxStyle.Information) 'The message box will appear and has a message of "No more rows"
- End If
- End If
- End Sub
- Private Sub btn_Previous_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btn_Previous.Click
- selectRecords() 'A SubName in retrieving all the records in the database
- If inc > 0 Then 'Check if the variable inc is greater than 0
- inc = inc - 1 'The variable inc - 1 is equal to variable inc
- 'Every click the button the rows will decrease by 1
- lbl_Increment.Text = inc 'Set your variable inc is equal to the Label
- navigateRecords(Me) 'A SubName for setting up the navigation of the records
- Else
- If inc - 1 Then 'check if variable inc is decrease of 1.
- MsgBox("First records", MsgBoxStyle.Information) 'The message box will appear and has a message of "No more rows"
- End If
- End If
- End Sub
- Private Sub btn_New_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btn_New.Click
- Try
- cleartext(Me) 'call for clearing the TextBox
- Call Form1_Load(sender, e) 'call your first load to set again the AutoNumber.
- Catch ex As Exception
- End Try
- End Sub
Add new comment
- 2980 views