Change ProgressBar Color in VB.NET
Submitted by donbermoy on Monday, April 20, 2015 - 23:41.
Usually, the color of the progressbar is green. Now, we will create a program that changes the progressbar color in vb.net.
Now, let's start this tutorial!
1. Let's start with creating a Windows Form Application for this tutorial by following the following steps in Microsoft Visual Studio: Go to File, click New Project, and choose Windows Application.
2. Next, add three ProgressBar named ProgressBar1, ProgressBar2, and ProgressBar3. Add also a timer named Timer1 to fill in the progressbar.
You must design your interface like this:
3. Then we will code for changing the color of our progressbars.
We will declare an auto function named SendMessage that will access the user32.dll because the color customization is found there.
Declare the colors using the enum property.
Now, we will create a sub procedure named ChangeProgBarColor so that we can now change the color of the progressbars.
Code for the Timer1_Tick to process the progressbar and increments its value.
Lastly, in the Form_Load, call the sub procedure ChangeProgBarColor to color the progressbars and start the time.
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below.
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
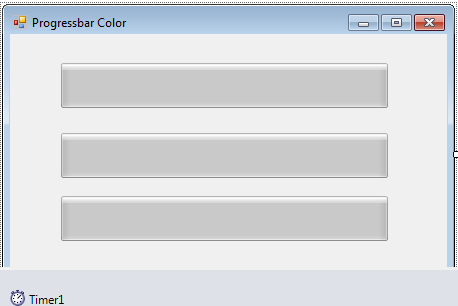
- Declare Auto Function SendMessage Lib "user32.dll" (ByVal hWnd As IntPtr, ByVal msg As Integer, ByVal wParam As Integer, ByVal lParam As Integer) As Integer
- Enum ProgressBarColor
- Green = &H1
- Red = &H2
- Yellow = &H3
- End Enum
- Private Shared Sub ChangeProgBarColor(ByVal ProgressBar_Name As Windows.Forms.ProgressBar, ByVal ProgressBar_Color As ProgressBarColor)
- SendMessage(ProgressBar_Name.Handle, &H410, ProgressBar_Color, 0)
- End Sub
- Private Sub Timer1_Tick(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Timer1.Tick
- ProgressBar1.Value += 1
- ProgressBar2.Value += 1
- ProgressBar3.Value += 1
- If ProgressBar1.Value = 99 And ProgressBar2.Value = 99 And ProgressBar3.Value = 99 Then
- Timer1.Stop()
- End If
- End Sub
- Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
- ChangeProgBarColor(ProgressBar1, ProgressBarColor.Red)
- ChangeProgBarColor(ProgressBar2, ProgressBarColor.Yellow)
- ChangeProgBarColor(ProgressBar3, ProgressBarColor.Green)
- Timer1.Enabled = True
- End Sub
Output:
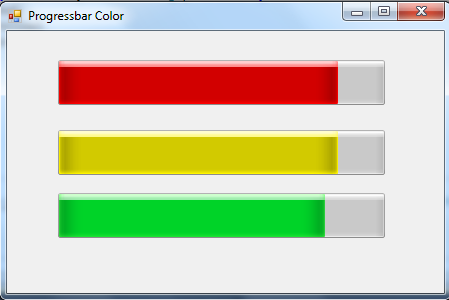
Add new comment
- 5278 views