Last day, I had created a tutorial on Text to Binary Conversion. But today, we will have a lecture on how to convert a binary into a text.
Here in this tutorial, we will create our own program to create a text to binary conversion. :)
So, now let's start this tutorial!
1. Let's start with creating a Windows Form Application for this tutorial by following the following steps in Microsoft Visual Studio: Go to
File, click
New Project, and choose
Windows Application.
2. Next, add two TextBox named
TextBox1 to input a binary digit and
TextBox2 for displaying the text conversion of the binary you have inputted. Add also a button named
Button1. Design your layout like this one below:
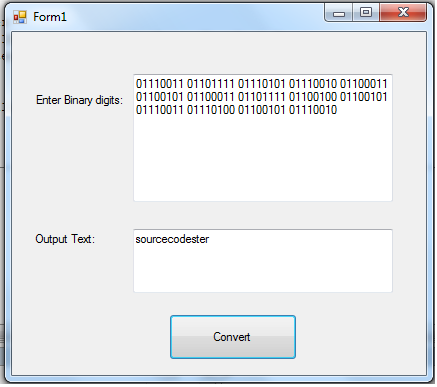
3. Put this code to your
Button1_Click. This will display the conversion of the binary to text.
Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click
Dim tempString As String = ""
Dim Character
As String = System
.Text.RegularExpressions.Regex.Replace(TextBox1
.Text,
"[^01]",
"") Dim Bytes((Character.Length / 8) - 1) As Byte
For Index As Integer = 0 To Bytes.Length - 1
Bytes(Index) = Convert.ToByte(Character.Substring(Index * 8, 8), 2)
Next
tempString = System.Text.ASCIIEncoding.ASCII.GetString(Bytes)
TextBox2.Text = tempString
End Sub
Explanation:
We initialized
tempString as String to nothing and a
Character as String that holds a regular expression of the textbox1 to 1 or 0 only. Next, we declare a Bytes variable that have the length of 8 bits as we have the code
((Character.Length / 8) - 1). We have a For Next loop that we initialized an
Index variable as an integer to start from 0 to the Bytes variable length minus 1 because it is an array of bytes (
Note: array starts at 0 so we minus 1 it). Next, the Bytes array index will be equal to the conversion of bytes from the characters inputted in textbox1.The tempString variable will get the equivalent string of the variable Bytes. And then the value of the binary inputted will now be equal to the variable tempString and will be displayed in textbox2.
Output:
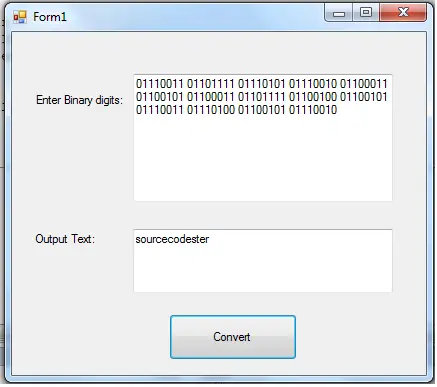
Download the source code below and try it! :)
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below and hire me.
Best Regards,
Engr. Lyndon R. Bermoy
IT Instructor/System Developer/Android Developer
Mobile: 09079373999
Telephone: 826-9296
E-mail:[email protected]
Visit and like my page on Facebook at: Bermz ISware Solutions
Subscribe at my YouTube Channel at: SerBermz