Connecting MySQL Database and Visual Basic 2008 Using a Class
Submitted by janobe on Sunday, January 5, 2014 - 10:04.
In this tutorial I will teach you how to use a class in connecting the MySQL Database and Visual Basic 2008. What is a class? A Class is a group of object that defines its functionality as a set of properties and the methods that will respond to. And it is also a container of your data and code. Wherein you can limit the data that you’re accessing. In Object-Oriented Programming(OOP) , classes, methods and properties help you create a complete application.
Let's begin:
Step1:
Create a Database.
Step2:
Open the Visual Basic 2008, create a Project, and set the Form just like this.
Step3:
Go to the solution explorer, right click the name of your project, click the add and add the class and name it “connection”.
Step4:
Inside the class(connection), do the following codes for the connection of MySQL Database.
Discriptions: Property has function that can be read-only,write-only and read-write. GET accessor provides to read the access and the Set accessor provides to write the access.
Step5:
Go back to the design views, double click the button and do the following codes.
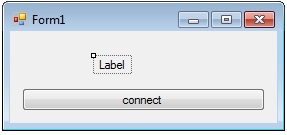
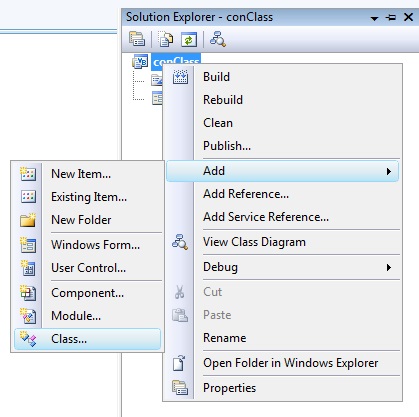
- Imports MySql.Data.MySqlClient
- Public Class connection
- 'set a string connection
- Private strCon As String = "server=localhost;user id=root;database=test"
- 'set a connection
- Private Function Mycon() As MySqlConnection
- Return New MySqlConnection(strCon)
- End Function
- 'pass the value of Mycon to Connection
- Private Connection As MySqlConnection = Mycon()
- 'set a property of connection
- Property con() As MySqlConnection
- Get
- 'return the connection
- Return Connection
- End Get
- Set(ByVal value As MySqlConnection)
- 'set the connection
- Connection = value
- End Set
- End Property
- End Class
- Public Class Form1
- Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click
- 'call a class and set a new class of connection
- Dim Mycon As connection = New connection
- If Button1.Text = "connect" Then 'checking the text of a button
- 'openning the connection
- Mycon.con.Open()
- If Mycon.con.State = ConnectionState.Open Then 'check if the connection is open or not.
- 'result
- Button1.Text = "disconnect" 'change the text of the button
- Label1.Text = "Connected" 'change the text of the label
- Else
- Button1.Text = "connect" 'change the text of the button
- Label1.Text = "Disconnected"
- End If
- Else
- 'clossing the connection
- If Mycon.con.State = ConnectionState.Open Then
- Button1.Text = "disconnect" 'change the text of the button
- Label1.Text = "Connected" 'change the text of the label
- Else
- Button1.Text = "connect" 'change the text of the button
- Label1.Text = "Disconnected" 'change the text of the label
- End If
- End If
- End Sub
- End Class