In one of our previous tutorials, we discuss on How to "
Connect MySQL Database using Visual Basic.Net". We also discuss on How to "
Insert and Read data from MySQL Database using Visual Basic". This time, we are going to answer some of the questions found in the internet like
“How to fill combobox with a data stored in a table in MySQL Database” and
“How to populate combobox with MySQL Database”.
Let’s start building our project by designing first our User Interface and the final output of this application is looks like shown below.
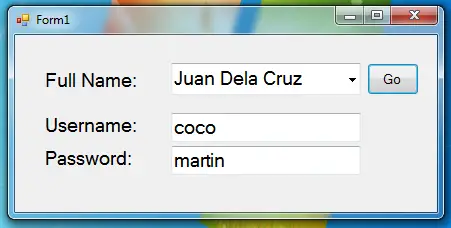
So we need to add three labels and change the each text to Full Name, Username and Password. Then add a button and name it as “btngo” and change the text property into “Go”. Next add two textbox and name the first one as “txtuser” and place it beside the Username, then the other one name it as “txtpass” and place it beside “Password”. And finally add a combobox and leave all the property to its default settings.
Next, double click the form. And above public class add the following code.
But to make sure you have already added the reference of mysql. And if you don’t know how to do it, just read follow this article called "
Connect MySQL Database using Visual Basic.Net" and have some background how to connect MySQL to Visual Basic.
Imports MySql.Data.MySqlClient
Then, under public class add the following code and now it looks like as shown below.
Imports MySql.Data.MySqlClient
Public Class Form1
Dim con As New MySqlConnection
'MySqlCommand It represents a SQL statement to execute against a MySQL Database
Dim cmd As New MySqlCommand
'Represents a set of data commands and a database connection that
'are used to fill a dataset and update a MySQL database. This class cannot be inherited.
Dim da As New MySqlDataAdapter
Next, on the form1_load. Add this following code:
This code is responsible for populating the combobox with data stored from the database.
con.ConnectionString = ("server=localhost;user id=root;password=;database=test")
Try
'we open Connection
con.Open()
With cmd
.Connection = con
.CommandText = "SELECT `fullname` from users;"
End With
'declare dt as new datatable
Dim dt As New DataTable
With ComboBox1
da.SelectCommand = cmd
'it fills the da values into dt
da.Fill(dt)
'dt provides the data surce of combobox
.DataSource = dt
'specify the what to display
.DisplayMember = "fullname"
'and the value
.ValueMember = "fullname"
End With
Catch ex As Exception
End Try
Before we forgot, heres the structure and the data of the table to be used in this project.
--
-- Dumping data for table `users`
--
(2, 'Juan Dela Cruz', 'coco', 'martin'),
(3, 'Deigo Tarzan', 'Diego', 'tarzan'),
(5, 'ecg', 'dsf', 'sdf');
Next let’s proceed, double click the “btngo” button and add the following code:
Dim table As New DataTable
Try
'Gets or sets an SQL statement or stored procedure used to select records in the database.
With cmd
.Connection = con
.CommandText = "SELECT * from users where `fullname`='" & ComboBox1.SelectedValue & "';"
End With
da.SelectCommand = cmd
da.Fill(table)
'it gets the data from specific column and fill it into textbox
txtuser.Text = table.Rows(0).Item(2)
txtpass.Text = table.Rows(0).Item(3)
da.Dispose()
Catch ex As Exception
End Try
And all the code in this application is should look like as shown below.
Imports MySql.Data.MySqlClient
Public Class Form1
Dim con As New MySqlConnection
'MySqlCommand It represents a SQL statement to execute against a MySQL Database
Dim cmd As New MySqlCommand
'Represents a set of data commands and a database connection that
'are used to fill a dataset and update a MySQL database. This class cannot be inherited.
Dim da As New MySqlDataAdapter
Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
con.ConnectionString = ("server=localhost;user id=root;password=;database=test")
Try
'we open Connection
con.Open()
With cmd
.Connection = con
.CommandText = "SELECT `fullname` from users;"
End With
'declare dt as new datatable
Dim dt As New DataTable
With ComboBox1
da.SelectCommand = cmd
'it fills the da values into dt
da.Fill(dt)
'dt provides the data surce of combobox
.DataSource = dt
'specify the what to display
.DisplayMember = "fullname"
'and the value
.ValueMember = "fullname"
End With
Catch ex As Exception
End Try
End Sub
Private Sub btngo_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btngo.Click
Dim table As New DataTable
Try
'Gets or sets an SQL statement or stored procedure used to select records in the database.
With cmd
.Connection = con
.CommandText = "SELECT * from users where `fullname`='" & ComboBox1.SelectedValue & "';"
End With
da.SelectCommand = cmd
da.Fill(table)
'it gets the data from specific column and fill it into textbox
txtuser.Text = table.Rows(0).Item(2)
txtpass.Text = table.Rows(0).Item(3)
da.Dispose()
Catch ex As Exception
End Try
End Sub
End Class
Then, to test this program just press “F5”.