How to Retrieve Data in the TextBox Based on ComboBox in VB.Net and MS Access Database
Submitted by janobe on Tuesday, July 9, 2019 - 12:03.
Now, in this tutorial, I’m going to teach you how to retrieve data in the textbox based on combobox in VB.Net and MS Access Database. This method has the ability to display all the data in a certain textbox by selecting the data in the combobox. If you this function the output will be displayed in all 5 textboxes.
Download the complete source code and run it on your computer.
For any questions about this article. You can contact me @
Email – [email protected]
Mobile No. – 09305235027 – TNT
Or feel free to comment below
Creating an Application
Step 1
Open Microsoft Visual Studio 2015 and create a new windows form application.Step 2
Do the form just like shown below.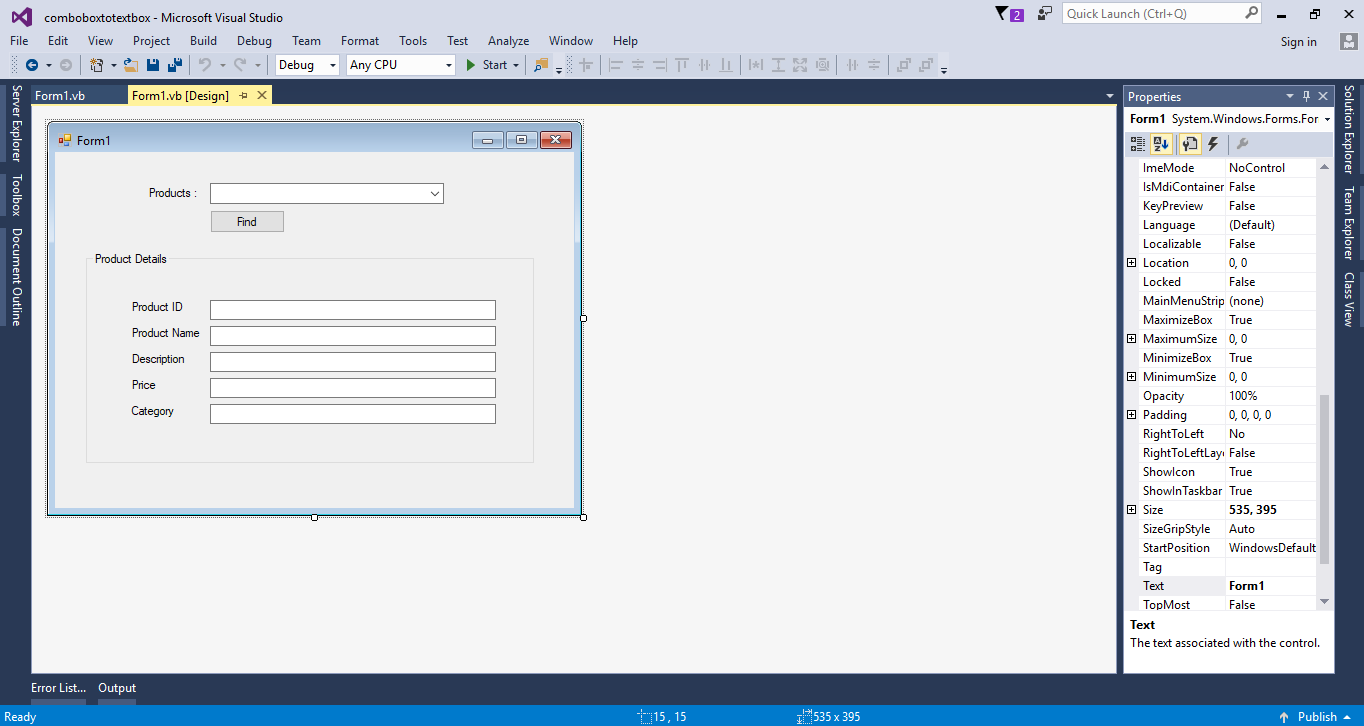
Step 3
Press F7 to open the code editor. In the code editor, add a namespace forOLeDB
to access OLeDB
libraries.
- imports System.Data.OleDb;
Step 4
Create a connection between the access database and vb.net. After that, declare all the classes that are needed.- Dim con As OleDbConnection = New OleDbConnection("Provider=Microsoft.ACE.OLEDB.12.0;Data Source=" & Application.StartupPath & "\dbproducts.accdb")
- Dim cmd As OleDbCommand
- Dim da As OleDbDataAdapter
- Dim dt As DataTable
- Dim sql As String
- Dim maxrow As Integer
Step 5
Create a method for filling data in the combobox.- Private Sub load_cbo(sql As String, cbo As ComboBox)
- Try
- con.Open()
- cmd = New OleDb.OleDbCommand
- da = New OleDb.OleDbDataAdapter
- dt = New DataTable
- With cmd
- .Connection = con
- .CommandText = sql
- End With
- da.SelectCommand = cmd
- da.Fill(dt)
- cbo.DataSource = dt
- cbo.DisplayMember = "Product"
- cbo.ValueMember = "ProductID"
- Catch ex As Exception
- MsgBox(ex.Message)
- Finally
- con.Close()
- da.Dispose()
- End Try
- End Sub
Step 6
Create a function for getting the total number of rows in the database.- Private Function get_maxrow(sql)
- Try
- con.Open()
- cmd = New OleDb.OleDbCommand
- da = New OleDb.OleDbDataAdapter
- dt = New DataTable
- With cmd
- .Connection = con
- .CommandText = sql
- End With
- da.SelectCommand = cmd
- da.Fill(dt)
- maxrow = dt.Rows.Count
- Catch ex As Exception
- MsgBox(ex.Message)
- Finally
- con.Close()
- da.Dispose()
- End Try
- Return maxrow
- End Function
Step 7
Write the following code for filling the data in the combobox in the first load of the form.- Private Sub Form1_Load(sender As Object, e As EventArgs) Handles MyBase.Load
- sql = "Select * From tblproducts"
- load_cbo(sql, cboProduct)
- End Sub
Step 8
Write the following code for retrieving the data in the textbox when the button is clicked.- Private Sub btnFind_Click(sender As Object, e As EventArgs) Handles btnFind.Click
- sql = "Select * From tblproducts Where ProductID = " & cboProduct.SelectedValue & ""
- maxrow = get_maxrow(sql)
- If maxrow > 0 Then
- With dt.Rows(0)
- txtProductID.Text = .Item("ProductID")
- txtProductName.Text = .Item("Product")
- txtDescription.Text = .Item("Description")
- txtPrice.Text = .Item("Price")
- txtCategory.Text = .Item("Category")
- End With
- End If
- End Sub
Add new comment
- 2282 views