In this tutorial we will create a
Simple CRUD With Django Framework. Django is an advanced Web framework written in Python that makes use of the model view controller (MVC) architectural pattern. The official project site describes Django as "a high-level Python Web framework that encourages rapid development and clean, pragmatic design. And It’s free and open source to every developer in the world.
Getting Started
First you will have to download & install the Python IDLE's, here's the link for the Integrated Development And Learning Environment for Python
https://www.python.org/downloads/.
After Python IDLE's is installed, open the command prompt then type "pip install Django", and hit enter.
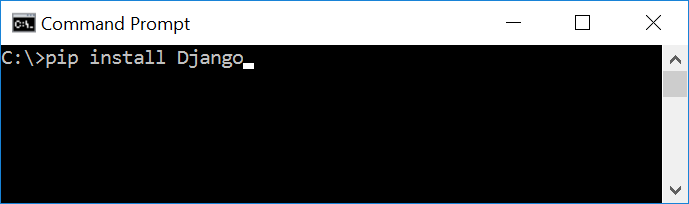
Wait for the django to be downloaded and installed at the same time. Then After that type "python -m django version" and hit enter to check if django is installed and what version of django is.
Creating the App
After setting django we will now create the web app for the web server. First create a new folder named "Django CRUD", then cd to a newly created folder, then type "django-admin startproject web" and hit enter. A new folder will be created on the directory named 'web'.
Running The Server
After creating a project, cd to the newly created directory, then type "manage.py runserver" and hit enter to start the server running. The "manage.py" is a command of django-admin that utilize the administrative tasks of python web framework.
Here is the image of python web server:
Note: Type '127.0.0.1:8000' in the url browser to view the server. When there is code changes in the server just (ctrl + C) to command prompt to stop the server from running, then start again to avoid errors.
Creating The Website
This time will now create the web app to display the web models. First locate the directory of the app via command prompt cd, then type "manage.py startapp crud" and hit enter. A new directory will be create inside the app named "crud".
Setting up The URL
This time will now create a url address to connect the app from the server. First Go to web directory, then open urls via Python IDLE's or any text editor. Then import "include" module beside the url module and import additional module to make a redirect url to your site "from . import views". After that copy/paste the code below inside the urlpatterns.
url(r'^$', views.index_redirect, name='index_redirect'),
url(r'^crud/', include('crud.urls')),
It will be look like this:
from django.conf.urls import include,url
from django.contrib import admin
from . import views
urlpatterns = [
url(r'^$', views.index_redirect, name='index_redirect'),
url(r'^crud/', include('crud.urls')),
url(r'^admin/', admin.site.urls),
]
Then after that create a view that will catch the redirect url. To do that create a file "views.py" then copy/paste the code below.
from django.shortcuts import redirect
def index_redirect(request):
return redirect('/crud/')
Creating The Path For The Pages
Now that we set the connect we will now create a path for the web pages. All you have to do first is to go to crud directory, then copy/paste the code below and save it inside "crud" directory named 'urls.py' The file name must be urls.py or else there will be an error in the code.
from django.conf.urls import url
from . import views
urlpatterns= [
url(r'^$', views.index, name='index'),
url(r'^create$', views.create, name='create'),
url(r'^edit/(?P<id>\d+)$', views.edit, name='edit'),
url(r'^edit/update/(?P<id>\d+)$', views.update, name='update'),
url(r'^delete/(?P<id>\d+)$', views.delete, name='delete'),
]
Creating A Static Folder
This time we will create a directory to import the statics and templates. First go to the crud directory then create a directory called "static", after that create a sub directory called "crud". You'll notice that it is the same as your main app directory name, to assure the absolute link. This is where you import the css, js, etc directory.
Creating The Views
The views contains the interface of the website. This is where you assign the html code for rendering it to django framework and contains a methods that call a specific functions. To do that first open the views.py, the copy/paste the code below.
from django.shortcuts import render, redirect
from .models import Member
# Create your views here.
def index(request):
members = Member.objects.all()
context = {'members': members}
return render(request, 'crud/index.html', context)
def create(request):
member = Member(firstname=request.POST['firstname'], lastname=request.POST['lastname'])
member.save()
return redirect('/')
def edit(request, id):
members = Member.objects.get(id=id)
context = {'members': members}
return render(request, 'crud/edit.html', context)
def update(request, id):
member = Member.objects.get(id=id)
member.firstname = request.POST['firstname']
member.lastname = request.POST['lastname']
member.save()
return redirect('/crud/')
def delete(request, id):
member = Member.objects.get(id=id)
member.delete()
return redirect('/crud/')
Creating The Models
Now that we're done with the views we will then create a models. Models is module that will store the database information to django. To do that locate and go to crud directory, then open the "models.py" after that copy/paste the code.
from django.db import models
# Create your models here.
class Member(models.Model):
firstname = models.CharField(max_length=40)
lastname = models.CharField(max_length=40)
def __str__(self):
return self.firstname + " " + self.lastname
Registering The App To The Server
Now that we created the interface we will now then register the app to the server. To do that go to the web directory, then open "settings.py" via Python IDLE's or any text editor. Then copy/paste this script inside the INSTALLED_APP variables 'crud'.
It will be like this:
INSTALLED_APPS = [
'crud',
'django.contrib.admin',
'django.contrib.auth',
'django.contrib.contenttypes',
'django.contrib.sessions',
'django.contrib.messages',
'django.contrib.staticfiles',
]
Creating The Mark up Language
Now we will create the html interface for the django framework. First go to crud directory, then create a directory called "templates" and create a sub directory on it called crud.
base.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" name="viewport" content="width=device-width, initial-scale=1"/>
{% load static %}
<link rel="stylesheet" type="text/css" href="{% static 'crud/css/bootstrap.css' %}"/>
</head>
<body>
<nav class="navbar navbar-default">
<div class="container-fluid">
<a class="navbar-brand" href="https://sourcecodester.com">Sourcecodester</a>
</div>
</nav>
<div class="col-md-3"></div>
<div class="col-md-6 well">
<h3 class="text-primary">Python - Simple CRUD With Django Framework</h3>
<hr style="border-top:1px dotted #000;"/>
{% block body %}
{% endblock %}
</div>
</body>
</html>
Save it as "base.html" inside the crud directory "sub directory of templates".
index.html
{% extends 'crud/base.html' %}
{% block body %}
<form class="form-inline" action="create" method="POST">
{% csrf_token %}
<div class="form-group">
<label for="firstname">Firstname</label>
<input type="text" name="firstname" class="form-control" style="width:30%;" required="required"/>
<label for="lastname">Lastname</label>
<input type="text" name="lastname" class="form-control" style="width:30%;" required="required"/>
<button type="submit" class="btn btn-sm btn-primary"><span class="glyphicon glyphicon-plus"></span> ADD</button>
</div>
</form>
<br />
<table class="table table-bordered">
<thead class="alert-warning">
<tr>
<th>Firstname</th>
<th>Lastname</th>
<th>Action</th>
</tr>
</thead>
<tbody>
{% for member in members %}
<tr>
<td>{{ member.firstname }}</td>
<td>{{ member.lastname }}</td>
<td><center><a class="btn btn-sm btn-warning" href="edit/{{ member.id }}"><span class="glyphicon glyphicon-edit"></span> Edit</a> <a class="btn btn-sm btn-danger" href="delete/{{ member.id }}"><span class="glyphicon glyphicon-trash"></span> Delete</a></center></td>
</tr>
{% endfor %}
</tbody>
</table>
{% endblock %}
Save it as "index.html" inside the crud directory "sub directory of templates".
edit.html
{% extends 'crud/base.html' %}
{% block body %}
<form method="POST" action="update/{{ members.id }}">
{% csrf_token %}
<div class="form-group">
<label for="firstname">Firstname</label>
<input type="text" name="firstname" value="{{ members.firstname }}" required="required"/>
</div>
<div class="form-group">
<label for="lastname">Lastname</label>
<input type="text" name="lastname" value="{{ members.lastname }}" required="required"/>
</div>
<div class="form-group">
<button type="submit" class="btn btn-sm btn-warning"><span class="glyphicon glyphicon-edit"></span> Update</button>
</div>
</form>
{% endblock %}
Save it as "edit.html" inside the crud directory "sub directory of templates".
Migrating The App To The Server
Now that we done in setting up all the necessary needed, we will now then make a migration and migrate the app to the server at the same time. To do that open the command prompt then cd to the "web" directory, then type "manage.py makemigrations" and hit enter. After that type again "manage.py migrate" then hit enter.
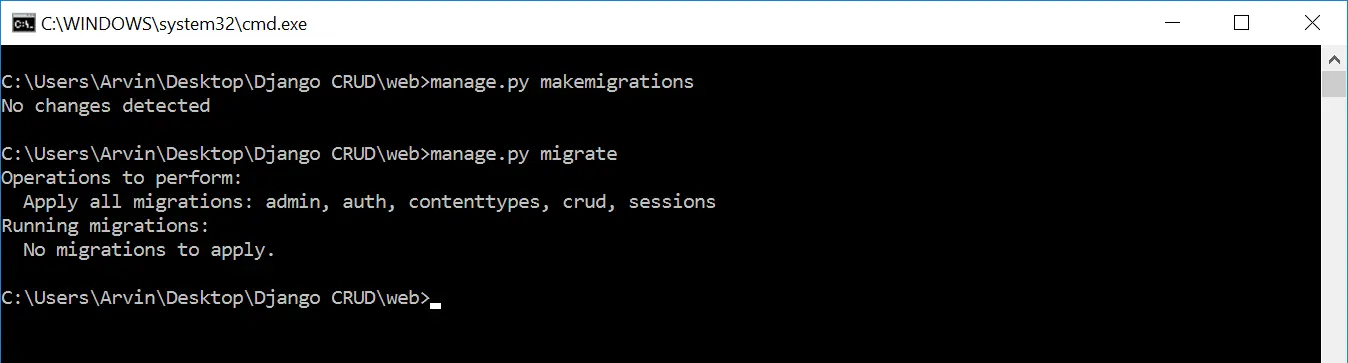
Now try to run the server again, and see if all things are done.
There you have it we successfully created a
Simple CRUD With Django Framework Using Python. I hope that this simple tutorial help you understand some complicated things about python programming. For more updates and tutorials just kindly visit this site. Enjoy Coding!!!