Python - How To Use DataTables With Django
Submitted by razormist on Tuesday, August 8, 2017 - 18:28.
In this tutorial we will try to use jQuery DataTables With Django Web Framework. Django is an advanced Web framework written in Python that makes use of the model view controller (MVC) architectural pattern. The official project site describes Django as "a high-level Python Web framework that encourages rapid development and clean, pragmatic design. And It’s free and open source to every developer in the world.
Getting Started
First you will have to download & install the Python IDLE's, here's the link for the Integrated Development And Learning Environment for Python https://www.python.org/downloads/.
Next is to download Data Tables. DataTables is a plug-in for the jQuery Javascript library. It is a highly flexible tool,that is easy to use and convinient. Here is the link for Data Tables Plugins https://datatables.net/
After Python IDLE's is installed, open the command prompt then type "pip install Django", and hit enter.
Wait for the django to be downloaded and installed at the same time. Then After that type "python -m django version" and hit enter to check if django is installed and what version of django is.
Creating the App
After setting django we will now create the web app for the web server. First create a new folder named "Python - How To Use DataTables With Django", then cd to a newly created folder, then type "django-admin startproject main" and hit enter. A new folder will be created on the directory named 'main'.
Running The Server
After creating a project, cd to the newly created directory, then type "manage.py runserver" and hit enter to start the server running. The "manage.py" is a command of django-admin that utilize the administrative tasks of python web framework.
Here is the image of python web server:
Note: Type '127.0.0.1:8000' in the url browser to view the server. When there is code changes in the server just (ctrl + C) to command prompt to stop the server from running, then start again to avoid errors.
Creating The Website
This time will now create the web app to display the web models. First locate the directory of the app via command prompt cd, then type "manage.py startapp table" and hit enter. A new directory will be create inside the app named "table".
Setting up The URL
This time will now create a url address to connect the app from the server. First Go to main directory, then open urls via Python IDLE's or any text editor. Then import "include" module beside the url module and import additional module to make a redirect url to your site "from main import views". After that copy/paste the code below inside the urlpatterns.
It will be look like this:
Then after that create a view that will catch the redirect url. To do that create a file "views.py" then copy/paste the code below and save it as "views.py".
Creating The Path For The Pages
Now that we set the connect we will now create a path for the web pages. All you have to do first is to go to table directory, then copy/paste the code below and save it inside "table" directory named 'urls.py' The file name must be urls.py or else there will be an error in the code.
Creating A Static Folder
This time we will create a directory to import the statics and templates. First go to the registration directory then create a directory called "static", after that create a sub directory called "table". You'll notice that it is the same as your main app directory name, to assure the absolute link. This is where you import the css, js, etc directory.
Creating The Views
The views contains the interface of the website. This is where you assign the html code for rendering it to django framework. To do that first open the views.py, the copy/paste the code below.
Creating The Models
Now that we're done with the views we will then create a models. Models is module that will store the database information to django. To do that locate and go to table directory, then open the "models.py" after that copy/paste the code.
After that go to table directory then open "admin.py" and copy/paste the code below.
Registering The App To The Server
Now that we created the interface we will now then register the app to the server. To do that go to the main directory, then open "settings.py" via Python IDLE's or any text editor. Then copy/paste this script inside the INSTALLED_APP variables 'table'.
It will be like this:
Creating An Account
After registering the app we will now create a user account that will login to the server. To do that cd to "main" directory, then type "manage.py createsuperuser" and hit enter. Fill up the required field to make to your registration ready.
Storing Information To The Database
After creating the account, run the server again then type "http://127.0.0.1:8000/admin" on the url address and hit enter. You notice that you are directed in the django admin login page, try to login your newly created account.
After logging in click Members then fill up the required field that will be later display in the table.
Creating The Mark up Language
Now we will create the html interface for the django framework. First go to table directory, then create a directory called "templates" and create a sub directory on it called table.
base.html
Save it as "base.html" inside the table directory "sub directory of templates".
index.html
Migrating The App To The Server
Now that we done in setting up all the necessary needed, we will now then make a migration and migrate the app to the server at the same time. To do that open the command prompt then cd to the "main" directory, then type "manage.py makemigrations" and hit enter. After that type again "manage.py migrate" then hit enter.
Now try to run the server again, and see if all things are done.
There you have it we successfully implement jQuery DataTables With Django Web Framework. I hope that this simple tutorial help you for what you are looking for. For more updates and tutorials just kindly visit this site. Enjoy Coding!!!
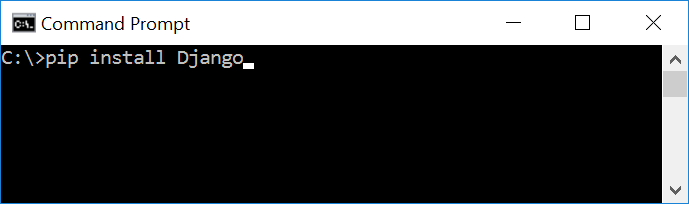
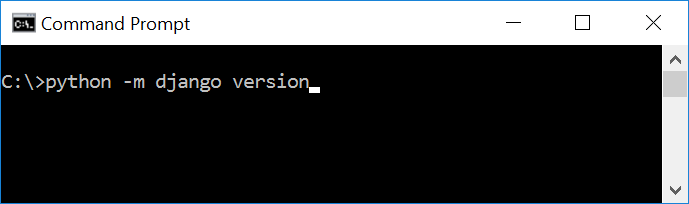

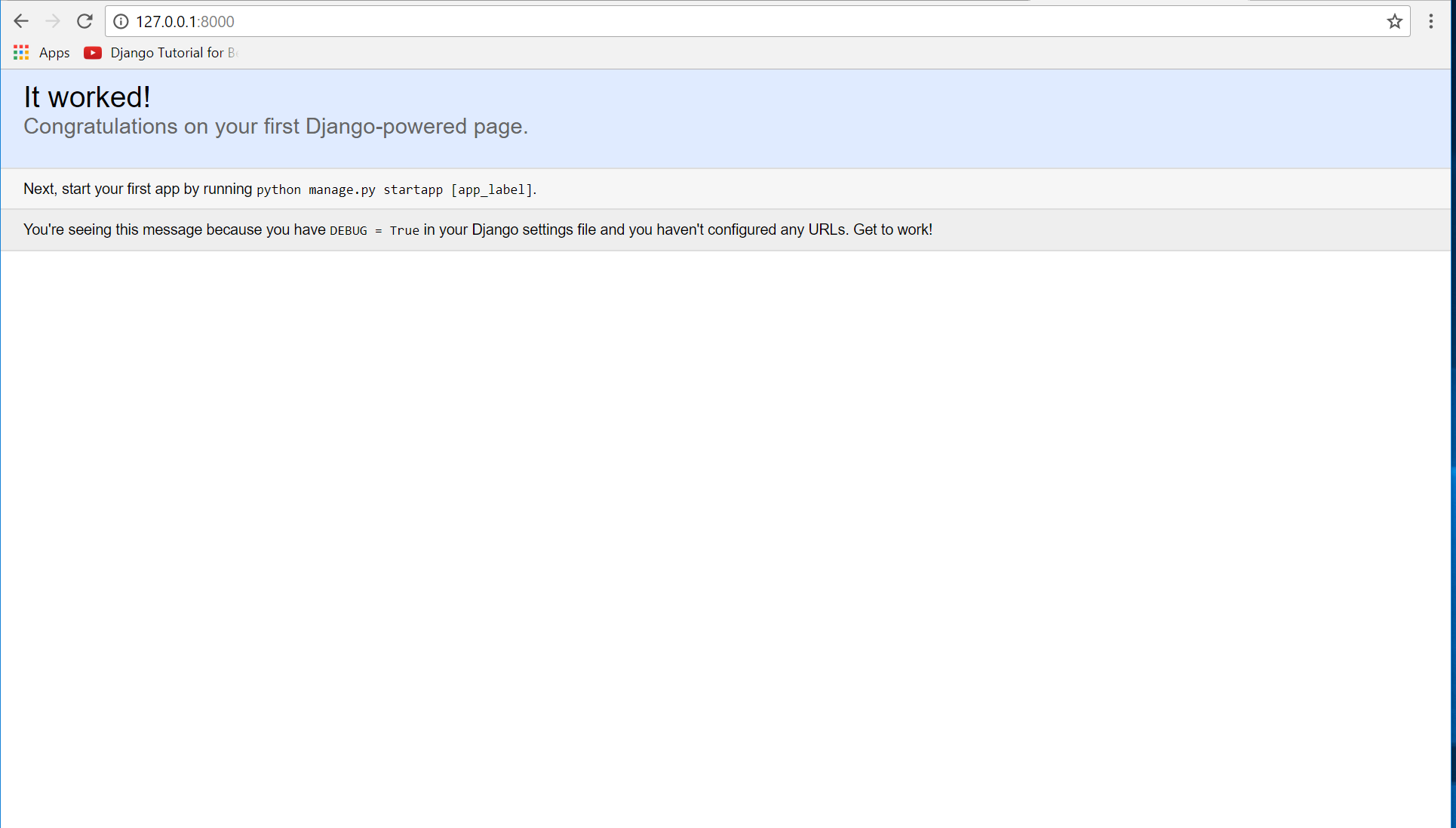

- url(r'^$', views.index_redirect, name='index_redirect'),
- url(r'^table/', include('table.urls')),
- from django.conf.urls import include, url
- from django.contrib import admin
- from main import views
- urlpatterns = [
- url(r'^$', views.index_redirect, name='index_redirect'),
- url(r'^table/', include('table.urls')),
- url(r'^admin/', admin.site.urls),
- ]
- from django.shortcuts import redirect
- def index_redirect(request):
- return redirect('/table/')
- from django.conf.urls import url
- from . import views
- urlpatterns = [
- url(r'^$', views.index, name='index'),
- from django.shortcuts import render
- from .models import Member
- # Create your views here.
- def index(request):
- all_members = Member.objects.all()
- return render(request, 'table/index.html', {'all_members': all_members})
- from django.db import models
- # Create your models here.
- class Member(models.Model):
- firstname = models.CharField(max_length=20)
- lastname = models.CharField(max_length=20)
- age = models.CharField(max_length=3)
- email = models.EmailField(max_length=50)
- address = models.CharField(max_length=50)
- def __str__(self):
- return self.firstname + " " + self.lastname
- from django.contrib import admin
- from table.models import Member
- # Register your models here.
- admin.site.register(Member)
- INSTALLED_APPS = [
- 'table',
- 'django.contrib.admin',
- 'django.contrib.auth',
- 'django.contrib.contenttypes',
- 'django.contrib.sessions',
- 'django.contrib.messages',
- 'django.contrib.staticfiles',
- ]
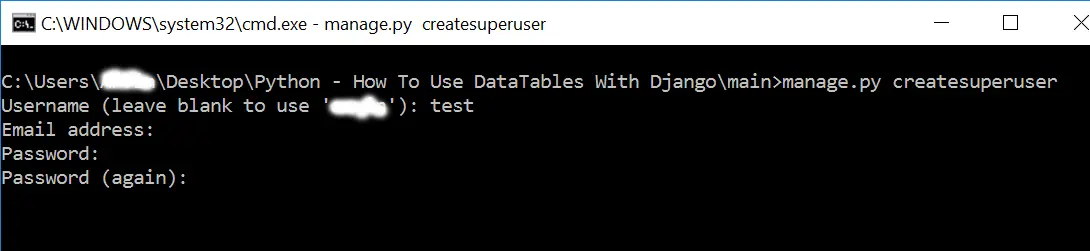

- <!DOCTYPE html>
- <html lang = "en">
- <head>
- <meta charset = "urf-8" name = "viewport" content = "width=device-width, initial-scale=1"/>
- {% load static %}
- <link rel = "stylesheet" type = "text/css" href = "{% static 'table/css/bootstrap.css' %}"/>
- <link rel = "stylesheet" type = "text/css" href = "{% static 'table/css/jquery.dataTables.css' %}"/>
- </head>
- <body>
- <nav class = "navbar navbar-default" >
- <div class = "container-fluid">
- </div>
- </nav>
- <div class = "col-md-6 well">
- <hr style = "border-top:1px dotted #000;"/>
- {% block body %}
- {% endblock %}
- </div>
- </body>
- <script type = "text/javascript">
- $(document).ready(function(){
- $('#table').DataTable();
- });
- </script>
- </html>
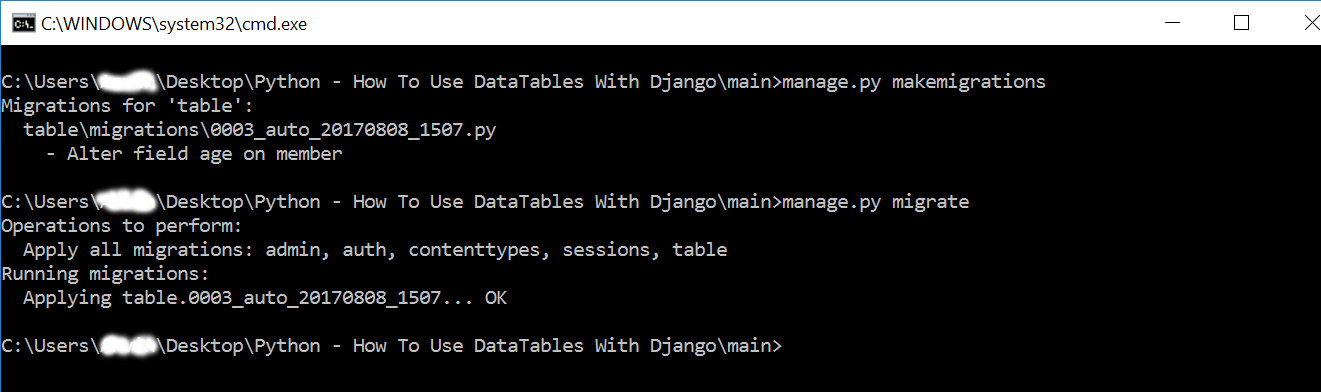
Add new comment
- 8192 views