PHP CRUD Operation using angular.js V2
Submitted by nurhodelta_17 on Wednesday, November 15, 2017 - 16:05.
Getting Started
Please take note that CSS and angular.js used in this tutorial are hosted so you need internet connection for them to work.Creating our Database
1. Open phpMyAdmin. 2. Click databases, create a database and name it as crud. 3. After creating a database, click the SQL and paste the below codes. See image below for detailed instruction.- CREATE TABLE `members` (
- `id` INT(10) UNSIGNED NOT NULL AUTO_INCREMENT,
- `firstname` VARCHAR(50) COLLATE utf8mb4_unicode_ci NOT NULL,
- `lastname` VARCHAR(50) COLLATE utf8mb4_unicode_ci NOT NULL,
- PRIMARY KEY(`id`)
- ) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4 COLLATE=utf8mb4_unicode_ci;
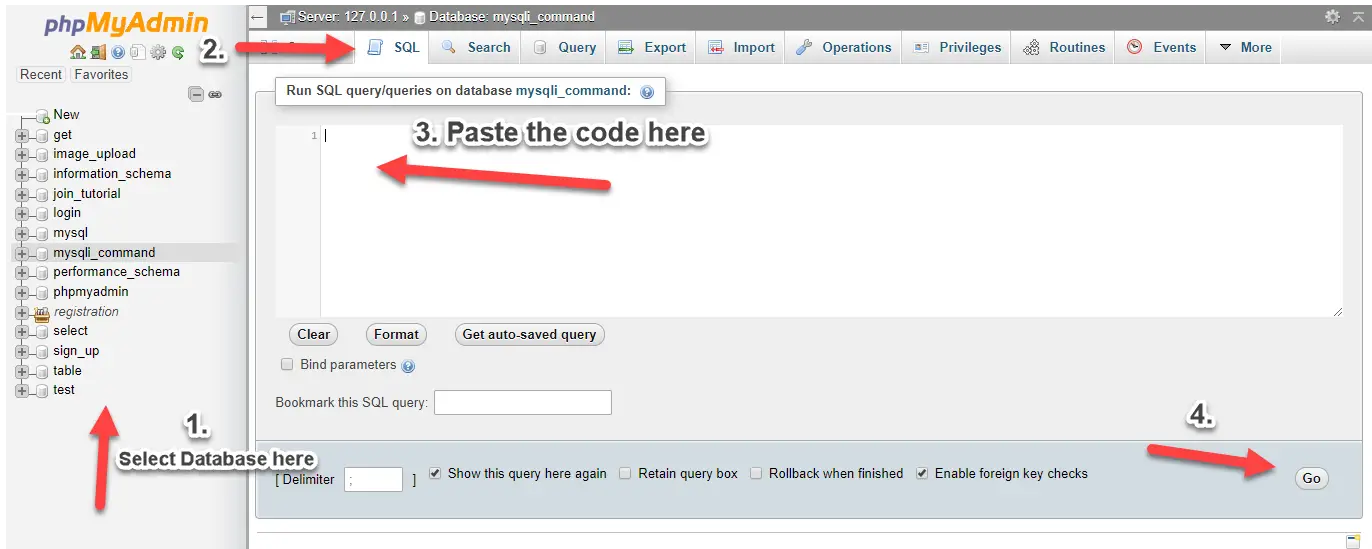
Creating our Connection
Next, we create our connection to our database. This will serve as the bridge between our forms and database. We name this as conn.php.- <?php
- $conn = new mysqli("localhost", "root", "", "crud");
- if ($conn->connect_error) {
- }
- ?>
index.php
This is our Read which contains our Member Table.- <!DOCTYPE html>
- <html ng-app="mem_app" ng-controller="controller" ng-init="showdata()">
- <head>
- <meta charset="UTF-8">
- <link href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css" rel="stylesheet">
- <link href="https://maxcdn.bootstrapcdn.com/font-awesome/4.7.0/css/font-awesome.min.css" rel="stylesheet">
- </head>
- <body>
- <div class="container">
- <div class="row">
- <div class="col-md-10 col-md-offset-1">
- <h2>Member List
- </h2>
- <table class="table table-bordered table-striped">
- <thead>
- </thead>
- <tbody>
- <tr ng-repeat="mem in member">
- <input type="hidden" value="{{mem.id}}">
- <td>
- </td>
- </tr>
- </tbody>
- </table>
- </div>
- </div>
- </div>
- <?php include('modal.php'); ?>
- </body>
- </html>
myangular.js
This is our angular script which contains all our angular functions.- var app = angular.module("mem_app", []);
- app.controller("controller", function($scope, $http) {
- $scope.action = function() {
- if ($scope.firstname == null) {
- alert("Please input Firstname");
- }
- else if ($scope.lastname == null) {
- alert("Please input Lastname");
- }
- else {
- $http.post(
- "action.php", {
- 'firstname': $scope.firstname,
- 'lastname': $scope.lastname,
- 'btnName': $scope.btnName,
- 'memberid': $scope.memberid,
- }
- ).success(function() {
- $scope.firstname = null;
- $scope.lastname = null;
- $scope.btnName = "Save";
- $scope.icon = "fa fa-save";
- $scope.btnClass = "btn btn-primary";
- $scope.showdata();
- });
- }
- }
- $scope.showdata = function() {
- $http.get("fetch.php")
- .success(function(data) {
- $scope.member = data;
- });
- }
- $scope.insert = function() {
- $scope.firstname = null;
- $scope.lastname = null;
- $scope.title = "Add New Member";
- $scope.btnName = "Save";
- $scope.icon = "fa fa-save";
- $scope.btnClass = "btn btn-primary";
- }
- $scope.update = function(memberid, firstname, lastname) {
- $scope.memberid = memberid;
- $scope.firstname = firstname;
- $scope.lastname = lastname;
- $scope.title = "Edit Member";
- $scope.icon = "fa fa-check-square-o";
- $scope.btnClass = "btn btn-success";
- $scope.btnName = "Update";
- }
- $scope.delmem = function(memberid) {
- $scope.delmember = memberid;
- }
- $scope.delete = function() {
- $http.post("delete.php", {
- 'memberid': $scope.delmember,
- }
- ).success(function(data) {
- $scope.showdata();
- });
- }
- $scope.enter = function(keyEvent) {
- if (keyEvent.which === 13){
- action();
- }
- }
- });
fetch.php
This is our PHP code in fetching our table data.- <?php
- include('conn.php');
- $query = $conn->query("select * from members");
- if ($query->num_rows > 0) {
- while ($row = $query->fetch_array()) {
- $output[] = $row;
- }
- }
- ?>
modal.php
This contains our 3 modals which are add modal, edit modal and delete modal.- <!-- Add/Edit Modal -->
- <div class="modal fade" id="addnew" tabindex="-1" role="dialog" aria-labelledby="myModalLabel">
- <div class="modal-dialog" role="document">
- <div class="modal-content">
- <div class="modal-header">
- </div>
- <div class="modal-body">
- <form ng-submit="myFunc()">
- <div class="form-group">
- <div class="row">
- <div class="col-md-2" style="margin-top:7px;">
- </div>
- <div class="col-md-10">
- <input type="text" class="form-control" ng-model="firstname" placeholder="Enter Firstname">
- </div>
- </div>
- </div>
- <div class="form-group">
- <div class="row">
- <div class="col-md-2" style="margin-top:7px;">
- </div>
- <div class="col-md-10">
- <input type="text" class="form-control" ng-model="lastname" placeholder="Enter Lastname">
- </div>
- </div>
- </div>
- </div>
- <div class="modal-footer">
- </form>
- </div>
- </div>
- </div>
- </div>
- <!-- Delete Modal -->
- <div class="modal fade" id="delete" tabindex="-1" role="dialog" aria-labelledby="myModalLabel">
- <div class="modal-dialog" role="document">
- <div class="modal-content">
- <div class="modal-header">
- </div>
- <div class="modal-body">
- <input type="hidden">
- </div>
- <div class="modal-footer">
- </div>
- </div>
- </div>
- </div>
action.php
This is our PHP code in adding and editing.- <?php
- include('conn.php');
- $btn_name = $data->btnName;
- if ($btn_name == "Save") {
- $conn->query("insert into members (firstname, lastname) values ('$firstname', '$lastname')");
- }
- if ($btn_name == "Update") {
- $memberid = $data->memberid;
- $conn->query("update members set firstname='$firstname', lastname='$lastname' where id='$memberid'");
- }
- }
- ?>
delete.php
Lastly, this is PHP delete code.- <?php
- include('conn.php');
- $id = $data->memberid;
- $conn->query("delete from members where id='$id'");
- ?>