PHP - OOP How to Create Page Views
Submitted by nurhodelta_17 on Monday, October 30, 2017 - 16:52.
This tutorial will teach you two ways in order to get the page view. First is a simple view that updates our database every load of the page and the other is creating another table for our views if ever you wanted to tally views per day/month/year.
Note: CSS and jQuery used in this tutorial are hosted so you need internet connection for them to work.
For page view with date, use this:
That ends this tutorial. If you have any question, comment, or suggestion, feel free to write it below or message me. Happy Coding :)
Creating our Database
1. Open phpMyAdmin. 2. Click databases, create a database and name it as pageview. 3. After creating a database, click the SQL and paste the below codes. See image below for detailed instruction. For simple page view, use this:- CREATE TABLE `animal` (
- `animalid` INT(11) NOT NULL AUTO_INCREMENT,
- `aname` VARCHAR(50) NOT NULL,
- `description` text NOT NULL,
- `photo` VARCHAR(150) NOT NULL,
- `views` INT(11) NOT NULL,
- PRIMARY KEY(animalid)
- ) ENGINE=InnoDB DEFAULT CHARSET=latin1;
- CREATE TABLE `animal` (
- `animalid` INT(11) NOT NULL AUTO_INCREMENT,
- `aname` VARCHAR(50) NOT NULL,
- `description` text NOT NULL,
- `photo` VARCHAR(150) NOT NULL,
- PRIMARY KEY(`animalid`)
- ) ENGINE=InnoDB DEFAULT CHARSET=latin1;
- CREATE TABLE `view` (
- `viewid` INT(11) NOT NULL AUTO_INCREMENT,
- `animalid` INT(11) NOT NULL,
- `date` DATE NOT NULL,
- PRIMARY KEY(`viewid`)
- ) ENGINE=InnoDB DEFAULT CHARSET=latin1;
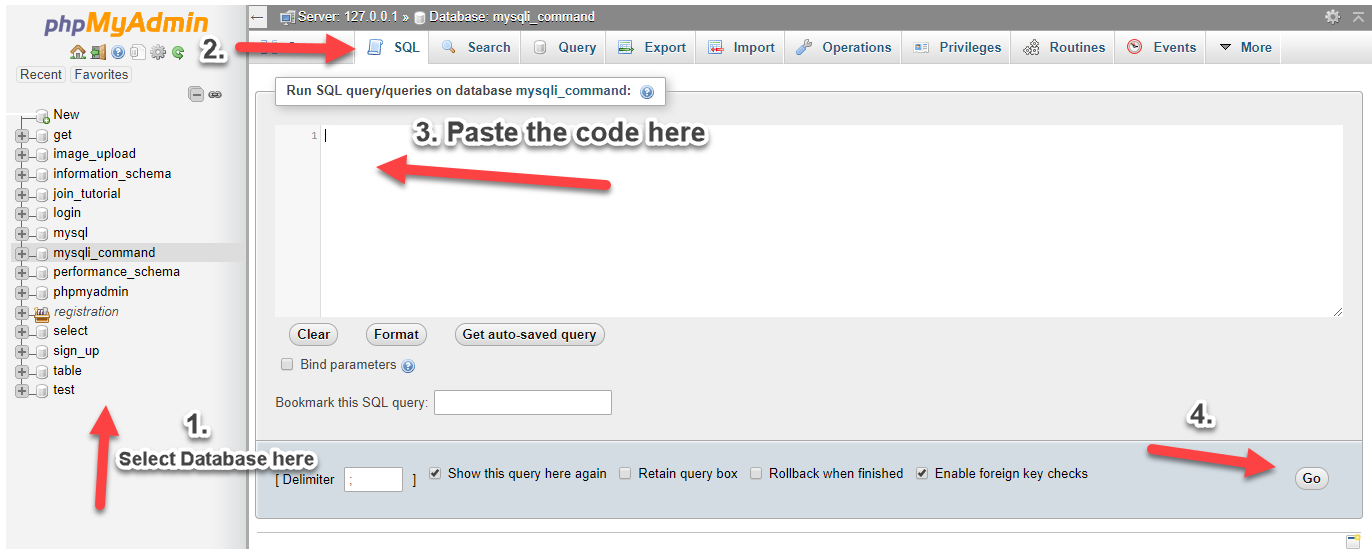
Inserting Sample Data
Next, we insert sample data for our page.- INSERT INTO `animal` (`animalid`, `aname`, `description`, `photo`, `views`) VALUES
- (1, 'Philippine Eagle', 'The Philippine eagle (Pithecophaga jefferyi), also known as the monkey-eating eagle or great Philippine eagle, is an eagle of the family Accipitridae endemic to forests in the Philippines. It has red coloured plumage, and a shaggy crest, and generally measures 86 to 102 cm (2.82 to 3.35 ft) in length and weighs 4.7 to 8.0 kg (10.4 to 17.6 lb). It is considered the largest of the extant eagles in the world in terms of length and wing surface, with Steller''s sea eagle and the harpy eagle being larger in terms of weight and bulk. Among the rarest and most powerful birds in the world, it has been declared the Philippine national bird. It is critically endangered, mainly due to massive loss of habitat resulting from deforestation in most of its range. Killing a Philippine eagle is punishable under Philippine law by 12 years in prison and heavy fines.', 'img/eagle.jpg', 1),
- (2, 'Tarsier', 'Tarsiers are any haplorrhine primates of the family Tarsiidae, which is itself the lone extant family within the infraorder Tarsiiformes. Although the group was once more widespread, all its species living today are found in the islands of Southeast Asia. On Bohol Island in the Philippines, tarsiers are locally known as mamag.', 'img/tarsier.jpg', 5),
- (3, 'Philippine Crocodile', 'The Philippine crocodile (Crocodylus mindorensis), also known as the Mindoro crocodile, the Philippine freshwater crocodile or locally, bukarot, is one of two species of crocodiles found in the Philippines; the other is the larger Indo-Pacific crocodile or saltwater crocodile (Crocodylus porosus). The Philippine crocodile, the species endemic only to the country, became data deficient to critically endangered in 2008 from exploitation and unsustainable fishing methods, such as dynamite fishing. Conservation methods are being taken by the Dutch/Filipino Mabuwaya foundation, the Crocodile Conservation Society and the Zoological Institute of HerpaWorld in Mindoro island. It is strictly prohibited to kill a crocodile in the country, and it is punishable by law.', 'img/crocodile.jpg', 1),
- (4, 'Tamaraw', 'The tamaraw or Mindoro dwarf buffalo (Bubalus mindorensis) is a small hoofed mammal belonging to the family Bovidae. It is endemic to the island of Mindoro in the Philippines, and is the only endemic Philippine bovine. It is believed, however, to have once also thrived on the larger island of Luzon. The tamaraw was originally found all over Mindoro, from sea level up to the mountains (2000 meters above sea level), but because of human habitation, hunting, and logging, it is now restricted to only a few remote grassy plains and is now an endangered species.\r\n\r\nContrary to common belief and past classification, the tamaraw is not a subspecies of the local carabao, which is only slightly larger, or the common water buffalo. In contrast to the carabao, it has a number of distinguishing characteristics: it is slightly hairier, has light markings on its face, is not gregarious, and has shorter horns that are somewhat V-shaped. It is the largest native terrestrial mammal in the country.', 'img/tamaraw.jpg', 15),
- (5, 'Visayan Warty Pig', 'The Visayan warty pig (Sus cebifrons) is a critically endangered species in the pig genus (Sus). It is known by many names in the region (depending on the island and linguistic group) with most translating into ''wild pig'': baboy damo (literally, ''bush pig'' in Tagalog), baboy ilahas (''wild pig'' in Cebuano and Hiligaynon), baboy talonon (''untamed pig'' in Hiligaynon), bakatin (''small pig'' in Cebuano), baboy sulop (''dark pig'' in Cebuano), manggalisak banban (''half grown male'' in Cebuano), and biggal (''sow'' in Cebuano). The Visayan warty pig is endemic to two of the Visayan Islands in the central Philippines, and is threatened by habitat loss, food shortages, and hunting these are the leading causes of the Visayan warty pig''s status as critically endangered. Due to the small numbers of remaining Visayan warty pigs in the wild, little is known of their behaviors or characteristics outside of captivity.', 'img/pig.jpg', 3),
- (6, 'Red-vented Cockatoo', 'The red-vented cockatoo (Cacatua haematuropygia) sometimes called the Philippine cockatoo or kalangay, is a critically endangered species of cockatoo that is endemic to the Philippines. It is roughly the size and shape of the Tanimbar corella, but is easily distinguished by the red feathers around the vent.', 'img/cockatoo.jpg', 2);
Creating our Connection
Next, we create our connection to our database. This will serve as the bridge between our forms and database. We name this as conn.php.- <?php
- $conn = new mysqli("localhost", "root", "", "pageview");
- if ($conn->connect_error) {
- }
- ?>
header.php
This is our header file for templating. This contains our hosted CSS and jQuery.- <!DOCTYPE html>
- <html>
- <head>
- <title>PHP - OOP Page Views</title>
- <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.1.0/jquery.min.js"></script>
- <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.6/css/bootstrap.min.css" />
- <script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/js/bootstrap.min.js"></script>
- </head>
index.php
This contains our sample data that we can click to view our page.- <?php include('header.php'); ?>
- <body>
- <div class="container">
- <h1 class="page-header">Gallery</h1>
- <?php
- include('conn.php');
- $inc=3;
- $query=$conn->query("select * from animal");
- while($row=$query->fetch_array()){
- $inc = ($inc == 3) ? 1 : $inc+1;
- if($inc == 1) echo '<div class="row">';
- ?>
- <div class="col-md-4">
- <img src="<?php echo $row['photo']; ?>" class="thumbail" style="height:300px; width:100%;">
- <h2><center><a href="page.php?id=<?php echo $row['animalid']; ?>"><?php echo $row['aname']; ?></a></center></h2>
- </div>
- <?php
- if($inc == 3) echo '</div><br>';
- }
- if($inc == 1) echo '<div class="col-md-4"></div><div class="col-md-4"></div></div><br>';
- if($inc == 2) echo '<div class="col-md-4"></div></div><br>';
- ?>
- </div>
- </body>
- </html>
page.php
Lastly, this is our page where we put our number of views. I've included the codes in the comment for the 2 ways that I have mentioned above.- <?php include('header.php'); ?>
- <?php
- include('conn.php');
- $id=$_GET['id'];
- //for a simple view
- $conn->query("update animal set views=views + 1 where animalid='$id'");
- $query=$conn->query("select * from animal where animalid='$id'");
- $row=$query->fetch_array();
- $views=$row['views'];
- //if you want to have a date that you can use later
- //$conn->query("insert into view (animalid, date) values ('$id', NOW())");
- //$query=$conn->query("select * from view left join animal on animal.animalid=view.animalid where view.animalid='$id'");
- //$row=$query->fetch_array();
- //$views=$query->num_rows;
- ?>
- <body>
- <div class="container">
- <h1 class="page-header"><?php echo $row['aname']; ?>
- <span class="pull-right" style="font-size:15px; margin-top:20px;">Page Views: <strong><?php echo $views; ?></strong></span>
- </h1>
- <div class="row">
- <div class="col-md-3">
- <img src="<?php echo $row['photo'] ?>" style="width:100%; height:230px;">
- </div>
- <div class="col-md-9">
- <p><?php echo $row['description']; ?></p>
- </div>
- </div>
- </div>
- </body>
- </html>