How to use SQL Limit to Limit Results in PHP/MySQLi
Submitted by nurhodelta_17 on Tuesday, October 10, 2017 - 21:12.
The use of limit limits the number of results of your MySQL Query and is usually at the last part of your SQL. This is most applicable if for example, you want to get the top selling product, you just need to order the result then limit it by 1. This tutorial will teach you how to use this limit.
Note: Bootstrap CSS and script used in this tutorial are hosted, therefore, you need internet connection for them to work.
That ends this tutorial. If you have any questions or comments, feel free to write it below or message me. Happy Coding :)
Creating our Database
First step is to create our database. 1. Open phpMyAdmin. 2. Click databases, create a database and name it as testing. 3. After creating a database, click the SQL and paste the below codes. See image below for detailed instruction.- CREATE TABLE `tutorial` (
- `tutorialid` INT(11) NOT NULL AUTO_INCREMENT,
- `title` VARCHAR(150) NOT NULL,
- `link` VARCHAR(150) NOT NULL,
- PRIMARY KEY(`tutorialid`)
- ) ENGINE=InnoDB DEFAULT CHARSET=latin1;
- INSERT INTO `tutorial` (`tutorialid`, `title`, `link`) VALUES
- (1, 'Submit Form using AJAX in PHP/MySQLi', 'https://www.sourcecodester.com/tutorials/php/11649/submit-form-using-ajax-phpmysqli.html'),
- (2, 'Image Upload using AJAX in PHP/MySQLi', 'https://www.sourcecodester.com/tutorials/php/11648/image-upload-using-ajax-phpmysqli.html'),
- (3, 'Creating an Iterator for While Loop Forms in PHP/MySQLi', 'https://www.sourcecodester.com/php/11643/creating-iterator-while-loop-forms-phpmysqli.html'),
- (4, 'Drag, Drop and Insert into Database using AJAX/jQuery in PHP', 'https://www.sourcecodester.com/tutorials/php/11641/drag-drop-and-insert-database-using-ajaxjquery-php.html'),
- (5, 'Simple POS and Inventory System', 'https://www.sourcecodester.com/php/11625/simple-pos-and-inventory-system.html'),
- (6, 'Performance Indicator System', 'https://www.sourcecodester.com/php/11638/performance-indicator-system.html'),
- (7, 'Simple Chat System', 'https://www.sourcecodester.com/php/11610/simple-chat-system.html'),
- (8, 'Uploading Multiple Files into MySQL Database using PHP/MySQLi', 'https://www.sourcecodester.com/tutorials/php/11634/uploading-multiple-files-mysql-database-using-phpmysqli.html'),
- (9, 'Deleting Multiple Rows using Checkbox in PHP/MySQLi', 'https://www.sourcecodester.com/tutorials/php/11631/deleting-multiple-rows-using-checkbox-phpmysqli.html'),
- (10, 'PHP/MySQLi CRUD Operation with Bootstrap/Modal', 'https://www.sourcecodester.com/php/11629/phpmysqli-crud-operation-bootstrapmodal.html'),
- (11, 'PHP Passing Value to Modal using jQuery', 'https://www.sourcecodester.com/tutorials/php/11627/php-passing-value-modal-using-jquery.html'),
- (12, 'How to Add Class to a Div using JQuery', 'https://www.sourcecodester.com/tutorials/javascript/11619/how-add-class-div-using-jquery.html'),
- (13, 'How to Limit Number of Items per Row in While Loop using PHP/MySQLi', 'https://www.sourcecodester.com/tutorials/php/11618/how-limit-number-items-row-while-loop-using-phpmysqli.html'),
- (14, 'PHP Prevent the Return to Login Page/Disable Back after Login', 'https://www.sourcecodester.com/tutorials/php/11614/php-prevent-return-login-pagedisable-back-after-login.html');
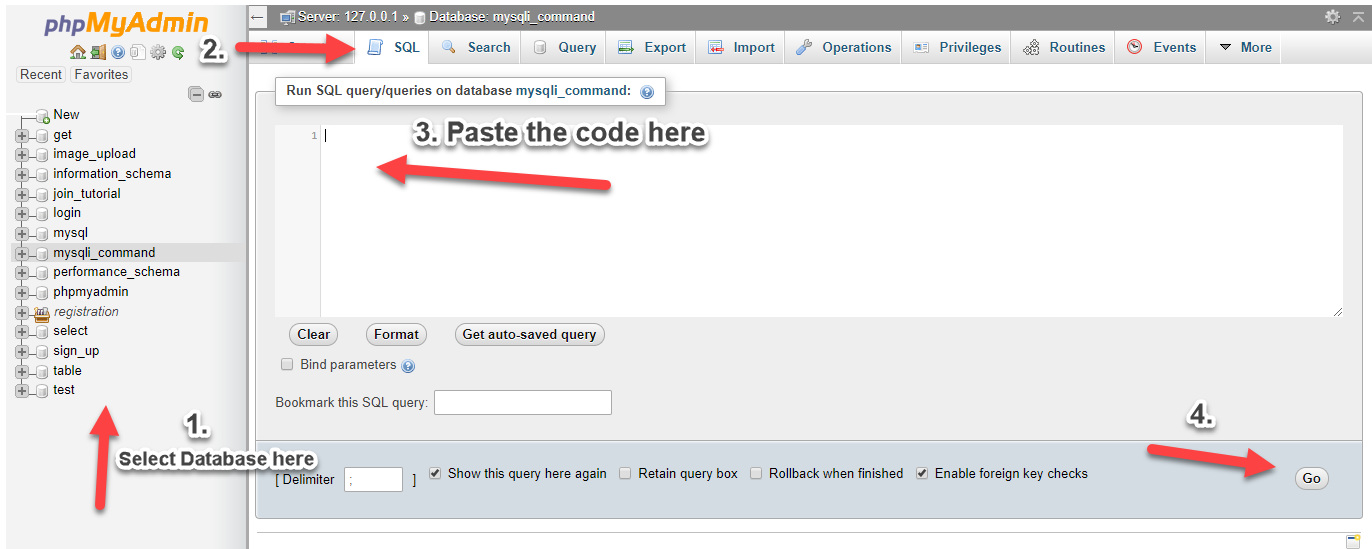
Creating our Connection
Next, we create our connection to our database. This will serve as the bridge between our forms and database. We name this as conn.php.- <?php
- if (!$conn) {
- }
- ?>
index.php
And this contains our sample table and a limit form so you can practice limiting our sample table.- <!DOCTYPE html>
- <html>
- <head>
- <title>How to use SQL Limit to Limit Results in PHP/MySQLi</title>
- <script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.6/js/bootstrap.min.js"></script>
- <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.6/css/bootstrap.min.css">
- </head>
- <body>
- <div class="container">
- <div class="row" style="margin-top:20px;">
- <center><span style="font-size:25px; color:blue"><strong>How to use SQL Limit to Limit Results in PHP/MySQLi</strong></span></center>
- <form method="POST" class="form-inline" style="margin-top:10px;">
- <input type="text" class="form-control" name="limit">
- <input type="submit" name="limitme" value="Limit Query" class="btn btn-primary">
- <input type="submit" name="all" value="Show All" class="btn btn-success">
- </form>
- </div>
- <div class="row"style="margin-top:20px;">
- <h4><a href="https://www.sourcecodester.com/user/224918/track" style="text-decoration:none;">My Tutorials</a></h4>
- <table class="table table-bordered table-striped">
- <thead>
- <th>ID</th>
- <th>Title</th>
- <th>Link</th>
- </thead>
- <tbody>
- <?php
- include('conn.php');
- $limit="";
- $number=$_POST['limit'];
- $limit='limit '.$number;
- }
- $limit='';
- }
- ?>
- <tr>
- <td><?php echo $row['tutorialid']; ?></td>
- <td><?php echo $row['title']; ?></td>
- <td><?php echo $row['link']; ?></td>
- </tr>
- <?php
- }
- ?>
- </tbody>
- </table>
- </div>
- </div>
- </body>
- </html>