How to Pass a PHP Value to a Modal using jQuery
This is a PHP tutorial on how to pass a value to a bootstrap modal using jQuery. This is most applicable if you want to use jQuery when you edit your MySQL Table using Bootstrap Modal.
Creating our Database
The first step is to create our database.
- Open phpMyAdmin.
- Click databases, create a database and name it as "
sample
". - After creating a database, click the SQL and paste the below code. See the image below for detailed instructions.
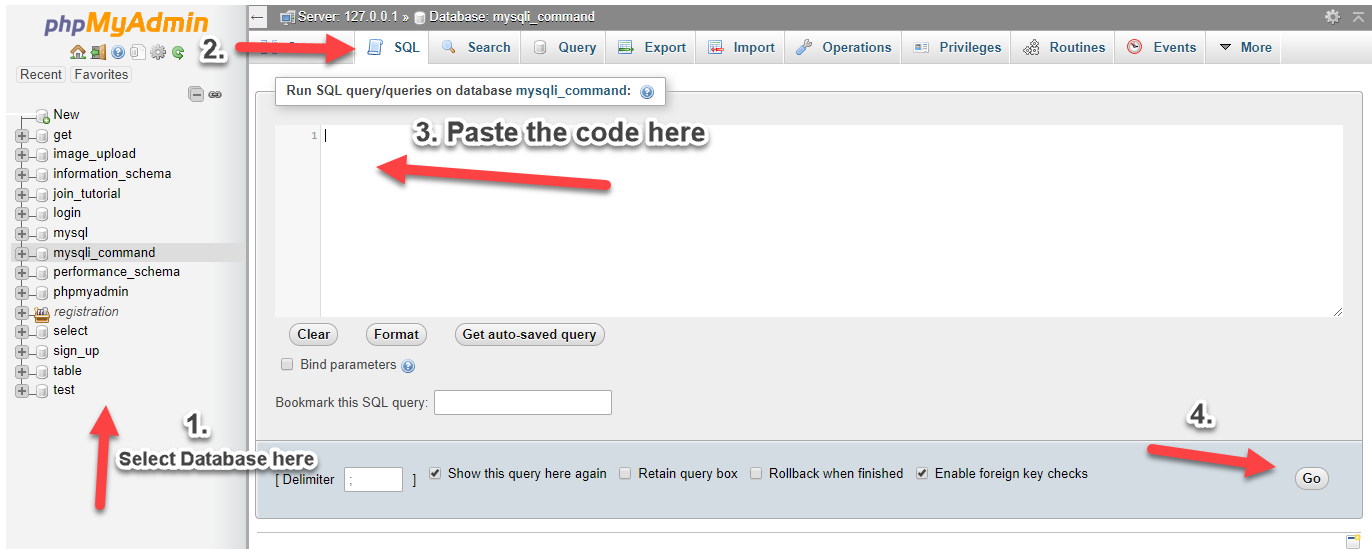
Inserting Data into our Database
The next step is to insert sample data into our database.
- Click the "
sample
" database that we have created. - Click SQL and paste the code below.
Creating our Table
Next, we create our sample table. We name this as index.php
.
- <!DOCTYPE html>
- <html lang="en">
- <head>
- <meta charset="utf-8">
- <meta http-equiv="X-UA-Compatible" content="IE=edge">
- <meta name="viewport" content="width=device-width, initial-scale=1">
- <meta name="description" content="">
- <meta name="author" content="">
- <title>PHP Passing Value to Modal using jQuery</title>
- <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.1.0/jquery.min.js"></script>
- <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.6/css/bootstrap.min.css" />
- <script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/js/bootstrap.min.js"></script>
- </head>
- <body>
- <div class="container">
- <div style="height:50px;"></div>
- <div class="well">
- <center><h2>Passing Values to Modal using jQuery</h2></center>
- <div style="height:10px;"></div>
- <table width="100%" class="table table-striped table-bordered table-hover">
- <thead>
- <th>Firstname</th>
- <th>Lastname</th>
- <th>Address</th>
- <th>Action</th>
- </thead>
- <tbody>
- <?php
- ?>
- <tr>
- <td><span id="firstname<?php echo $row['userid']; ?>"><?php echo $row['firstname']; ?></span></td>
- <td><span id="lastname<?php echo $row['userid']; ?>"><?php echo $row['lastname']; ?></span></td>
- <td><span id="address<?php echo $row['userid']; ?>"><?php echo $row['address']; ?></span></td>
- <td><button type="button" class="btn btn-success edit" value="<?php echo $row['userid']; ?>"><span class="glyphicon glyphicon-edit"></span> Edit</button></td>
- </tr>
- <?php
- }
- ?>
- </tbody>
- </table>
- </div>
- <?php include('modal.php'); ?>
- <script src="custom.js"></script>
- </body>
- </html>
Creating our Modal
Next is to create our modal. We name this asmodal.php
.
- <div class="modal fade" id="edit" tabindex="-1" role="dialog" aria-labelledby="myModalLabel" aria-hidden="true">
- <div class="modal-dialog">
- <div class="modal-content">
- <div class="modal-header">
- </div>
- <div class="modal-body">
- <div class="container-fluid">
- <div class="form-group input-group">
- <input type="text" style="width:350px;" class="form-control" id="efirstname">
- </div>
- <div class="form-group input-group">
- <input type="text" style="width:350px;" class="form-control" id="elastname">
- </div>
- <div class="form-group input-group">
- <input type="text" style="width:350px;" class="form-control" id="eaddress">
- </div>
- </div>
- </div>
- <div class="modal-footer">
- </div>
- </div>
- </div>
- </div>
Creating our Script
The last step is to create our script to pass a value to our modal. We name this as custom.js
.
- $(document).ready(function(){
- $(document).on('click', '.edit', function(){
- var id=$(this).val();
- var first=$('#firstname'+id).text();
- var last=$('#lastname'+id).text();
- var address=$('#address'+id).text();
- $('#edit').modal('show');
- $('#efirstname').val(first);
- $('#elastname').val(last);
- $('#eaddress').val(address);
- });
- });
That ends our tutorial. Note that I have only used CDN's in this tutorial to include the Bootstrap and jQuery Libraries which means you need an internet connection to test the source code in this tutorial. Download the the Bootstrap library here and jQuery here to run the source code even without any internet connection.
DEMOI hope this will help you and you have learned something useful with this tutorial. Explore more on this website for more tutorials and free source codes.
Happy Coding :)