Delete Data Using Date in PHP Using PDO
Submitted by alpha_luna on Monday, June 6, 2016 - 13:27.
If you are looking for Auto Delete Data Using Current Date then you are at the right place. This source code will help you on how to create a simple program that the data will automatically delete when the expiration date will appear. To set the expiration date, we have one input field to add a number of days to the current date and this step to get the expiration date to delete the data automatically. Let's start with:
This is the result of the code above:
And, that's it. This is the steps on how to delete data automatically using a date in PHP using PDO.
Kindly click the "Download Code" button below for full source code. Thank you very much.
Share us your thoughts and comments below. Thank you so much for dropping by and reading this tutorial post. For more updates, don’t hesitate and feel free to visit this website more often and please share this with your friends or email me at [email protected]. Practice Coding. Thank you very much.
Creating our Table
We are going to make our database.- Open the PHPMyAdmin.
- Create a database and name it as "delete_by_date".
- After creating a database name, then we are going to create our table. And name it as "tbl_member".
- Kindly copy the code below.
- CREATE TABLE `tbl_member` (
- `tbl_member_id` INT(11) NOT NULL,
- `first_name` VARCHAR(100) NOT NULL,
- `last_name` VARCHAR(100) NOT NULL,
- `contact_number` VARCHAR(100) NOT NULL,
- `email` VARCHAR(100) NOT NULL,
- `address` VARCHAR(100) NOT NULL,
- `expiration_date` VARCHAR(100) NOT NULL
- ) ENGINE=InnoDB DEFAULT CHARSET=latin1;
Creating Database Connection
- <?php
- $conn = new PDO('mysql:host=localhost; dbname=delete_by_date','root', '');
- ?>
Creating Form Field
This form field where you enter the information or data and to set the expiration date to delete the data automatically in the database table.- <form method="post" action="add_person_query.php">
- <table border="1" cellspacing="5" cellpadding="5" width="100%">
- <tr>
- <td>
- </td>
- <td width="395px">
- <input type="text" class="text_Box" name="first_name" autofocus="autofocus" placeholder="First Name ....." required />
- </td>
- </tr>
- <tr>
- <td>
- </td>
- <td width="395px">
- <input type="text" class="text_Box" name="last_name" placeholder="Last Name ....." required />
- </td>
- </tr>
- <tr>
- <td>
- </td>
- <td width="395px">
- <input type="text" class="text_Box" name="contact_number" placeholder="Contact Number ....." required />
- </td>
- </tr>
- <tr>
- <td>
- </td>
- <td width="395px">
- <input type="email" class="text_Box" name="email" placeholder="Email .....">
- </td>
- </tr>
- <tr>
- <td>
- </td>
- <td width="395px">
- <input type="text" class="text_Box" name="address" placeholder="Address ....." required />
- </td>
- </tr>
- <tr>
- <td>
- </td>
- <td width="395px">
- <input type="text" class="text_Box" name="number_of_days" placeholder="Number of Days ....." required />
- </td>
- </tr>
- <tr>
- <td colspan="2">
- <a>
- <button type="submit" class="btn_confirm">
- Save Data
- </button>
- </a>
- </td>
- </tr>
- </table>
- </form>
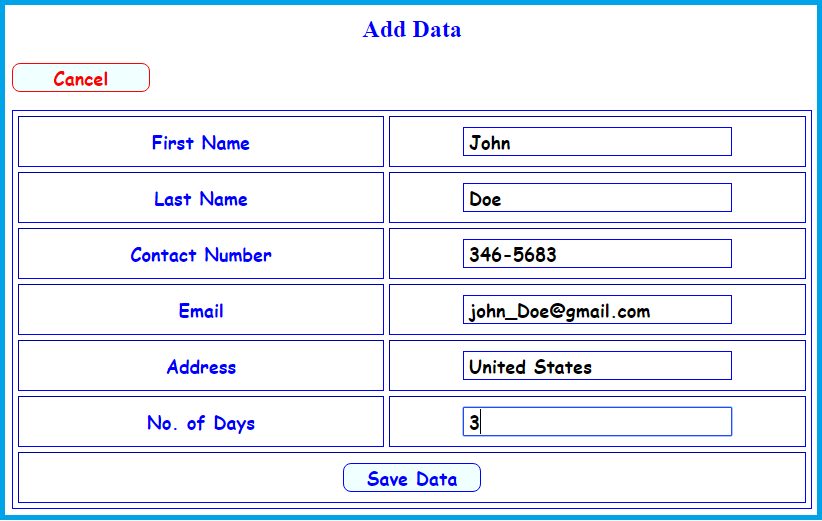
Creating INSERT Statement Using PDO
This PHP script is used to save our data in the database table.- <?php
- include ('db.php');
- $first_name = $_POST['first_name'];
- $last_name = $_POST['last_name'];
- $contact_number = $_POST['contact_number'];
- $email = $_POST['email'];
- $address = $_POST['address'];
- $number_of_days = $_POST['number_of_days'];
- $conn->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);
- $sql = "INSERT INTO tbl_member (first_name, last_name, contact_number, email, address, expiration_date)
- VALUES ('$first_name', '$last_name', '$contact_number', '$email', '$address', '$expiration_date')";
- echo "<script>alert('Successfully Added!'); window.location='index.php'</script>";
- ?>
Creating DELETE Statement
This PHP script for DELETE Statement is used to delete the data in the database table and we are going to use the set of expiration date and it will automatically delete when the expiration date will appear.This our example data in the database table:
As you can see in the box, that's the expiration date of every data in the database table. It will delete automatically if the expiration date will appear.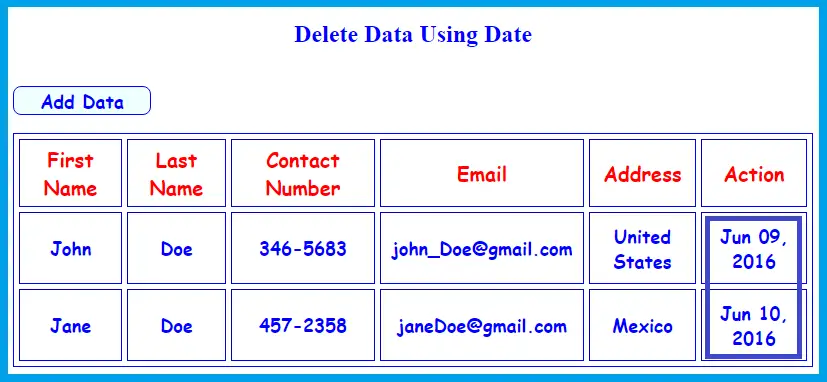