AngularJS Signup/Register using PHP/MySQLi
Submitted by nurhodelta_17 on Sunday, January 7, 2018 - 17:47.
Getting Started
I've used CDN for Bootstrap, Angular JS and Angular Route so you need internet connection for them to work. This tutorial is a continuation of the previous tutorial, Angular JS Login with Session using PHP/MySQLi, which discuss on how to create a login page. We're gonna be using the files we created in the previous tutorial.Creating our Database
First, we're going to create our database. 1. Open phpMyAdmin. 2. Click databases, create a database and name it as angular. 3. After creating a database, click the SQL and paste the below codes. See image below for detailed instruction.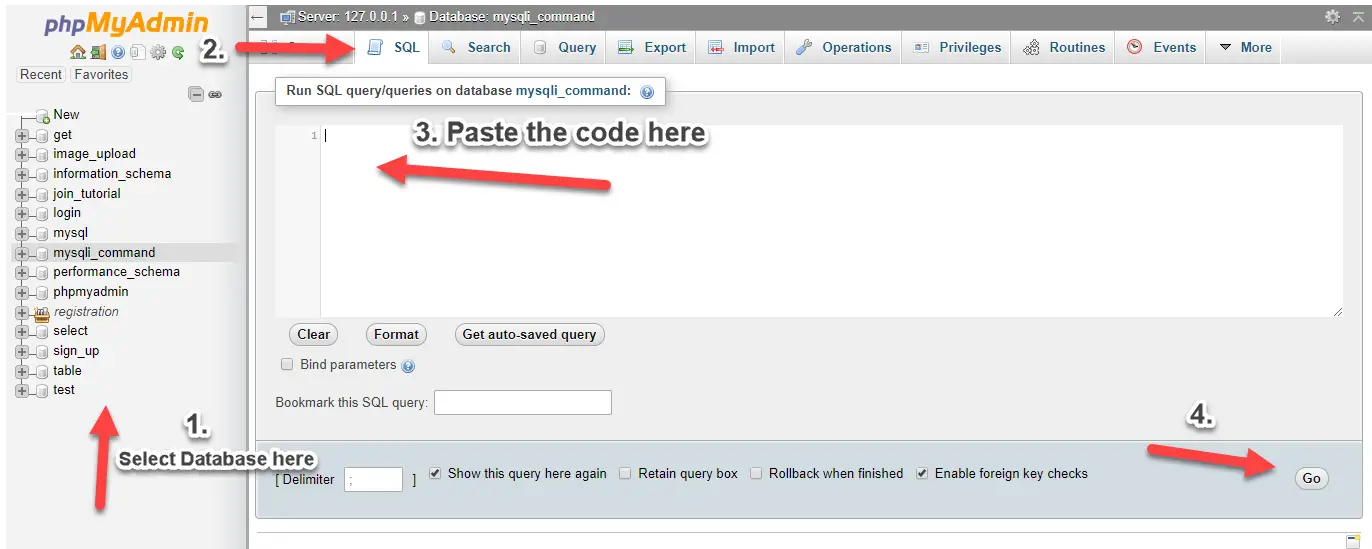
Updating our Main Page
Next, we update index.html by adding the ff. javascript files for our registration.- <script src="js/controllers/signupCtrl.js"></script>
- <script src="js/services/signupService.js"></script>
Creating the link for Signup
Next, in the login.html, in the panel heading, add this to have a link to our registration form.Updating our Main Angular JS Script
Next, we update angular.js to include our signup form.- var app = angular.module('app', ['ngRoute']);
- app.config(function($routeProvider){
- $routeProvider
- .when('/', {
- templateUrl: 'login.html',
- controller: 'loginCtrl'
- })
- .when('/signup', {
- templateUrl: 'signup.html',
- controller: 'signupCtrl'
- })
- .when('/home', {
- templateUrl: 'home.html',
- controller: 'homeCtrl'
- })
- .otherwise({
- redirectTo: '/'
- });
- });
- app.run(function($rootScope, $location, loginService){
- //prevent going to homepage if not loggedin
- var routePermit = ['/home'];
- $rootScope.$on('$routeChangeStart', function(){
- if(routePermit.indexOf($location.path()) !=-1){
- var connected = loginService.islogged();
- connected.then(function(response){
- if(!response.data){
- $location.path('/');
- }
- });
- }
- });
- //prevent going back to login page if sessino is set
- var sessionStarted = ['/'];
- $rootScope.$on('$routeChangeStart', function(){
- if(sessionStarted.indexOf($location.path()) !=-1){
- var cantgoback = loginService.islogged();
- cantgoback.then(function(response){
- if(response.data){
- $location.path('/home');
- }
- });
- }
- });
- //prevent going back to singup page
- var signupcompleted = ['/signup'];
- $rootScope.$on('$routeChangeStart', function(){
- if(signupcompleted.indexOf($location.path()) !=-1){
- var signupsuccess = loginService.islogged();
- signupsuccess.then(function(response){
- if(response.data){
- $location.path('/home');
- }
- });
- }
- });
- });
Creating our Signup Form
Next, we create our sign up form and we're gonna name it as signup.html.- <div class="col-md-4 col-md-offset-4">
- <div class="login-panel panel panel-primary">
- <div class="panel-heading">
- </h3>
- </div>
- <div class="panel-body">
- <form role="form" name="signupform">
- <fieldset>
- <div class="form-group">
- <input class="form-control" placeholder="Username" type="text" autofocus ng-model="user.username" required>
- </div>
- <div class="form-group">
- <input class="form-control" placeholder="Password" type="password" ng-model="user.password" required>
- </div>
- <div class="form-group">
- <input class="form-control" placeholder="Firstname" type="text" ng-model="user.firstname" required>
- </div>
- <div class="form-group">
- <input class="form-control" placeholder="Lastname" type="text" ng-model="user.lastname" required>
- </div>
- <div class="form-group">
- <input class="form-control" placeholder="Address" type="text" ng-model="user.address" required>
- </div>
- </fieldset>
- </form>
- </div>
- </div>
- <div class="alert alert-danger text-center" ng-show="errorSignup">
- {{ errorMsg }}
- </div>
- <div class="alert alert-success text-center" ng-show="successSignup">
- {{ successMsg }}
- </div>
- </div>
Creating our Signup Controller
Next step is to create the controller for our sign up and we're gonna name it as signupCtrl.js.- 'use strict';
- app.controller('signupCtrl', function($scope, signupService){
- $scope.errorSignup = false;
- $scope.successSignup = false;
- $scope.signup = function(user){
- signupService.signup(user, $scope);
- }
- $scope.clearMsg = function(){
- $scope.errorSignup = false;
- $scope.successSignup = false;
- }
- });
Creating our Signup Service
Next, we create our sign up service and we're gonna name it as signupService.js- 'use strict';
- app.factory('signupService', function($http, $location, sessionService){
- return{
- signup: function(user, $scope){
- var validate = $http.post('signup.php', user);
- validate.then(function(response){
- //console.log(response);
- var uid = response.data.user;
- if(uid){
- sessionService.set('user',uid);
- $location.path('/home');
- }
- else{
- $scope.successLogin = false;
- $scope.errorLogin = true;
- $scope.errorMsg = response.data.message;
- }
- });
- }
- }
- });
Creating our Signup API
Lastly, this is our Signup PHP code/api to insert user in our database.- <?php
- $conn = new mysqli("localhost", "root", "", "angular");
- if ($conn->connect_error) {
- }
- $username = $user->username;
- $password = $user->password;
- $firstname = $user->firstname;
- $lastname = $user->lastname;
- $address = $user->address;
- $sql = "INSERT INTO members (username, password, firstname, lastname, address) VALUES ('$username', '$password', '$firstname', '$lastname', '$address')";
- $query = $conn->query($sql);
- if($query){
- $nid = $conn->insert_id;
- $_SESSION['user'] = $nid;
- }
- else{
- $out['error'] = true;
- $out['message'] = 'Signup Failed';
- }
- ?>