Caret Event and Listener in Java
Submitted by donbermoy on Tuesday, December 23, 2014 - 12:48.
This tutorial will teach you how to create a program that will have an example of Caret Event and Listener in Java. A CaretEvent lets the user notify interested parties that the text caret has changed in the event with its source. Note: A caret is the cursor indicating the insertion point
So, now let's start this tutorial!
1. Open JCreator or NetBeans and make a java program with a file name of caretListener.java.
2. Import the swing package library:
3. We will initialize variables in our Main, variable frame as JFrame and txtArea as JTextArea.
4. Now, we will add the CaretListener to the txtArea.
As what have you seen in the code above, a caret changes its position or if text is selected, a caret event is fired by the textArea. The class CaretEvent supports the methods getDot() and getMark() to retrieve the current location and the end position of a text selection. And it will display the console the dot and the mark position of the text.
5. Lastly, add the txtArea with its JScrollPane component, set the size, visibility, and the close operation of the frame. Have this code below:
Output:
Here's the full code of this tutorial:
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below.
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
- import javax.swing.*; //used to access the JFrame,JScrollPane, and JTextArea
- import javax.swing.event.*; //used to access CaretEvent and CaretListener
- }
- });
- frame.setSize(300, 200);
- frame.setVisible(true);
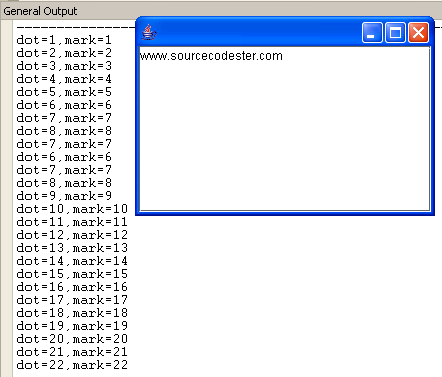
- import javax.swing.*; //used to access the JFrame,JScrollPane, and JTextArea
- import javax.swing.event.*; //used to access CaretEvent and CaretListener
- public class caretListener {
- }
- });
- frame.setSize(300, 200);
- frame.setVisible(true);
- }
- }