SpringLayout as Layout Manager in Java
Submitted by donbermoy on Thursday, December 4, 2014 - 23:05.
This tutorial is about the SpringLayout as Layout Manager in Java. A SpringLayout is a layout by defining constraints betweem the edges of the components. This attaches springs to other components taht lays out the children of its associated container according to a set of constraints.
So, now let's start this tutorial!
1. Open JCreator or NetBeans and make a java program with a file name of springLayout.java.
2. Import javax.swing.* package library to access JFrame, JLabel, JTextField, and SpringLayout class.
3. Initialize your variables of the components in your Main.
We have created two JTextFields named textField1 and textField2 and two JLabels named label1 and label2. We initialize the SpringLayout named layout variable.
4. Make your layout to SpringLayout using the setLayout method.
Now, add the components to the frame using the add method.
5. Now, put a constraint to the variable layout of SpringLayout where you want to display your components.
The first putConstraint in label1 specifies that the label's left west position will be 10 in the x position from the frame's left edge. The second putConstraint call sets up to the north position of 10 initiating the y coordinate.
The label2 sets up its constraint to 10 as x-coordinate and 30 as the y-coordinate.
The first putConstraint in textField1 specifies that the label's left west position will be added to the 10 as x-coordinate. The second putConstraint call sets up to the north position of 30 initiating the y coordinate.
6. Lastly, set its size and visibility, and its close operation. Have this code below:
Output:
Here's the full code of this tutorial:
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below.
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
- import javax.swing.*; //used to access JFrame, JLabel, JTextField, and SpringLayout class
- SpringLayout layout = new SpringLayout();
- frame.getContentPane().setLayout(layout);
- frame.getContentPane().add(label1);
- frame.getContentPane().add(textField1);
- frame.getContentPane().add(label2);
- frame.getContentPane().add(textField2);
- // Adjust constraints for the label1 so it's at (10,10).
- layout.putConstraint(SpringLayout.WEST, label1, 10, SpringLayout.WEST, frame.getContentPane());
- layout.putConstraint(SpringLayout.NORTH, label1, 10, SpringLayout.NORTH, frame.getContentPane());
- // Adjust constraints for the label2 so it's at (10,30).
- layout.putConstraint(SpringLayout.WEST, label2, 10, SpringLayout.WEST,frame.getContentPane());
- layout.putConstraint(SpringLayout.NORTH, label2, 30, SpringLayout.NORTH, frame.getContentPane());
- // Adjust constraints for the text field so it's at
- // (<label1's right edge> + 10, 10).
- layout.putConstraint(SpringLayout.WEST, textField1, 10, SpringLayout.EAST, label1);
- layout.putConstraint(SpringLayout.NORTH, textField1, 10, SpringLayout.NORTH, frame.getContentPane());
- // Adjust constraints for the text field so it's at
- // (<label2's right edge> + 10, 30).
- layout.putConstraint(SpringLayout.WEST, textField2, 10, SpringLayout.EAST, label2);
- layout.putConstraint(SpringLayout.NORTH, textField2, 30, SpringLayout.NORTH, frame.getContentPane());
- // Display the window.
- frame.setSize(400,300);
- frame.setVisible(true);
- //close operation
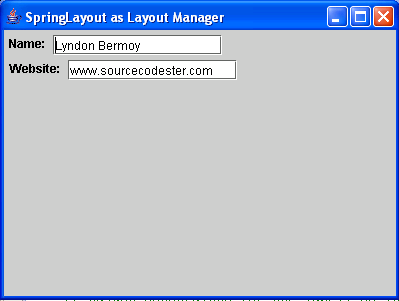
- import javax.swing.*; //used to access JFrame, JLabel, JTextField, and SpringLayout class
- public class springLayout {
- SpringLayout layout = new SpringLayout();
- frame.getContentPane().setLayout(layout);
- frame.getContentPane().add(label1);
- frame.getContentPane().add(textField1);
- frame.getContentPane().add(label2);
- frame.getContentPane().add(textField2);
- // Adjust constraints for the label1 so it's at (10,10).
- layout.putConstraint(SpringLayout.WEST, label1, 10, SpringLayout.WEST, frame.getContentPane());
- layout.putConstraint(SpringLayout.NORTH, label1, 10, SpringLayout.NORTH, frame.getContentPane());
- // Adjust constraints for the text field so it's at
- // (<label1's right edge> + 10, 10).
- layout.putConstraint(SpringLayout.WEST, textField1, 10, SpringLayout.EAST, label1);
- layout.putConstraint(SpringLayout.NORTH, textField1, 10, SpringLayout.NORTH, frame.getContentPane());
- // Adjust constraints for the label2 so it's at (10,30).
- layout.putConstraint(SpringLayout.WEST, label2, 10, SpringLayout.WEST,frame.getContentPane());
- layout.putConstraint(SpringLayout.NORTH, label2, 30, SpringLayout.NORTH, frame.getContentPane());
- // Adjust constraints for the text field so it's at
- // (<label2's right edge> + 10, 30).
- layout.putConstraint(SpringLayout.WEST, textField2, 10, SpringLayout.EAST, label2);
- layout.putConstraint(SpringLayout.NORTH, textField2, 30, SpringLayout.NORTH, frame.getContentPane());
- // Display the window.
- frame.setSize(400,300);
- frame.setVisible(true);
- //close operation
- }
- }
Add new comment
- 105 views