JToolBar Component in Java
Submitted by donbermoy on Tuesday, November 25, 2014 - 15:44.
This is a tutorial in which we will going to create a program that will have a JToolBar in Java. A JToolBar provides a bar on the top of the program where it contains a group of components such as buttons, icons, labels, textfields, etc., that provide easy access to functionality of the other components.
So, now let's start this tutorial!
1. Open JCreator or NetBeans and make a java program with a file name of jToolBarComponent.java.
2. Import the javax.swing.* package to access the JButton, JFrame, JScrollPane, JTextArea, JList, JLabel, and JToolBar class.
3. Initialize your variable in your Main, variable frame for JFrame, variable textArea for JTextArea, variable pane for JScrollPane, and variable toolbar for JToolBar component.
4. To add components on the toolbar, we will used the add method.
To separate another components on the toolbar so that i cannot be connected to other components, we will use the addSeparator method.
We can also instantiate a component into the add method of the toolbar. We will instantiate a label and a list inside the toolbar. Have this code below:
Output:
Here's the full code of this tutorial:
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below.
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
- import javax.swing.*; // used to access the JButton, JFrame, JScrollPane, JTextArea, JList, JLabel, and JToolBar class
- toolbar.add(button);
- toolbar.addSeparator();
5. Now, add the toolbar and panel variable to the frame using the getContentPane().add method with the default BorderLayout of North and Center position, respectively. Lastly, set the size, visibility, and the close operation of the frame. Have this code below:
- frame.getContentPane().add(toolbar, "North");
- frame.getContentPane().add(pane, "Center");
- frame.setSize(350, 150);
- frame.setVisible(true);
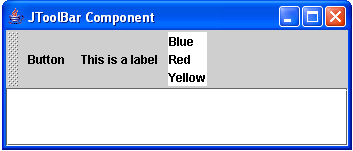
- import javax.swing.*; // used to access the JButton, JFrame, JScrollPane, JTextArea, JList, JLabel, and JToolBar class
- public class jToolBarComponent{
- toolbar.add(button);
- toolbar.addSeparator();
- toolbar.addSeparator();
- frame.getContentPane().add(toolbar, "North");
- frame.getContentPane().add(pane, "Center");
- frame.setSize(350, 150);
- frame.setVisible(true);
- }
- }